How to Run Another Python Script in Python
-
Use the
import
Statement to Run a Python Script in Another Python Script -
Use the
execfile()
Method to Run a Python Script in Another Python Script -
Use the
subprocess
Module to Run a Python Script in Another Python Script -
Use the
os.system()
Function to Run a Python Script in Another Python Script -
Use the
importlib
Module to Run a Python Script in Another Python Script - Conclusion
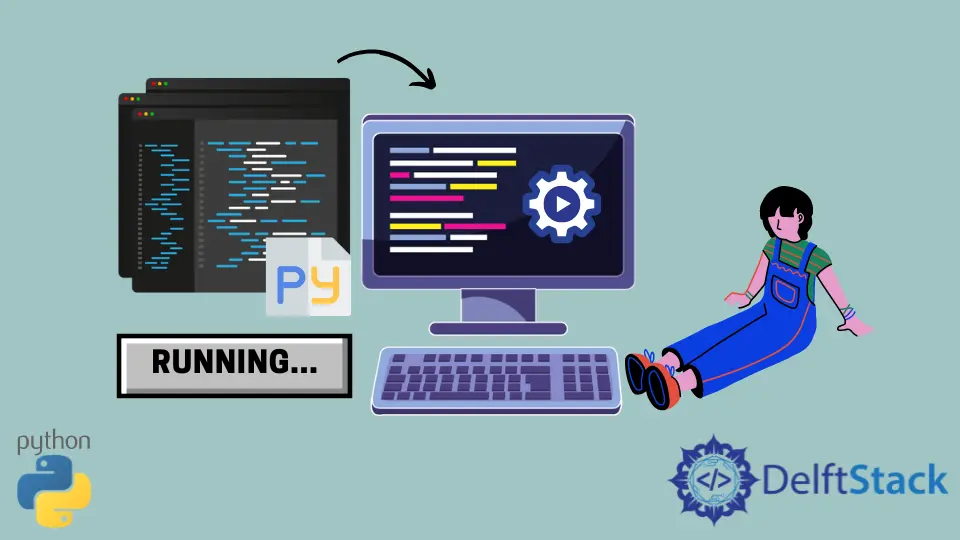
A basic text file containing Python code that is intended to be directly executed by the client is typically called a script, formally known as a top-level program file.
Scripts are meant to be directly executed in Python. Learning to run scripts and code is a fundamental skill to learn in the world of Python programming.
Python script usually has the extension '.py'
. If the script is run on a Windows machine, it might have an extension, .pyw
.
This tutorial will discuss different methods to run a Python script inside another Python script.
Use the import
Statement to Run a Python Script in Another Python Script
The import
statement is used to import several modules to the Python code. It is used to get access to a particular code from a module. This method uses the import
statement to import the script in the Python code and uses it as a module.
Modules can be defined as a file that contains Python definitions and statements.
The following code uses the import
statement to run a Python script in another Python script.
helper_script.py
:
def helper_function():
print("Hello from helper_function!")
if __name__ == "__main__":
# This will run if helper_script.py is executed directly
print("helper_script.py is being run directly")
helper_function()
main_script.py
:
import helper_script
# Using the function from helper_script.py
helper_script.helper_function()
if __name__ == "__main__":
print("main_script.py is being run directly")
In helper_script.py
, we have created the function helper_function()
along with some top-level code within the if __name__ == "__main__":
block. This specific block is designed to run only when helper_script.py
is executed directly, and it won’t run during an import.
When we work with main_script.py
, we import helper_script
. During this importation, Python runs the helper_script.py
file.
However, because it’s an import action, the if __name__ == "__main__":
block in helper_script.py
does not get executed.
In main_script.py
, we then call helper_function()
from helper_script
. This script also includes its own if __name__ == "__main__":
block, which is set to execute code specifically when main_script.py
is run directly.
Output:
Use the execfile()
Method to Run a Python Script in Another Python Script
The execfile()
function executes the desired file in the interpreter. This function only works in Python 2.
In Python 3, the execfile()
function was removed, but the same thing can be achieved in Python 3 using the exec()
method.
The following code uses the execfile()
function to run a Python script in another Python script.
executed_script.py
:
# Python 2 code
print("This script is being run.")
if __name__ == "__main__":
print("executed_script is run directly")
else:
print("executed_script is executed from another script")
main_script.py
:
# Python 2 code
filename = "executed_script.py"
execfile(filename, {"__name__": ""})
In executed_script.py
, we have defined a function some_function()
and included a top-level code check using __name__ == "__main__"
. This check enables the script to ascertain whether it is being run directly or executed by another script.
In main_script.py
, we apply execfile()
to execute executed_script.py
. When we invoke execfile()
, it runs executed_script.py
as though its contents were part of main_script.py
.
In terms of namespace manipulation, we pass {"__name__": ""}
as the second argument to execfile()
, creating a global namespace for execution. By setting the "__name__"
key in this dictionary, we simulate an environment where executed_script.py
is not the main program.
