How to Get the Filename and a Line Number in Python
-
Using the
__file__
and__line__
Attributes -
Using the
__file__
Attribute and the__LINE__
Variable -
Using the
traceback
Module -
Using the
inspect
Module - Conclusion
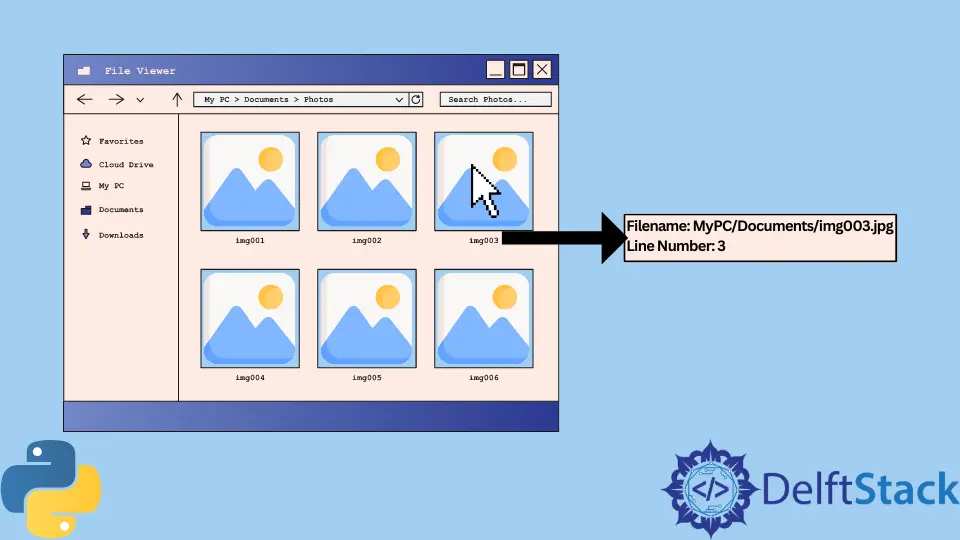
When working on real-world applications or side projects, we often have to retrieve line numbers and filenames for debugging purposes. Generally, this is done to understand what code is getting executed, to analyze the flow of control of any application, and to fasten the debugging process.
In this article, we will learn how to get a line number and the filename of the Python script using the __file__
and __line__
attributes, the __file__
attribute and __LINE__
variable, the traceback
module, and the inspect
module.
Using the __file__
and __line__
Attributes
The first method, using the __file__
and __line__
attributes, is concise and straightforward, especially when using Python 3.8 or above. It directly retrieves the filename and line number, making it easy to integrate into your code.
You can use the __file__
attribute to get the current script’s file name. On the other hand, the line number can be accessed using the inspect
module or by directly including the line number in the code.
Here is the example code for better understanding.
import inspect
current_filename = __file__
current_line = inspect.currentframe().f_lineno
print(f"Filename: {current_filename}, Line number: {current_line}")
Output:
Filename: main.py, Line number: 4
The __file__
provides the filename of the current script, while the __line__
(for Python 3.8 and above) represents the current line number.
Using the __file__
Attribute and the __LINE__
Variable
The second method consists of the __file__
attribute and __LINE__
variable, which provide a concise and direct means of retrieving the file name and line number, respectively.
Introduced in Python 3.8, the __LINE__
variable simplifies the process, offering a streamlined approach to enhance code traceability and get the current line number.
Here is the example code for better understanding.
current_filename = __file__
current_line = __line__
print(f"Filename: {current_filename}, Line number: {current_line}")
Output:
Filename: <your_script_name.py>, Line number: <current_line_number>
Similar to the first method, this approach is concise and effective, leveraging the __LINE__
variable to obtain the line number directly.
Using the traceback
Module
The third method introduces the traceback
module, which emerges as a power tool in this discussion.
This method allows developers to extract detailed information about the execution stack, including the filenames and line numbers associated with exceptions.
Here is the example code for better understanding.
import traceback
try:
raise Exception("Getting traceback")
except Exception as e:
trace = traceback.extract_tb(e.__traceback__)[-1]
current_filename, current_line, _, _ = trace
print(f"Filename: {current_filename}, Line number: {current_line}")
Output:
Filename: <your_script_name.py>, Line number: <current_line_number>
This example uses traceback.extract_tb
to extract the traceback information and e.__traceback__
to retrieve the traceback object associated with the caught exception using except Exception as e
.
This method provides a more detailed traceback, extracting information related to the filename and line number from the exception’s traceback.
Using the inspect
Module
The fourth method uses the inspect
module Python. The inspect
module contains several utilities to fetch information about objects, classes, methods, functions, frame objects, and code objects.
This library has a getframeinfo()
method that retrieves information about a frame or a traceback object. This method accepts a frame
argument about which it retrieves details.
The currentFrame()
method returns the frame object for the caller’s stack frame. We can use these utilities for our use case.
Refer to the following Python code to understand the usage.
from inspect import currentframe, getframeinfo
frame = getframeinfo(currentframe())
filename = frame.filename
line = frame.lineno
print("Filename:", filename)
print("Line Number:", line)
Output:
Filename: full/path/to/file/main.py
Line Number: 3
As we can see, the filename
attribute will return the full path to the Python file.
In my case, the Python file’s name was main.py
; hence, it shows main.py
in the output. And the lineno
attribute returns the line number at which this frame = getframeinfo(currentframe())
statement was executed.
The mentioned statement was executed at line 3
; hence, the output has a 3
after the Line Number
label.
Conclusion
In this discussion, we have tackled the four methods using __file__
and __line__
attributes, __file__
attribute and __LINE__
variable, traceback
module, and inspect
module.
The __file__
and __line__
attributes offer a simple and direct way to access file and line information, promoting code clarity. Introducing the __LINE__
variable, while not native to Python, can be a useful extension for developers accustomed to this convention in other programming languages.
The traceback
module proves invaluable for comprehensive traceback information, facilitating effective error diagnosis and resolution. Similarly, the inspect
module enhances introspection capabilities, empowering developers with dynamic access to the code structure.
Each method caters to distinct needs, contributing to a well-rounded approach for code analysis, debugging, and maintenance in Python programming. Depending on your specific needs, you can choose the method that best aligns with your debugging strategy.