How to Print Variable in Python
-
Use the
print
Statement to Print a Variable in Python -
Use a Comma (
,
) to Separate the Variables to Print a Variable in Python -
Use the String Formatting With the Help of
%
to Print a Variable in Python -
Use the String Formatting With the Help of
{}
to Print a Variable in Python -
Use the
+
Operator in theprint
Statement to Print a Variable in Python -
Use the
f-string
in theprint
Statement to Print a Variable in Python -
Use the
format
Method in theprint
Statement to Print a Variable in Python - Conclusion
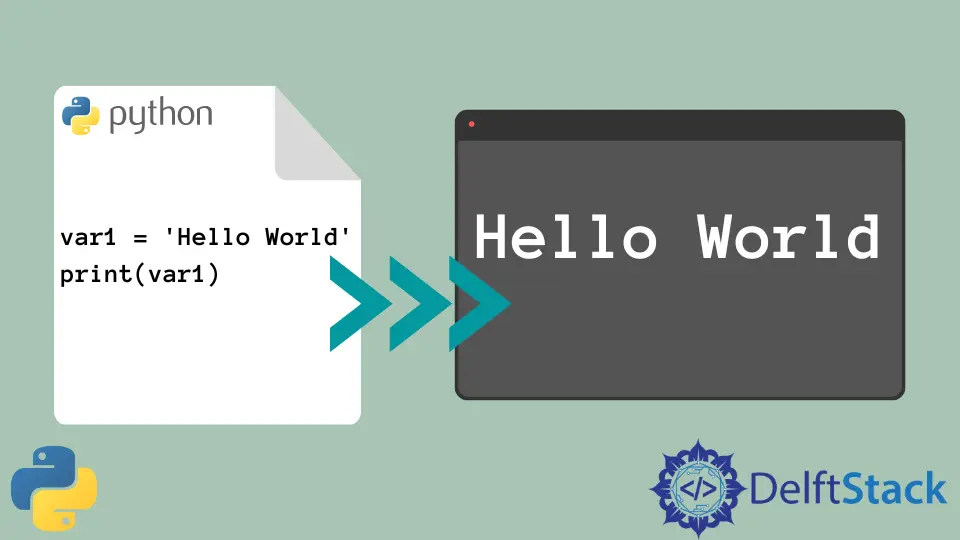
The print
statement in Python can be used in many different ways. We can choose the way we want to use the statement according to our convenience.
We’ll discuss in this tutorial the different methods to print a variable in Python.
Use the print
Statement to Print a Variable in Python
In Python, the print
statement is used to display the value of a variable or an expression on the console. It is a basic command that allows you to output information for debugging, testing, or user interaction.
The print
statement is commonly used to show the content of variables and is a fundamental tool for displaying output in Python programs.
The print
statement syntax can vary, depending on the Python’s version.
In Python 2, the syntax for the print
statement is pretty simple. All we have to do is use the print
keyword.
Example Code:
# Python 2 code
print "Hello to the World"
Output:
Hello to the World
In Python 3, to run the print
statement without any errors, there needs to be a parenthesis followed by the print
keyword.
Example Code:
# Python 3 code
print("Hello to the World")
Output:
Hello to the World
The print
statement is used to print variables, strings, and the other data types provided in Python. We can print different data types in Python’s single statement by separating the data types or variables with the ,
symbol.
We will show in this article the different methods to print a variable in Python.
Use a Comma (,
) to Separate the Variables to Print a Variable in Python
In Python, you can use a comma (,
) to separate variables within the print
statement, allowing you to display multiple variables or values in a single line. This syntax enables you to concatenate different pieces of information in the output without explicitly converting them to strings.
Using commas in the print
statement is a concise way to format and display multiple values together.
Example code:
var1 = 123
var2 = "World"
print("Hello to the", var2, var1)
Output:
Hello to the World 123
In the code, we defined two variables, var1
with the numeric value 123
and var2
with the string value World
. The print
statement is used to display a message, "Hello to the"
, followed by the values of var2
and var1
.
The output will be Hello to the World 123
. The comma-separated values in the print
statement automatically insert spaces between the words in the output.
Use the String Formatting With the Help of %
to Print a Variable in Python
In addition to just printing the statements, string formatting can also be used. This gives the user more options to customize the string-like setting and adjust the padding, alignment, set precision, and even the width.
One of the two ways to implement string formatting is using the %
sign. The %
sign, followed by a letter, works as a placeholder for the variable.
For example, %d
can be used as a placeholder when we need to substitute numerical values.
