How to Check List Equality in Python
-
Check Equality of Lists in Python Using the Equality
==
Operator -
Check Equality of Arrays in Python Using the Equality
==
Operator and thenumpy.all()
Method -
Check Equality of Arrays in Python Using
all()
and==
-
Check Equality of Arrays in Python Using
numpy.array_equal()
-
Check Equality of Arrays in Python Using
collections.Counter()
-
Check Equality of Arrays in Python Using
operator.countOf()
-
Check Equality of Arrays in Python Using
reduce()
andmap()
-
Check Equality of Arrays in Python Using
list.sort()
and the==
Operator - Conclusion
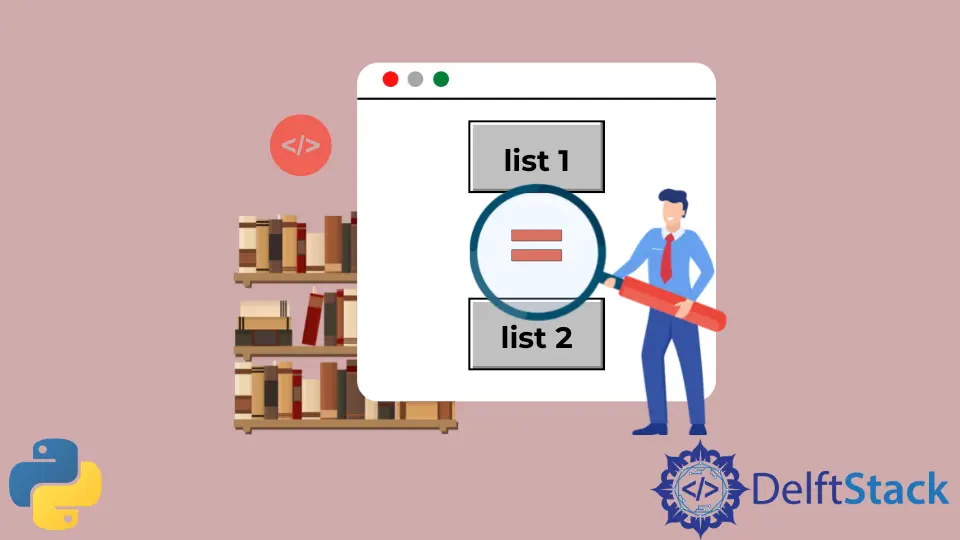
When working with lists in Python, it’s often crucial to determine whether two lists are equal or identical. For the two lists to be equal, each element of the first list should be equal to the second list’s corresponding element.
Also, if the two lists have the same elements, but the sequence is not the same, they will not be considered equal or identical lists.
Python provides various methods for list comparison, each catering to different scenarios and requirements. In this tutorial, we will explore several approaches, including the equality ==
operator, NumPy, collections.Counter()
, operator.countOf()
, reduce()
, map()
, and list.sort()
combined with ==
.
Let’s dive into each method with code examples and explanations.
Check Equality of Lists in Python Using the Equality ==
Operator
A simple way to check the equality of the two lists in Python is by using the equality ==
operator. This operator is a comparison operator in Python that returns True
if the operands are equal and False
otherwise.
When applied to lists, it compares the elements at corresponding indices in both lists.
The below example code demonstrates how to use the equality ==
operator to check if the two lists are equal in Python.
a = [4, 7, 3, 5, 8]
b = [4, 7, 3, 5, 8]
c = [1, 7, 3, 5, 2]
print(a == b)
print(a == c)
Output:
True
False
In this example, we have three lists—a
, b
, and c
. The next two lines use the print
function to output the results of the equality comparisons.
The expression a == b
evaluates to True
because both lists have the same elements in the same order. On the other hand, the expression a == c
evaluates to False
because the elements of lists a
and c
differ, even though they have the same length.
Element-wise Comparison Using the Equality ==
Operator and NumPy
Now, let us look into the scenario where we want to get element-wise results. Suppose we want to check which corresponding elements of the second array are equal and which are not equal.
For this, we first need to convert the lists to the NumPy
array using the np.array()
method and then use the equality ==
operator, which will return True
or False
for each element.
The below example code demonstrates how to check if the elements of two lists are equal or not in Python.
import numpy as np
a = [4, 7, 3, 5, 8]
b = [4, 7, 3, 5, 8]
c = [1, 7, 3, 5, 2]
print((np.array(a) == np.array(b)))
print((np.array(a) == np.array(c)))
Output:
[ True True True True True]
[False True True True False]
In this code, we have the same lists: a
, b
, and c
. We then utilize NumPy’s np.array()
method to convert these lists into NumPy arrays. This conversion facilitates element-wise operations and comparisons.
The first print statement uses the equality ==
operator within the context of NumPy arrays to check for element-wise equality between arrays a
and b
. The result is a NumPy array of boolean values, where each element represents whether the corresponding elements in a
and b
are equal.
The second print statement similarly compares arrays a
and c
element-wise, producing a boolean array as a result. As you can see in the output, the elements at corresponding positions in arrays a
and c
are not equal for all positions except the second and the rest.
This approach provides a detailed understanding of the equality of elements at corresponding positions in the arrays. The resulting boolean arrays can be useful for identifying differences between datasets or verifying specific conditions in your Python program.
Check Equality of Arrays in Python Using the Equality ==
Operator and the numpy.all()
Method
In many cases, we use the NumPy
arrays for different tasks. If we use the equality ==
operator to check the equality, we will get the element-wise result, as shown in the above example code.
Therefore, to check the equality of the NumPy
arrays in Python, the numpy.all()
method has to be used to check the arrays’ equality. The np.all()
method returns True
if the elements along the given axis evaluate to True
and returns False
otherwise.
The below example code demonstrates how to check whether the two arrays are equal or not in Python.
import numpy as np
a = np.array([1, 6, 4, 8, 3])
b = np.array([1, 6, 4, 8, 3])
c = np.array([1, 4, 8, 2, 3])
print((a == b).all())
print((a == c).all())
Output:
True
False
In the provided code snippet, the first three lines of code import the NumPy library and create three NumPy arrays: a
, b
, and c
.
Then, the expression (a == b).all()
checks if all corresponding elements of arrays a
and b
are equal. Since the arrays are identical, this expression evaluates to True
.
Conversely, the expression (a == c).all()
checks if all corresponding elements of arrays a
and c
are equal. As the elements differ between a
and c
, this expression evaluates to False
.
Check Equality of Arrays in Python Using all()
and ==
Another way to check whether two lists are identical or equal in Python is by using the all()
function in conjunction with the == operator. This approach provides a concise way to determine if two lists contain the same elements in the same order.
In Python, the all()
function returns True
if all elements in an iterable are true. Combining this with the ==
operator allows us to check if the corresponding elements in two lists are equal.
The syntax for this comparison is as follows:
result = all(x == y for x, y in zip(list1, list2))
Here, zip(list1, list2)
pairs elements from list1
and list2
together. The all()
function then checks if all pairs are equal.
Code example:
def are_lists_equal(list1, list2):
result = all(x == y for x, y in zip(list1, list2))
return result
list_a = [1, 2, 3, 4, 5]
list_b = [1, 2, 3, 4, 5]
lists_equal = are_lists_equal(list_a, list_b)
print("Are the lists equal? {}".format(lists_equal))
In the provided code, the are_lists_equal
function takes two lists, list1
and list2
, as arguments. Inside the function, the zip(list1, list2)
function pairs corresponding elements from both lists together.
The all()
function then iterates through these pairs, checking if each element in list1
is equal to the corresponding element in list2
. The result is a boolean value stored in the variable result
.
In the example lists list_a
and list_b
, which are identical, the function returns True
. If the lists were not identical, the result would be False
.
Output:
Are the lists equal? True
Feel free to modify the example lists and observe how the output changes based on the equality of the lists.
Check Equality of Arrays in Python Using numpy.array_equal()
While Python provides built-in tools for list comparison, the numpy
library offers a specialized function, numpy.array_equal()
, to effortlessly check if two lists are identical or equal. This method is particularly powerful when dealing with numerical data and multi-dimensional arrays.
In the context of Python lists, we can utilize this function by first converting the lists into NumPy arrays. The syntax is as follows:
import numpy as np
result = np.array_equal(list1, list2)
Here, list1
and list2
are converted into NumPy arrays, and np.array_equal()
then checks if the arrays are equal.
Code example:
import numpy as np
def are_lists_equal_np(list1, list2):
result = np.array_equal(list1, list2)
return result
list_a = [1, 2, 3, 4, 5]
list_b = [1, 2, 3, 4, 5]
lists_equal_np = are_lists_equal_np(list_a, list_b)
print("Are the lists equal? {}".format(lists_equal_np))
In this example, the are_lists_equal_np
function takes two lists, list1
and list2
, as arguments. The import numpy as np
statement brings the NumPy library into the script, allowing us to use its functions.
Inside the function, np.array_equal(list1, list2)
directly compares the NumPy arrays created from list1
and list2
. The result is a boolean value stored in the variable result
, which is then returned.
The provided example lists, list_a
and list_b
, are identical, resulting in the function returning True
. Again, if the lists were not identical, the result would be False
.
Output:
Are the lists equal? True
Check Equality of Arrays in Python Using collections.Counter()
The collections.Counter()
class offers another efficient approach to determine if two lists are identical or equal. This method is particularly valuable when the order of elements is not crucial, as Counter
disregards the order and focuses solely on the occurrence of elements.
This class provides a convenient way to count the occurrences of elements in a list. To check if the two lists are identical, we can use Counter
to create dictionaries of element counts and then compare these dictionaries.
The syntax is as follows:
from collections import Counter
result = Counter(list1) == Counter(list2)
Here, Counter(list1)
and Counter(list2)
create dictionaries of element counts for each list, and the ==
operator then checks if the dictionaries are equal.
Code example:
from collections import Counter
def are_lists_equal_counter(list1, list2):
result = Counter(list1) == Counter(list2)
return result
list_a = [1, 2, 3, 4, 5]
list_b = [1, 2, 3, 4, 5]
lists_equal_counter = are_lists_equal_counter(list_a, list_b)
print("Are the lists equal? {}".format(lists_equal_counter))
Inside the are_lists_equal_counter()
function, Counter(list1)
and Counter(list2)
create dictionaries that represent the counts of elements in each list. The ==
operator then checks if these dictionaries are equal, and the result is a boolean value stored in the variable result
, which is returned.
For this example, list_a
and list_b
are identical, so the function returns True
. Otherwise, the result would be False
.
Output:
Are the lists equal? True
Check Equality of Arrays in Python Using operator.countOf()
The operator
module provides another handy method called countOf()
for comparing lists in Python. This function facilitates the counting of occurrences of a specific element in a list.
By comparing the counts of each element in two lists, we can determine if the lists are identical.
The syntax is as follows:
import operator
result = all(
operator.countOf(list1, elem) == operator.countOf(list2, elem)
for elem in set(list1 + list2)
)
Here, operator.countOf(list1, elem)
and operator.countOf(list2, elem)
count the occurrences of elem
in list1
and list2
respectively. The all()
function ensures that the counts are the same for all unique elements in the combined lists.
Code example:
import operator
def are_lists_equal_operator(list1, list2):
result = all(
operator.countOf(list1, elem) == operator.countOf(list2, elem)
for elem in set(list1 + list2)
)
return result
list_a = [1, 2, 3, 4, 5]
list_b = [1, 2, 3, 4, 5]
lists_equal_operator = are_lists_equal_operator(list_a, list_b)
print("Are the lists equal? {}".format(lists_equal_operator))
In this code example, the are_lists_equal_operator
function also takes two lists, list1
and list2
, as arguments. Inside the function, set(list1 + list2)
creates a set containing all unique elements present in both lists, which is iterated over using a generator expression within the all()
function.
For each unique element, represented by the variable elem
, the operator.countOf(list1, elem)
and operator.countOf(list2, elem)
functions count the occurrences of that element in both list1
and list2
, respectively. The equality of these counts is checked, ensuring that the lists have the same occurrences of each unique element.
