How to Loop Through a List in Python
- Using a Basic For Loop
-
Using a
while
Loop - Using List Comprehensions
- Using the Enumerate Function
- Conclusion
- FAQ
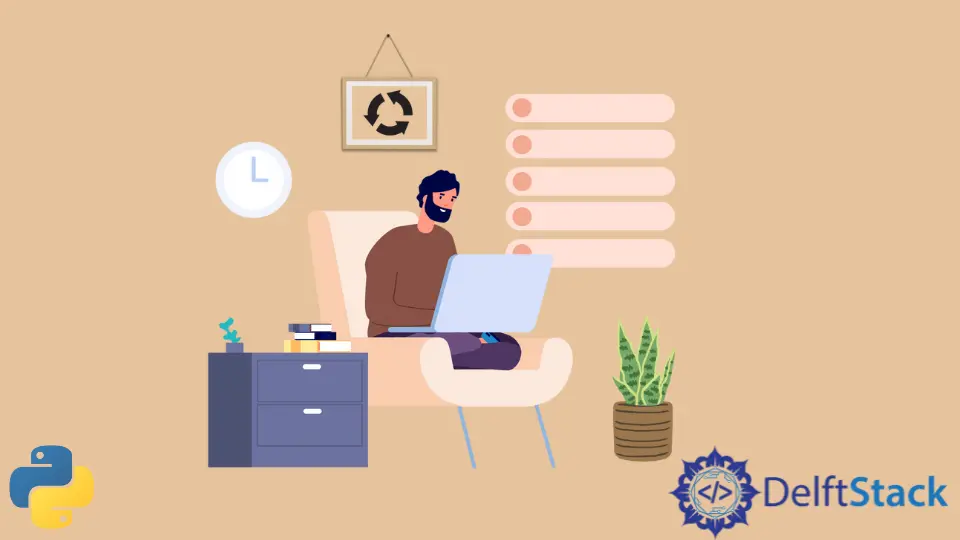
When working with lists in Python, looping through them is a fundamental skill that every programmer should master. Whether you’re processing data, manipulating elements, or simply iterating for display, understanding how to effectively loop through lists can significantly enhance your coding efficiency.
In this tutorial, we’ll explore various methods to loop through a list in Python, including the basic for loop, while
loop, and some advanced techniques like list comprehensions. By the end of this article, you’ll have a solid grasp of how to navigate through lists, enabling you to write cleaner and more efficient code.
Using a Basic For Loop
The most straightforward way to loop through a list in Python is by using the for loop. This method allows you to iterate through each element in the list, performing operations as needed. Here’s how you can do it:
fruits = ['apple', 'banana', 'cherry', 'date']
for fruit in fruits:
print(fruit)
Output:
apple
banana
cherry
date
In this example, we define a list called fruits
containing several fruit names. The for loop iterates over each element in the list, assigning it to the variable fruit
during each iteration. The print statement then outputs the current fruit to the console. This method is simple and effective, making it ideal for beginners. It allows you to access each element without needing to worry about the index, making your code cleaner and easier to read.
Using a while
Loop
Another approach to loop through a list is by using a while
loop. This method can be particularly useful when you need more control over the iteration process. Here’s an example:
fruits = ['apple', 'banana', 'cherry', 'date']
index = 0
while index < len(fruits):
print(fruits[index])
index += 1
Output:
apple
banana
cherry
date
In this case, we initialize an index variable to zero. The while
loop continues as long as the index is less than the length of the fruits list. Inside the loop, we access each element using its index and print it. After printing, we increment the index by one to move to the next element. While this method provides more flexibility, it requires careful management of the index variable to avoid errors, such as going out of bounds.
Using List Comprehensions
List comprehensions are a powerful feature in Python that allows for concise and efficient looping through lists. They provide a way to create new lists by applying an expression to each element in an existing list. Here’s how you can use a list comprehension:
fruits = ['apple', 'banana', 'cherry', 'date']
upper_fruits = [fruit.upper() for fruit in fruits]
print(upper_fruits)
Output:
['APPLE', 'BANANA', 'CHERRY', 'DATE']
In this example, we create a new list called upper_fruits
that contains the uppercase versions of each fruit in the original list. The list comprehension iterates through each fruit
in fruits
, applies the upper()
method, and collects the results in a new list. This method is not only more concise but also often more efficient than traditional loops, especially for larger lists. It allows you to express complex operations in a single line of code, making your programs cleaner and more readable.
Using the Enumerate Function
Sometimes, you may want to loop through a list while also keeping track of the index of each element. The enumerate()
function is perfect for this scenario, as it provides both the index and the value during iteration. Here’s an example:
fruits = ['apple', 'banana', 'cherry', 'date']
for index, fruit in enumerate(fruits):
print(f"Index {index}: {fruit}")
Output:
Index 0: apple
Index 1: banana
Index 2: cherry
Index 3: date
In this code snippet, the enumerate()
function returns both the index and the fruit as we loop through the list. This is particularly useful when you need to perform operations that depend on the position of the element within the list. By using enumerate()
, you avoid the need to manually manage an index variable, which can reduce the likelihood of errors and make your code cleaner.
Conclusion
Looping through lists in Python is an essential skill that every programmer should develop. Whether you opt for a basic for loop, a while
loop, list comprehensions, or the enumerate function, each method has its own advantages and use cases. By understanding these techniques, you can write more efficient and readable code, making your programming experience smoother and more enjoyable. As you continue to practice and explore these methods, you’ll find that they become second nature, allowing you to focus on solving problems rather than getting bogged down in syntax.
FAQ
-
What is a list in Python?
A list in Python is a collection of items that can be of different types, including integers, strings, and other objects. Lists are mutable, meaning you can change their content. -
Can you loop through a list without using a loop?
Yes, you can use functions likemap()
andfilter()
, which operate on lists without explicit loops, but they may not provide the same level of control. -
What is the difference between a for loop and a
while
loop?
A for loop iterates over a sequence (like a list), while awhile
loop continues until a specified condition is met. For loops are generally easier for iterating through lists. -
How can I loop through a list in reverse?
You can loop through a list in reverse by using thereversed()
function or by using slicing withlist[::-1]
. -
Are list comprehensions better than traditional loops?
List comprehensions can be more concise and often faster than traditional loops, but they may sacrifice readability for complex operations. It’s essential to use them judiciously.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python