Example code:
var1 = 123
var2 = "World"
print("Hello to the %s %d " % (var2, var1))
Output:
Hello to the World 123
In this code, we defined two variables, var1
and var2
. The print
statement uses the %
formatting approach to embed the values of var2
and var1
into a string.
Specifically, the placeholders %s
and %d
indicate that a string and a decimal (integer) value should be inserted, respectively. The output will be Hello to the World 123
.
This method allows us to create a formatted string with a more structured approach.
Use the String Formatting With the Help of {}
to Print a Variable in Python
In Python, you can use string formatting with the help of curly braces {}
to embed variables within a string when using the print
statement.
This allows you to create more structured and readable output by inserting variable values into specific positions within the string. The variables are then substituted into the string at runtime.
String formatting is a versatile and effective way to customize the appearance of output in Python.
Example code:
var1 = 123
var2 = "World"
print("Hello to the {} {}".format(var2, var1))
Output:
Hello to the World 123
In this code, we defined two variables, var1
and var2
. The print
statement utilizes the format
method to construct a string with placeholders.
The curly braces {}
act as placeholders for the values of var2
and var1
. The format
method replaces these placeholders with the actual values of var2
and var1
.
The resulting output is Hello to the World 123
. The format()
function is added to Python 2.6 and works in all other versions after that.
Use the +
Operator in the print
Statement to Print a Variable in Python
The +
operator can also be used to separate the variables while using the print
statement.
We can use the +
operator within the print
statement to concatenate variables with strings and display them together. This approach involves combining the variable and string values directly using the +
operator, providing a simple way to include variable content in the output.
However, it’s worth noting that this method may require explicit type conversion if the variable is not already a string.
Example code:
var1 = 123
var2 = "World"
print("Hello to the " + var2 + " " + str(var1))
Output:
Hello to the World 123
In this code, we defined two variables, var1
and var2
. The print
statement uses the +
operator for string concatenation to construct a message.
Next, we are going to print the phrase "Hello to the"
, followed by the value of var2
and a space, and then the string representation of var1
. The output will be Hello to the World 123
.
Use the f-string
in the print
Statement to Print a Variable in Python
The f-string
in Python is another method of achieving string formatting and has the edge over the other two methods as it is comparatively faster than the other two mechanisms. An f-string
, short for “formatted string literal”, is a feature introduced in Python 3.6 that provides a short and readable way to embed expressions and variables into strings.
The f-strings
are created by prefixing a string literal with the letter 'f'
or 'F'
. Inside an f-string
, expressions enclosed in curly braces {}
are evaluated at runtime, and their results are inserted into the string.
Example code:
var1 = 123
var2 = "World"
print(f"Hello to the {var2} {var1}")
Output:
Hello to the World 123
In this code, we defined two variables, var1
and var2
. The print
statement utilizes an f-string
, denoted by the f
prefix before the string, and this allows us to directly embed the values of var2
and var1
within the string using curly braces {}
.
When executed, the output will be Hello to the World 123
. The f-strings
provide a convenient and expressive way to format strings in Python.
Use the format
Method in the print
Statement to Print a Variable in Python
We can use the format
method in the print
statement to format strings in Python. This method is used to replace placeholders in a string with values of variables.
This approach is especially useful when you want to create dynamic strings with variable values. The format
method allows for more complex formatting, including specifying the order of variables, aligning text, and formatting numbers.
Basic Syntax:
"string {} {}".format(variable1, variable2)
In the syntax, the .format()
method is applied to a string containing placeholders represented by curly braces("string {} {}".format()
). The variables variable1
and variable2
are specified as arguments within the parentheses of the .format()
method, and these variables replace the corresponding placeholders (the curly braces) in the string, allowing for dynamic content insertion.
Let’s have an example for better understanding.
Example code:
var1 = 123
var2 = "World"
print("Hello to the {} {}".format(var2, var1))
Output:
Hello to the World 123
In this code, we defined two variables, var1
and var2
. The print
statement employs the format
method to create a formatted string.
Next, we use curly braces {}
as placeholders within the string and then apply the format
method to replace these placeholders with the values of var2
and var1
. The output will be Hello to the World 123
.
Conclusion
In Python, the print
statement offers various methods for displaying variables. We explored syntax differences between Python 2 and 3, then delved into techniques like comma separation, %
formatting, {}
placeholders, and +
operator concatenation.
The f-strings
were highlighted for conciseness and the format
method for its flexibility. Each method serves different needs, providing a toolkit for effective variable output in Python.
Understanding these options enhances our ability to communicate information in a readable and expressive manner within Python programs.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn