How to Pass List to Function in Python
- Passing Lists as Function Arguments
- Preserving Data Integrity When Passing List to Python Function
- Passing Lists as Multiple Arguments
- Variable-Length Arguments and Utilizing Lists
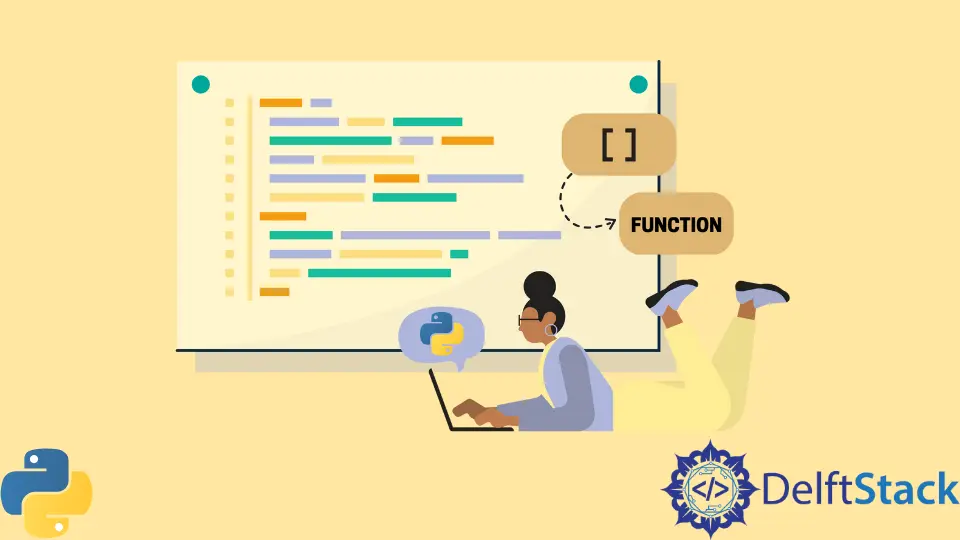
A list is one of the four fundamental data types that Python provides to store data. All these data types like lists, tuples, and dictionaries must sometimes be passed as an argument to a generic function.
This flexibility allows you to work with and manipulate lists within functions, making your code more modular and versatile. Let’s explore the basics of passing lists to functions with simple examples.
Passing Lists as Function Arguments
When you pass a list as an argument to a Python function, you’re essentially providing that function access to the list’s data. Any changes made to the list within the function will directly affect the original list outside the function. This behavior is because lists are mutable in Python, and when passed to a function, they are passed by reference.
Here’s a straightforward example to illustrate this concept:
def add_one_to_elements(my_list):
for i in range(len(my_list)):
my_list[i] += 1
In this example, the add_one_to_elements
function takes a list my_list
as an argument. It then iterates through the elements of the list and adds one to each element. When you call this function and pass a list to it, the original list will be modified.
my_numbers = [1, 2, 3, 4, 5]
add_one_to_elements(my_numbers)
print(my_numbers) # Output: [2, 3, 4, 5, 6]
As you can see, the original my_numbers
list has been modified by the function because lists are mutable and are passed by reference. This behavior can be useful, but it can also lead to unintended side effects if you’re not careful.
In the following sections, we’ll explore the implications of passing mutable lists to functions and discuss techniques to overcome potential challenges.
The Risk of Unintended Modifications
One significant implication of mutable lists in functions is the risk of unintended modifications to the original list. If you’re not careful or aware that a function modifies a list, it can lead to unexpected behavior in your code.
Here’s an example that demonstrates this risk:
def remove_first_element(my_list):
my_list.pop(0)
my_numbers = [1, 2, 3]
remove_first_element(my_numbers)
print(my_numbers) # Output: [2, 3]
In this scenario, we pass my_numbers
to the remove_first_element
function, which removes the first element from the list. If you didn’t anticipate this behavior, it could cause issues in your code.
Preserving Data Integrity When Passing List to Python Function
To maintain data integrity in your function calls, it’s essential to be aware of the mutable nature of lists and take steps to prevent unintended modifications. You can do this by:
1. Creating a Copy of the List
def modify_list_safely(my_list):
my_list_copy = my_list.copy()
my_list_copy.append(4)
return my_list_copy
original_list = [0, 1, 2]
new_list = modify_list_safely(original_list)
print(original_list) # Output: [0, 1, 2]
print(new_list) # Output: [0, 1, 2, 4]
Here, we make a copy of the original_list
within the modify_list_safely
function. Any changes made to my_list_copy
won’t affect the original list.
