Python-PPTX Library
-
What Is the
python-pptx
Library in Python -
How to Install the
python-pptx
Library - Create and Edit a PowerPoint File in Python
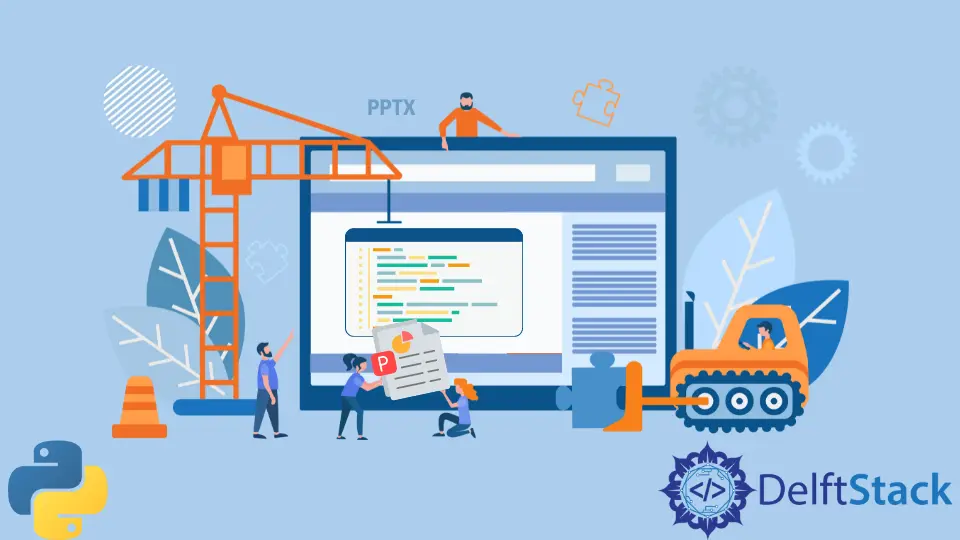
This tutorial will discuss the python-pptx
library and implement it in Python.
What Is the python-pptx
Library in Python
PowerPoint is widely recognized as the most popular software for creating and editing presentations. Python provides a library named python-pptx
utilized to create or edit PowerPoint files.
These files have a pptx
extension. The python-pptx
library can function only on newer versions released after Microsoft Office 2003.
Inserting paragraphs, shapes, slides, and plenty more to a PowerPoint presentation can be done through this library.
How to Install the python-pptx
Library
The python pptx library can be installed by simply utilizing the pip
command. The following command must be written on the command prompt to install the python-pptx
library.
pip install python-pptx
We should note that this package works on Python 2.6 and releases after that.
The first half of the article explains what the python-pptx
library was. The other half of the article will demonstrate the various functions of the python-pptx
library to create and edit a PowerPoint presentation.
Create and Edit a PowerPoint File in Python
Create a New PowerPoint File and Add Title/Subtitles
Firstly, we will import the pptx
library to the Python code to ensure no errors while utilizing the pptx
library functions. Then, we will create a presentation object and apply the necessary functions to it.
The following code shows how to create a presentation object and add a title and subtitles.
from pptx import Presentation
X = Presentation() # Presentation object created
slide1_layout = X.slide_layouts[0] # Slide layout process
slide = X.slides.add_slide(slide1_layout)
# Then, we create title
slide.shapes.title.text = " PPT TITLE (PYTHON) "
X.save("delft.pptx") # File saved
print("done")
The above code provides the following output.
Convert .pptx
File to a .txt
File
Another essential step to understanding Python’s pptx
library is converting a presentation with the (.pptx)
extension to a text file with the (.txt)
extension.
The following code converts a file with the (.pptx)
to the (.txt)
extension.
from pptx import Presentation
X = Presentation("abc.pptx") # Presentation object created
# Then file is opened in write mode
ftw_data = open("fte_ppt.txt", "w")
# write text from powerpoint
# file into .txt file
for slide in X.slides:
for shape in slide.shapes:
if not shape.has_text_frame:
continue
for paragraph in shape.text_frame.paragraphs:
for run in paragraph.runs:
ftw_data.write(run.text)
ftw_data.close() # The file is closed
print("Done")
Insert an Image Into a PowerPoint Presentation
Another one of the essentials of editing a PowerPoint presentation through Python is to learn how to add an image to a PowerPoint presentation.
The following code inserts an image into a PowerPoint presentation.
from pptx import Presentation
from pptx.util import Inches
img_path = "vk.png" # specify image path
X = Presentation() # presentation object created
bs_layout = X.slide_layouts[6] # select blank slide layout
slide = X.slides.add_slide(bs_layout)
left = top = Inches(1) # add margins
pic = slide.shapes.add_picture(img_path, left, top) # add images
left = Inches(1)
height = Inches(1)
pic = slide.shapes.add_picture(img_path, left, top, height=height)
X.save("test_4.pptx") # file is saved
print("Done")
The above code provides the following output.
Here, we have covered some essentials of creating and editing PowerPoint presentations in Python.
Additionally, we can utilize several functions in the pptx
library to customize more things like adding charts, tables, shapes, etc.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn