How to Get Length of the List in Python
- Using the Built-in len() Function
- Using a Loop to Count Elements
- Using List Comprehension with the sum() Function
- Conclusion
- FAQ
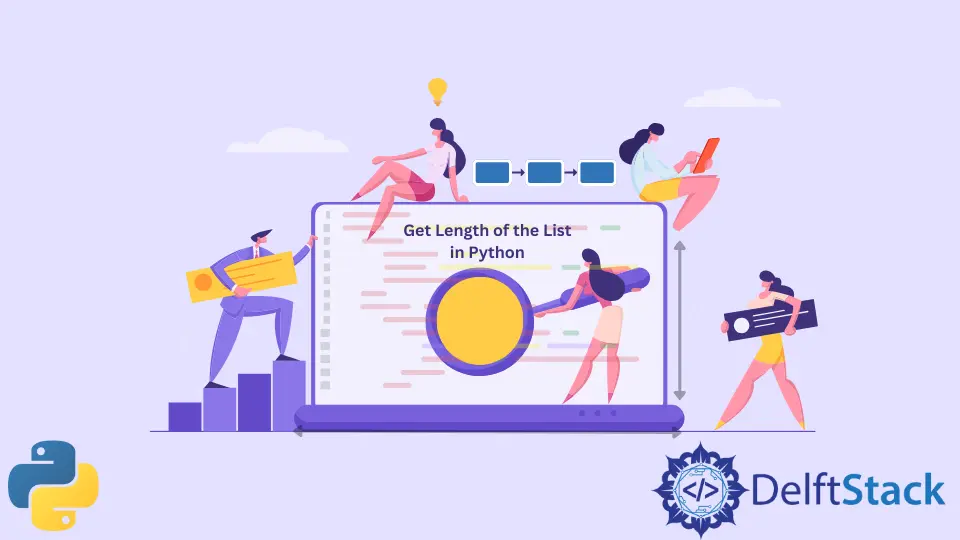
When working with lists in Python, knowing how to determine their length is essential. Whether you’re processing data, analyzing user inputs, or simply managing collections of items, understanding how to retrieve the length of a list can streamline your code and enhance functionality.
This tutorial will guide you through several effective methods to get the length of a list in Python, complete with clear examples and explanations. By the end of this guide, you’ll be equipped with the knowledge to handle lists efficiently and confidently in your Python projects.
Using the Built-in len() Function
The most straightforward way to get the length of a list in Python is by using the built-in len()
function. This function takes a single argument, which is the list you want to measure, and returns the number of elements in that list. It’s simple, efficient, and a standard practice in Python programming.
Here’s how you can use it:
my_list = [10, 20, 30, 40, 50]
length_of_list = len(my_list)
print(length_of_list)
Output:
5
In this example, we created a list named my_list
containing five integers. By passing my_list
to the len()
function, we received the output 5
, indicating that there are five elements in the list. This method is not only concise but also highly efficient. The len()
function operates in constant time, O(1), meaning it retrieves the length without needing to iterate through the list. This makes it the preferred method for obtaining a list’s length in Python.
Using a Loop to Count Elements
While the len()
function is the most efficient way to get the length of a list, you might encounter situations where you want to manually count the elements, perhaps for educational purposes or to understand the underlying mechanics. You can achieve this using a simple loop.
Here’s an example:
my_list = [10, 20, 30, 40, 50]
count = 0
for item in my_list:
count += 1
print(count)
Output:
5
In this code snippet, we initialize a counter variable count
to zero. We then loop through each item in my_list
, incrementing count
by one for each iteration. After the loop completes, we print the value of count
, which gives us the total number of elements in the list. While this method is more verbose and less efficient than using len()
, it serves as a great demonstration of how lists work in Python. It also provides insights into iteration and counting, which are fundamental programming concepts.
Using List Comprehension with the sum() Function
Another interesting approach to get the length of a list is by using list comprehension combined with the sum()
function. This method is less common but can be useful in specific scenarios where you might want to apply conditions while counting.
Here’s how you can do it:
my_list = [10, 20, 30, 40, 50]
length_of_list = sum(1 for _ in my_list)
print(length_of_list)
Output:
5
In this example, we use a generator expression within the sum()
function. The expression 1 for _ in my_list
generates a 1
for each element in my_list
, and sum()
adds them up. The result is the total number of elements in the list. This method is quite elegant and showcases Python’s capabilities in handling iterations and functional programming styles. However, it’s worth noting that this approach is less efficient than using len()
since it involves creating an additional generator and iterating through the list.
Conclusion
Determining the length of a list in Python is a fundamental skill that every programmer should master. While the len()
function is the most efficient and straightforward method, understanding how to count elements using loops or list comprehensions can deepen your understanding of Python’s capabilities. Whether you are a beginner or an experienced developer, these techniques will enhance your coding toolkit and make your list management tasks more efficient. Now that you know how to get the length of a list, you can apply these methods in your projects with confidence.
FAQ
-
How do I get the length of an empty list?
You can use thelen()
function. For example,len([])
will return0
. -
Can I use
len()
on other data types?
Yes,len()
can be used on strings, tuples, and dictionaries to get their respective lengths. -
Is there a performance difference between using
len()
and counting with a loop?
Yes,len()
is much faster as it operates in constant time, while counting with a loop takes linear time. -
How can I get the length of a nested list?
You can uselen()
on the outer list. To count elements in nested lists, you would need to iterate through each sublist.
- Are there any limitations to using
len()
?
No,len()
works on any iterable object in Python, making it a versatile function.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python