Since executed_script.py
is not the main program in execution, __name__ != "__main__"
within executed_script.py
and behaves like an imported module.
Output:
The same thing can be done in Python 3 by using:
main_script.py
:
# main_script.py
with open("executed_script.py", "r") as file:
exec(file.read(), {"__name__": ""})
In our process, we first open executed_script.py
for reading using with open('executed_script.py', 'r') as file:
. This approach ensures that executed_script.py
is appropriately closed after reading.
We then read and execute the script with exec(file.read(), {"__name__": ""})
. Here, file.read()
retrieves the entire content of executed_script.py
as a string, and exec()
executes this string as Python code.
In terms of namespace manipulation, we pass {"__name__": ""}
as the second argument to exec()
, creating a global namespace for execution. By setting the "__name__"
key in this dictionary, we simulate an environment where executed_script.py
is not the main program.
In Python, a script’s __name__
variable is set to "__main__"
when run directly. However, we manually adjust the namespace to mimic an imported module’s behavior.
Consequently, in executed_script.py
, our conditional check if __name__ == "__main__":
normally evaluates to True
when the script runs directly. But due to our namespace manipulation in main_script.py
, __name__
is not "__main__"
in the executed script’s context, making it behave as if it were imported.
Output:
Use the subprocess
Module to Run a Python Script in Another Python Script
The subprocess
module is capable of spawning new processes and can also return their outputs. This is a new module and is intended to replace several older modules like os.system
, which was previously used to run a Python script in another Python script.
The following code uses the subprocess
module to run a Python script in another Python script.
executed_script.py
:
# executed_script.py
def main():
print("This is executed_script.py running!")
if __name__ == "__main__":
main()
main_script.py
:
# main_script.py
import subprocess
# Command to run executed_script.py
command = ["python3", "executed_script.py"]
# Using subprocess to run the script
subprocess.run(command)
In executed_script.py
, we define a main()
function responsible for printing a message. Additionally, we include an if __name__ == "__main__":
check to determine if the script is the program’s entry point.
If this is the case, we call main()
.
Moving to main_script.py
, we start by importing the subprocess
module. We then define a command to run executed_script.py
using the Python interpreter.
To execute the script, we call subprocess.run(command)
, thereby running executed_script.py
.
Output:
Use the os.system()
Function to Run a Python Script in Another Python Script
Running a Python script from another Python script using the os.system
method is a straightforward approach. This method is a part of the os
module, which provides a way of using operating system dependent functionality.
The os.system
command takes a string as an argument and runs it as a command in the system’s command line interface (CLI).
executed_script.py
:
# executed_script.py
def main():
print("This is executed_script.py running!")
if __name__ == "__main__":
main()
main_script.py
:
import os
exit_status = os.system("python3 executed_script.py")
print(f"Exit Status: {exit_status}")
In executed_script.py
, we define a main()
function that outputs a message. We also use if __name__ == "__main__":
to guarantee that main()
only runs when the script serves as the program’s entry point.
Meanwhile, in main_script.py
, we import the os
module. Here, we set up the command to run executed_script.py
as a string.
By using os.system(command)
, we execute the script as a command within the system’s shell, allowing executed_script.py
to be run externally.
Output:
Use the importlib
Module to Run a Python Script in Another Python Script
The importlib
module is part of Python’s standard library and is used for importing modules programmatically.
Python’s flexibility in running scripts is one of its key strengths, and the importlib
module provides a dynamic way of importing and running scripts. Unlike the conventional import
statement, importlib
allows for the dynamic execution of Python scripts, even when their names are not known until runtime.
executed_script.py
:
# executed_script.py
def main():
print("executed_script.py is running as a module.")
if __name__ == "__main__":
print("executed_script.py is running directly.")
else:
main()
main_script.py
:
import importlib
# Dynamically importing executed_script.py
module_name = "executed_script"
executed_script = importlib.import_module(module_name)
In executed_script.py
, we incorporate a main()
function that features a print
statement. To distinguish between the script being executed directly and being imported as a module, we use if __name__ == "__main__":
.
In main_script.py
, our approach involves importing the importlib
module. We then employ importlib.import_module()
to dynamically import executed_script.py
, relying on the string module_name
to specify the module.
As a result of this importation, executed_script.py
executes the code within its else
block, which includes a call to the main()
function.
Output:
Conclusion
In this article, we explored various methods to execute a Python script from another Python script, each catering to different needs. The import
statement, ideal for integrating scripts as modules, emphasizes Python’s readability and simplicity.
importlib
offers flexibility for dynamic script importation, which is useful in complex scenarios. For executing scripts as standalone processes, subprocess
and os.system()
are practical choices.
subprocess
is robust and versatile, ideal for detailed process control, while os.system()
suits simpler, direct executions. Each method has its unique advantages, and the choice largely depends on the task’s specific requirements, such as process control needs, script complexity, and the desired level of script integration.
This guide aims to provide Python developers with the knowledge to choose the right tool for running scripts efficiently within various contexts.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn