if...else in Python List Comprehension
- Understanding List Comprehension
- Using if…else in List Comprehension
- Filtering with if…else
- Conclusion
- FAQ
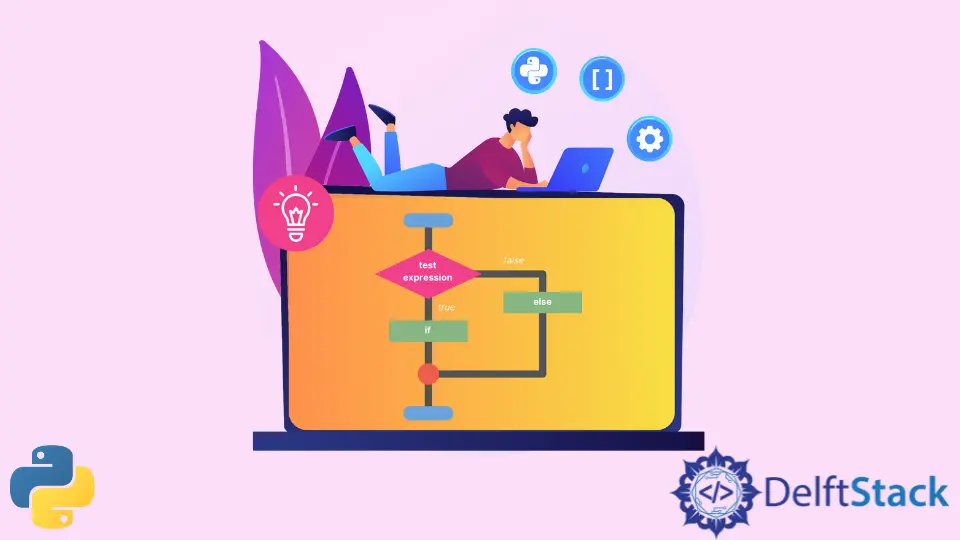
List comprehension is one of Python’s most powerful features, allowing developers to create new lists from existing ones in a concise and readable way. One of the most common use cases for list comprehension is when you need to apply conditional logic to the elements being processed.
This tutorial will guide you through using the if…else statement within list comprehensions, showcasing how to create lists based on specific conditions. Whether you’re filtering data or transforming values, mastering this technique will enhance your Python programming skills and streamline your code. Let’s dive into the world of Python list comprehensions with if…else statements!
Understanding List Comprehension
List comprehension is a compact way to process all or part of the elements in a sequence and return a list. The basic syntax is:
[expression for item in iterable if condition]
In this structure, the expression defines what to include in the new list, the item is the current element being processed, and the iterable is the collection being traversed. The optional condition filters items, allowing you to include only those that meet specific criteria.
However, when you want to include an else clause, the syntax changes slightly:
[expression_if_true if condition else expression_if_false for item in iterable]
This format allows you to specify two expressions based on whether the condition is true or false. Understanding how to utilize this can significantly simplify your code.
Using if…else in List Comprehension
Let’s explore how to effectively use the if…else statement in list comprehensions with some practical examples. This will help you understand how to apply conditional logic while generating lists.
Example 1: Categorizing Numbers
Suppose you have a list of numbers and you want to categorize them as “Even” or “Odd”. You can achieve this using list comprehension with an if…else statement.
numbers = [1, 2, 3, 4, 5]
categories = ["Even" if num % 2 == 0 else "Odd" for num in numbers]
print(categories)
Output:
['Odd', 'Even', 'Odd', 'Even', 'Odd']
In this example, we have a list of integers. The list comprehension iterates through each number, checking if it is even or odd. If the number is even (i.e., num % 2 == 0
), it adds “Even” to the new list; otherwise, it adds “Odd”. The result is a new list that categorizes each number accordingly. This technique is not only concise but also enhances readability.
Example 2: Transforming Values
Let’s say you want to create a new list that doubles the values of positive numbers and replaces negative numbers with zero. Here’s how to do it:
values = [-5, 3, -1, 7, 0]
transformed = [value * 2 if value > 0 else 0 for value in values]
print(transformed)
Output:
[0, 6, 0, 14, 0]
In this case, the list comprehension checks each value in the original list. If the value is greater than zero, it doubles it; otherwise, it replaces it with zero. This approach allows for efficient transformation of lists while maintaining clarity in your code. Such conditional transformations are common in data processing tasks.
Filtering with if…else
Filtering elements based on conditions is another powerful application of list comprehensions. Let’s see how to use if…else to filter and transform data simultaneously.
Example 3: Filtering and Labeling
Imagine you have a list of ages and want to label them as “Adult” or “Minor”. Here’s how you can do this:
ages = [12, 18, 20, 15, 30]
labels = ["Adult" if age >= 18 else "Minor" for age in ages]
print(labels)
Output:
['Minor', 'Adult', 'Adult', 'Minor', 'Adult']
In this example, the list comprehension goes through each age in the ages
list. It checks if the age is 18 or older and labels it as “Adult”; otherwise, it labels it as “Minor”. This is a great way to generate a new list based on conditions without needing to write multiple lines of code. The clarity and brevity of this approach make it a favorite among Python developers.
Example 4: Handling Mixed Data Types
Sometimes, you might have a list with mixed data types, and you want to filter out non-numeric values while doubling the numeric ones. Here’s how you can handle that:
mixed_data = [1, 'two', 3, 'four', 5]
processed = [item * 2 if isinstance(item, int) else "Not a number" for item in mixed_data]
print(processed)
Output:
[2, 'Not a number', 6, 'Not a number', 10]
In this case, the list comprehension checks each item to see if it’s an integer. If it is, it doubles the value; if not, it replaces it with “Not a number”. This method is particularly useful when cleaning data or preparing it for analysis, as it allows you to maintain control over the types of data being processed.
Conclusion
Mastering the use of if…else statements within list comprehensions is a valuable skill for any Python developer. It allows for cleaner, more efficient code that can handle complex logic in a single line. As you practice these techniques, you’ll find that they can significantly enhance your coding efficiency and readability. Whether you’re processing data, filtering lists, or transforming values, incorporating conditional logic in your list comprehensions will elevate your Python programming abilities. Happy coding!
FAQ
-
What is list comprehension in Python?
List comprehension is a concise way to create lists by iterating over an iterable and applying an expression to each item. -
Can I use multiple conditions in list comprehensions?
Yes, you can use multiple conditions by combining them with logical operators likeand
andor
. -
Is list comprehension faster than traditional loops?
Generally, list comprehensions are faster and more efficient than traditional for loops because they are optimized for performance.
-
Can I use functions inside list comprehensions?
Absolutely! You can call functions within the expression part of a list comprehension. -
What are the benefits of using if…else in list comprehensions?
Using if…else allows for more complex logic and transformations in a single line, improving code readability and efficiency.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python