The all()
function ensures that this equality check holds for all unique elements in the combined set. If the counts match for every element, the variable result
is set to True
. Otherwise, it is set to False
.
Finally, the function returns the value of result
, indicating whether the two lists are equal in terms of element occurrences.
Output:
Are the lists equal? True
Check Equality of Arrays in Python Using reduce()
and map()
The reduce()
and map()
functions can also be combined to create a concise method for list comparison in Python. These functions provide a functional programming approach to iterate and reduce a list to a single value, making them useful for comparing the elements of two lists.
The reduce()
function from the functools
module is employed to iteratively apply a specified function to the elements of a list. When combined with map()
, which applies a given function to all items in an iterable, we can compare corresponding elements from two lists.
The syntax is as follows:
from functools import reduce
result = reduce(lambda x, y: x and y, map(lambda a, b: a == b, list1, list2), True)
Here, the map(lambda a, b: a == b, list1, list2)
applies the equality check function to corresponding elements of list1
and list2
. The reduce()
function then iteratively performs a logical AND
operation (and
) on the results, ensuring all elements are equal.
Code example:
from functools import reduce
def are_lists_equal_reduce_map(list1, list2):
result = reduce(lambda x, y: x and y, map(lambda a, b: a == b, list1, list2), True)
return result
list_a = [1, 2, 3, 4, 5]
list_b = [1, 2, 3, 4, 5]
lists_equal_reduce_map = are_lists_equal_reduce_map(list_a, list_b)
print("Are the lists equal? {}".format(lists_equal_reduce_map))
Firstly, the from functools import reduce
statement imports the reduce()
function from the functools
module, making it available for use in the script.
As you can see inside the are_lists_equal_reduce_map
function, the map(lambda a, b: a == b, list1, list2)
applies the lambda function to corresponding elements of both lists, generating a list of boolean values indicating whether each pair of elements is equal.
The reduce(lambda x, y: x and y, ..., True)
function is then used to iteratively apply a logical AND
operation (and
) on the boolean values produced by the map()
function. The True
at the end serves as the initial value for the reduction process.
The result of this reduction is a single boolean value stored in the variable result
, representing whether all elements in the two lists are equal. This result is then returned by the function.
Output:
Are the lists equal? True
Check Equality of Arrays in Python Using list.sort()
and the ==
Operator
Comparing two lists for equality can also be achieved using the list.sort()
method in combination with the ==
operator. This approach is particularly useful when the order of elements is crucial for determining equality.
The list.sort()
method is used to sort the elements of a list in ascending order. By sorting both lists and subsequently comparing them using the ==
operator, we can efficiently check if the elements are not only the same but also arranged in the same order.
The syntax is as follows:
list1.sort()
list2.sort()
result = list1 == list2
Here, list1.sort()
and list2.sort()
sort the elements of both lists in place, and list1 == list2
then checks if the sorted lists are equal.
Code example:
def are_lists_equal_sort(list1, list2):
list1.sort()
list2.sort()
result = list1 == list2
return result
list_a = [5, 2, 3, 1, 4]
list_b = [1, 2, 3, 4, 5]
lists_equal_sort = are_lists_equal_sort(list_a, list_b)
print("Are the lists equal? {}".format(lists_equal_sort))
In this code example, list1.sort()
and list2.sort()
sort the elements of both given lists in ascending order. The ==
operator then compares the sorted lists element-wise, checking if they are equal.
The result of this comparison is stored in the variable result
, which is then returned by the function. If the two lists are identical, including the order of elements, the result is True
; otherwise, it is False
.
For the example lists, list_a
and list_b
, even though they are not in the same order initially, sorting them with are_lists_equal_sort
results in equal lists, so the function returns True
.
Output:
Are the lists equal? True
Conclusion
In conclusion, Python provides multiple methods for comparing lists, each suited to specific scenarios. Consider the nature of your data and the requirements of your comparison to choose the most appropriate method.
Whether it’s straightforward equality, element-wise comparison, or disregarding order, Python offers versatile tools for efficient list comparison.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python