How to Convert a List to Lowercase in Python
-
Method 1: Using a
for
Loop - Method 2: Using List Comprehensions
- Method 3: Using the Map Function
- Conclusion
- FAQ
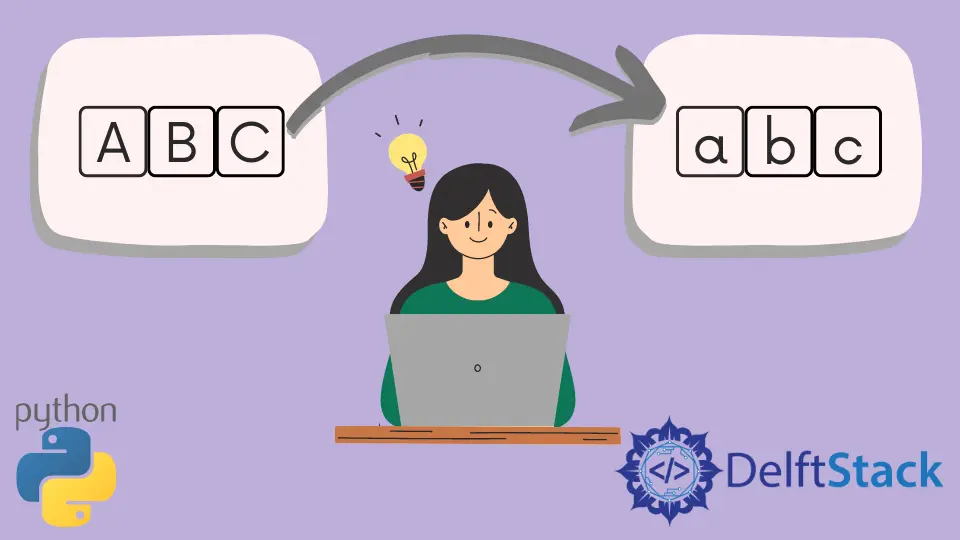
In the world of programming, data manipulation is a fundamental skill. One common task that many Python developers encounter is converting a list of strings to lowercase. Whether you’re preparing data for analysis, cleaning user input, or simply standardizing text, transforming strings to lowercase can be essential.
In this tutorial, we will explore several effective methods to achieve this in Python. We’ll cover simple loops, list comprehensions, and the built-in map
function. Each method has its own advantages, and by the end of this article, you will be well-equipped to handle string manipulation tasks with ease. Let’s dive into the various techniques for converting lists to lowercase in Python.
Method 1: Using a for
Loop
One straightforward way to convert a list of strings to lowercase is by using a for loop. This method involves iterating over each element in the list, applying the lower()
method, and appending the results to a new list. This approach is easy to understand and read, making it an excellent choice for beginners.
Here’s how you can implement it:
original_list = ['Hello', 'WORLD', 'Python', 'Programming']
lowercase_list = []
for item in original_list:
lowercase_list.append(item.lower())
print(lowercase_list)
Output:
['hello', 'world', 'python', 'programming']
In this example, we start with a list called original_list
containing mixed-case strings. We create an empty list named lowercase_list
to store the results. By iterating through each string in the original list, we apply the lower()
method, which converts the string to lowercase. Each converted string is then appended to lowercase_list
. Finally, we print the new list, which contains all the elements in lowercase. This method is straightforward and effective, especially for those who prefer explicit loops.
Method 2: Using List Comprehensions
If you’re looking for a more concise and Pythonic way to convert a list to lowercase, list comprehensions are the way to go. This method allows you to create a new list in a single line of code, making it not only efficient but also elegant.
Here’s how it works:
original_list = ['Hello', 'WORLD', 'Python', 'Programming']
lowercase_list = [item.lower() for item in original_list]
print(lowercase_list)
Output:
['hello', 'world', 'python', 'programming']
In this snippet, we utilize a list comprehension to iterate over original_list
. For each item
, we call the lower()
method and directly construct a new list, lowercase_list
, with the lowercase versions of the strings. This method is not only shorter but also improves readability for those familiar with Python’s syntax. List comprehensions are often favored for their efficiency and clarity, making them a popular choice among Python developers.
Method 3: Using the Map Function
Another effective method to convert a list to lowercase is by using the built-in map()
function. This function applies a specified function to each item in the iterable, which in this case is our list of strings. The map()
function is particularly useful when you want to apply a transformation across all elements without explicitly writing a loop.
Here’s how to use it:
original_list = ['Hello', 'WORLD', 'Python', 'Programming']
lowercase_list = list(map(str.lower, original_list))
print(lowercase_list)
Output:
['hello', 'world', 'python', 'programming']
In this example, we use map()
to apply the str.lower
method to each element of original_list
. The map()
function returns a map object, which we convert to a list using the list()
constructor. This method is efficient and can handle larger datasets with ease. It’s a great option for those looking to streamline their code and minimize the use of explicit loops. Additionally, using map()
can sometimes lead to better performance in larger applications.
Conclusion
Converting a list to lowercase in Python is a fundamental task that can be approached in various ways. Whether you prefer the clarity of a for loop, the elegance of list comprehensions, or the efficiency of the map()
function, Python offers flexible options to meet your needs. Each method has its strengths, so you can choose the one that best fits your coding style and project requirements. Armed with these techniques, you’ll be well-prepared to handle string manipulation tasks in Python with confidence.
FAQ
-
How do I convert a single string to lowercase in Python?
You can use thelower()
method directly on a string, like this:my_string.lower()
. -
Can I convert a list of mixed data types to lowercase?
If your list contains non-string items, you’ll need to filter or convert them to strings first before applying thelower()
method. -
Are there performance differences between these methods?
Generally, list comprehensions and themap()
function are faster than traditional for loops, especially with larger lists. -
What if my list contains empty strings?
Thelower()
method will simply return an empty string for any empty elements, so your list will still be processed correctly.
- Is there a way to convert a list to uppercase?
Yes, you can use the same methods but replacelower()
withupper()
to convert strings to uppercase.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python