How to Print Tab in Python
-
Print Python Tab in File Using the
\t
in theprint()
Function - Print Python Tab in the List
- Print Python Tab in the Datapoints
-
Print Python Tab Using the
tab
Symbol Directly in theprint
Statement
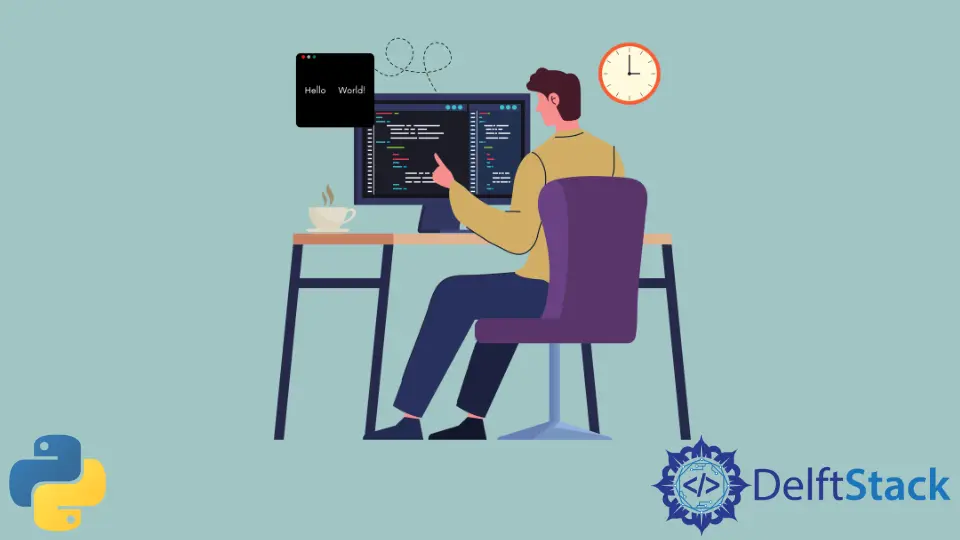
The '\'
backslash in Python strings is a special character, sometimes called the escape
character. It is used to represent whitespace characters as '\t'
represents a tab.
This article will discuss some methods to print the Python tab.
Print Python Tab in File Using the \t
in the print()
Function
We can use \t
in the print()
function to print the tab correctly in Python.
The complete example code is given below.
print("Python\tprogramming")
Output:
Python Programming
Print Python Tab in the List
This method will insert tabs between different elements of the list.
The complete example code is given below:
Lst = ["Python", "Java", "C++"]
print(str(Lst[0]) + "\t" + str(Lst[1]) + "\t" + str(Lst[2]))
Output:
python Java C++
str
will convert the list elements into the string with all values separated by Python tabs.
Print Python Tab in the Datapoints
This method will print tabs in the given statement or some data points.
The complete example code is given below:
Data_Points = "2\t6\t10"
print(Data_Points)
Output:
2 6 10
Print Python Tab Using the tab
Symbol Directly in the print
Statement
In this method, we will use the escape sequences in the string literals to print tab
. The escape sequences could be below types.
Escape Sequence | Description |
---|---|
\N{name} |
name is the Character name in the Unicode database |
\uxxxx |
16-bit Unicode |
\Uxxxxxxxx |
32-bit Unicode |
\xhh |
8-bit Unicode |
The name
of the table in the Unicode database is TAB
or tab
, or TaB
because it is not case insensitive.
The other names representing the tab in the Unicode database are HT
, CHARACTER TABULATION
, and HORIZONTAL TABULATION
.
The tab’s Unicode value is 09
for \x
, 0009
for \u
, and 00000009
for \U
.
The complete example code is given below:
print("python\N{TAB}programming")
print("python\N{tab}programming")
print("python\N{TaB}programming")
print("python\N{HT}programming")
print("python\N{CHARACTER TABULATION}programming")
print("python\N{HORIZONTAL TABULATION}programming")
print("python\x09programming")
print("python\u0009programming")
print("python\U00000009programming")
Output:
python programming
python programming
python programming
python programming
python programming
python programming
python programming
python programming
python programming