How to Read a CSV With Its Header in Python
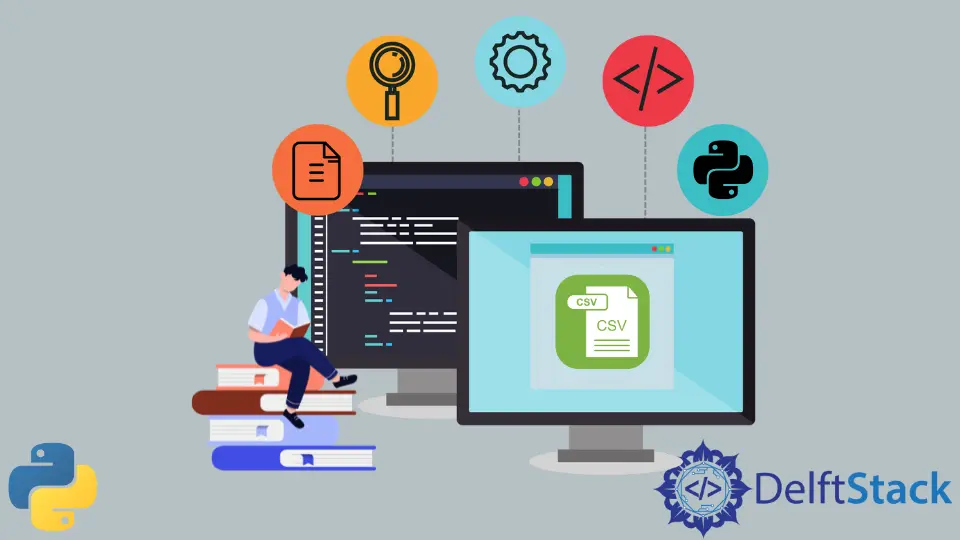
Python is a definite and easy-to-understand general-purpose programming language.
Python is one of the top five programming languages globally because it has a straightforward syntax and a lot of utilities for multiple domains such as machine learning, data science, game development, web development, app development, etc. Thanks to the APIs working behind the scenes.
Python makes file handling a seamless task. We can easily create files, read files, append data, or overwrite data in existing files using Python since it can handle a lot of file formats such as txt
, csv
, xlsx
, pdf
, etc.
Moreover, it has a lot of open-source packages that make file handling efficient and simpler.
We will learn how to read a CSV file and its header using Python in this article. So that we are on the same note, we will use a sample file for illustration purposes in the code snippets. You can download this CSV file from here. The examples ahead will consider the first row of the CSV file as its header.
Read a CSV With Its Header in Python
Python has a csv
package that we can use to read CSV files. This package is present by default in the official Python installation.
The csv
package has a reader()
method that we can use to read CSV files. It returns an iterable object that we can traverse to print the contents of the CSV file being read.
from csv import reader
file_name = "email.csv"
with open(file_name, "r") as csv_file:
csv_reader = reader(csv_file)
for row in csv_reader:
print(row)
Output:
['Login email', 'Identifier', 'First name', 'Last name']
['laura@example.com', '2070', 'Laura', 'Grey']
['craig@example.com', '4081', 'Craig', 'Johnson']
['mary@example.com', '9346', 'Mary', 'Jenkins']
['jamie@example.com', '5079', 'Jamie', 'Smith']
The time complexity of the above solution is O(n)
.
As we can see, the output shows that the first row is the header and the other rows have the values. We can further beautify the output by storing the header in a separate variable and printing everything in a suitable-looking format.
from csv import reader
file_name = "email.csv"
with open(file_name, "r") as csv_file:
csv_reader = reader(csv_file)
header = next(csv_reader)
print("Header:")
print(", ".join(header))
print("Values:")
for row in csv_reader:
print(", ".join(row))
Output:
Header:
Login email, Identifier, First name, Last name
Values:
laura@example.com, 2070, Laura, Grey
craig@example.com, 4081, Craig, Johnson
mary@example.com, 9346, Mary, Jenkins
jamie@example.com, 5079, Jamie, Smith
The time complexity of the above solution is O(n)
.
In the code above, csv_reader
is iterable. Using the next()
method, we first fetched the header from csv_reader
and then iterated over the values using a for
loop.
As the name suggests, CSV files have comma-separated values.
Sometimes, values inside CSV files are not comma-separated; they could be using a semicolon (;
), a colon (:
), etc., as its separator. In such cases, one can use the delimiter
argument of the reader()
method from the csv
package.
The delimiter
argument accepts a string or a character that should be considered as the separator. For example, if a CSV file has ;
as its separator, the code for reading such a CSV file would be as follows.
from csv import reader
file_name = "email.csv"
with open(file_name, "r") as csv_file:
csv_reader = reader(csv_file, delimiter=";") # Important
header = next(csv_reader)
print("Header:")
print(", ".join(header))
print("Values:")
for row in csv_reader:
print(", ".join(row))
Output:
Header:
Login email, Identifier, First name, Last name
Values:
laura@example.com, 2070, Laura, Grey
craig@example.com, 4081, Craig, Johnson
mary@example.com, 9346, Mary, Jenkins
jamie@example.com, 5079, Jamie, Smith
Related Article - Python CSV
- How to Import Multiple CSV Files Into Pandas and Concatenate Into One DataFrame
- How to Split CSV Into Multiple Files in Python
- How to Compare Two CSV Files and Print Differences Using Python
- How to Convert XLSX to CSV File in Python
- How to Write List to CSV Columns in Python
- How to Write to CSV Line by Line in Python