How to Print on the Same Line in Python
-
Multiple Prints on the Same Line in Python Using the
print()
Function -
Multiple Prints on the Same Line in Python Using the
stdout.write()
Method of thesys
Module in Python
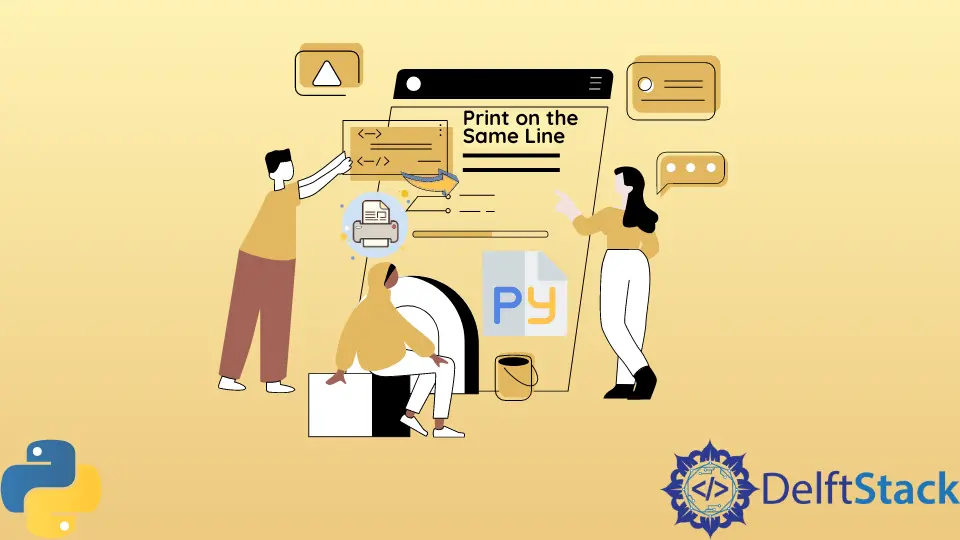
This tutorial will explain various methods to print multiple things on the same line in Python. Usually, the print()
method prints the content in the new line each time. We can use the following methods to print multiple things on the same line in Python.
Multiple Prints on the Same Line in Python Using the print()
Function
The print
method takes a string or any valid object as input, converts it into a string, and print it on the screen. To print multiple things on the same line using the print
function in Python, we will have to follow different ways depending on the Python version.
Python 2.x
In Python 2.x, we can put ,
at the end of the print
method to print multiple times on the same line. The following example code demonstrates how to the print
function for this.
print "hello...",
print "how are you?"
Output:
hello...how are you?
Python 3.x
And in Python 3.x, we will have to change the value of the end
parameter of the print()
method, as it is set to the \n
by default. The example code below demonstrates how we can use the print()
method with the end
parameter set as ""
to print multiple times on the same line.
print("hello...", end=""),
print("how are you?")
Output:
hello...how are you?
Multiple Prints on the Same Line in Python Using the stdout.write()
Method of the sys
Module in Python
The stdout.write()
method of the sys
module prints the output on the screen. As the stdout.write()
does not add the new line at the end of the string by default, it can be used to print multiple times on the same line.
Unlike the print()
method, this method works on all the Python versions, but we will need to import the sys
module first to use the stdout.write()
method. The example code below shows how to use the stdout.write()
method to print multiple strings on the same line in Python.
import sys
sys.stdout.write("hello...")
sys.stdout.write("how are you?")
Output:
hello...how are you?