2. Using Immutable Data Types
If possible, consider using immutable data types like tuples. Tuples are similar to lists but cannot be modified after creation. By passing a tuple to a function, you ensure that its contents remain unchanged.
def modify_tuple(my_tuple):
my_list = list(my_tuple)
my_list.append(4)
return my_list
original_tuple = (0, 1, 2)
new_list = modify_tuple(original_tuple)
print(original_tuple) # Output: (0, 1, 2)
print(new_list) # Output: [0, 1, 2, 4]
3. Using *args to Convert Lists to Tuples
You can use the *args
syntax in the function definition to automatically convert a list to a tuple inside the function. This prevents modifications to the original list.
def process_data(*args):
# args is now a tuple, not a list
args_list = list(args)
args_list.append(4)
return args_list
original_list = [0, 1, 2]
new_list = process_data(*original_list)
print(original_list) # Output: [0, 1, 2]
print(new_list) # Output: [0, 1, 2, 4]
Understanding the implications of mutable lists and taking steps to preserve data integrity in function calls are essential aspects of writing robust and predictable Python code.
Passing Lists as Multiple Arguments
In Python, you can pass the elements of a list as multiple arguments to a function using the asterisk (*
) operator. This technique is known as unpacking, and it allows you to pass each element of the list as an individual argument to the function. Let’s dive into the concept of passing lists as multiple arguments and provide examples to illustrate this functionality.
Unpacking Lists with the Asterisk Operator
The asterisk (*
) operator, when applied to a list or any iterable, allows you to unpack its elements. This means that each element in the list will be treated as a separate argument when calling a function.
Here’s a simple example:
def add(a, b, c):
return a + b + c
my_list = [1, 2, 3]
result = add(*my_list)
print(result) # Output: 6
In this example, the elements of my_list
are unpacked and passed as arguments to the add
function, allowing us to perform the addition operation as if we had called add(1, 2, 3)
.
Benefits of Unpacking Lists
Unpacking lists can be particularly useful when working with functions that require a specific number of arguments. It allows you to use the contents of a list as function arguments, making your code more flexible and concise.
Unpacking also simplifies the process of passing dynamic lists with varying lengths to functions, as you can adapt the number of arguments based on the list’s size.
In summary, handling lists inside functions involves understanding how lists maintain their mutable nature when passed as arguments and leveraging common operations to manipulate their contents. Additionally, passing lists as multiple arguments using the asterisk operator enhances the versatility and readability of your code.
Variable-Length Arguments and Utilizing Lists
Python provides a powerful feature for defining functions that accept a variable number of arguments. This feature is known as variable-length arguments, and it allows you to pass an arbitrary number of arguments to a function. Lists can be a valuable tool when working with variable-length arguments, offering flexibility and convenience in function design.
Understanding Variable-Length Arguments
In Python, you can use two special symbols to denote variable-length arguments:
*args
: This notation allows you to pass a non-keyword variable-length argument list to a function. It collects any number of positional arguments into a tuple.**kwargs
: This notation allows you to pass a keyword variable-length argument list to a function. It collects any number of keyword arguments into a dictionary.
For this discussion, we’ll focus on *args
and how lists can be utilized in conjunction with it.
Leveraging Lists with *args
When working with variable-length arguments using *args
, lists can serve as a convenient means to pass multiple values to a function. Lists can be unpacked and passed as separate arguments within the tuple created by *args
. This enables you to work with collections of data efficiently and simplifies your function calls.
Here’s an illustrative example:
def calculate_total(*args):
total = sum(args)
return total
numbers = [1, 2, 3, 4, 5]
result = calculate_total(*numbers)
print(result) # Output: 15
In this example, the calculate_total
function accepts a variable number of arguments using *args
. By unpacking the numbers
list with *numbers
, we can pass its elements as separate arguments to the function. This allows us to calculate the total sum of the numbers effortlessly.
Utilizing lists with *args
enhances the versatility of your functions, as it enables you to work with various sets of data without having to specify the exact number of arguments in advance. This flexibility can be particularly valuable in situations where you need to process different-sized datasets or handle dynamic input.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python