How to Replace an Element in Python List
- Find and Replace the Python List Elements With the List Indexing Method
-
Find and Replace the Python List Elements With the
for
Loop Method - Find and Replace the Python List Elements With the List Comprehension Method
-
Find and Replace the Python List Elements With the
map
Method - Remarks
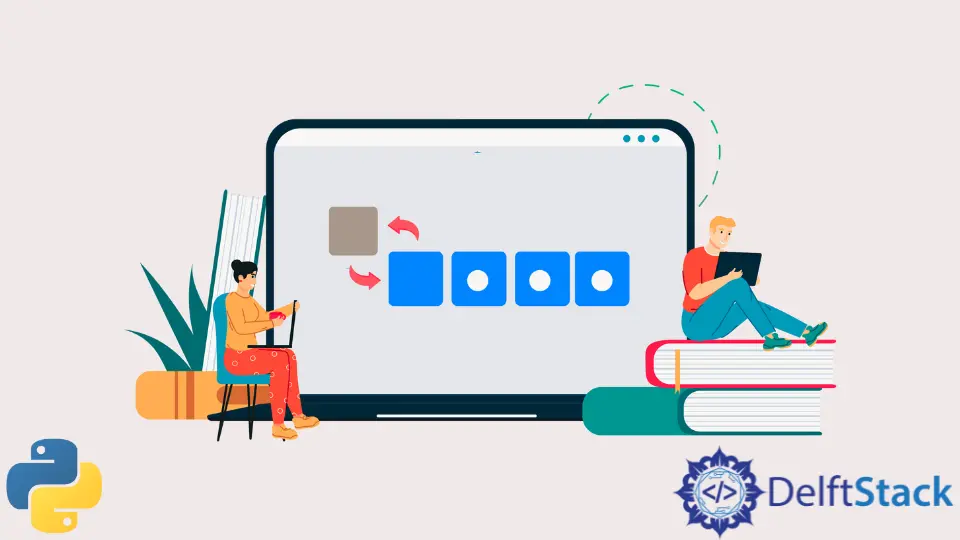
We could replace elements in a Python list in several ways. We can use Python list elements indexing, for
loop, map
function, and list comprehension methods.
This article will discuss the above methods to find and replace the Python list elements.
Find and Replace the Python List Elements With the List Indexing Method
Let’s take the below list as an example.
my_list = [5, 10, 7, 5, 6, 8, 5, 15]
We will change the element at the index 0
from 5 to 20.
The example code is as follows.
my_list = [5, 10, 7, 5, 6, 8, 5, 15]
my_list[0] = 20
print(my_list)
Output:
[20, 10, 7, 5, 6, 8, 5, 15]
Find and Replace the Python List Elements With the for
Loop Method
We use the enumerate()
function in this method. It returns a enumerate
object that also contains the counter together with the elements. When we combine the enumerate()
function with the for
loop, it iterates the enumerate
object and gets the index and element together.
The code is:
my_list = [5, 10, 7, 5, 6, 8, 5, 15]
for index, value in enumerate(my_list):
if value == 5:
my_list[index] = 9
print(my_list)
Output:
[9, 10, 7, 9, 6, 8, 9, 15]
Find and Replace the Python List Elements With the List Comprehension Method
In this method, we can generate a new list by applying pre-defined conditions on the old list.
The Syntax is:
my_list = [5, 10, 7, 5, 6, 8, 5, 15]
[9 if value == 5 else value for value in my_list]
print(my_list)
Output:
[9, 10, 7, 9, 6, 8, 9, 15]
Find and Replace the Python List Elements With the map
Method
This method changes the entries of the second list with the index of the first list items.
The code is:
list_1 = [5, 10, 7]
list_2 = [7, 10, 7, 5, 7, 5, 10]
ent = {k: i for i, k in enumerate(list_1)}
result = list(map(ent.get, list_2))
print("list2 after replacement is:", result)
Output:
list2 after replacement is: [2, 1, 2, 0, 2, 0, 1]
Remarks
- List indexing method is good when we replace one element in a list.
- List comprehension method is the right choice when we replace multiple elements in a list based on selective criteria.
- Loop methods are discouraged, as it takes more execution time and memory.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python