Python Lambda With Multiple Lines
-
Understanding the
lambda
Function in Python -
the Multiline
lambda
Function in Python -
Use Parentheses and Backslashes to Write Python
lambda
With Multiple Lines -
Use Explicit
return
Statements to Write Pythonlambda
With Multiple Lines -
Use Helper Functions to Write Python
lambda
With Multiple Lines -
Use the
def
Keyword Instead of thelambda
Function in Python - Conclusion
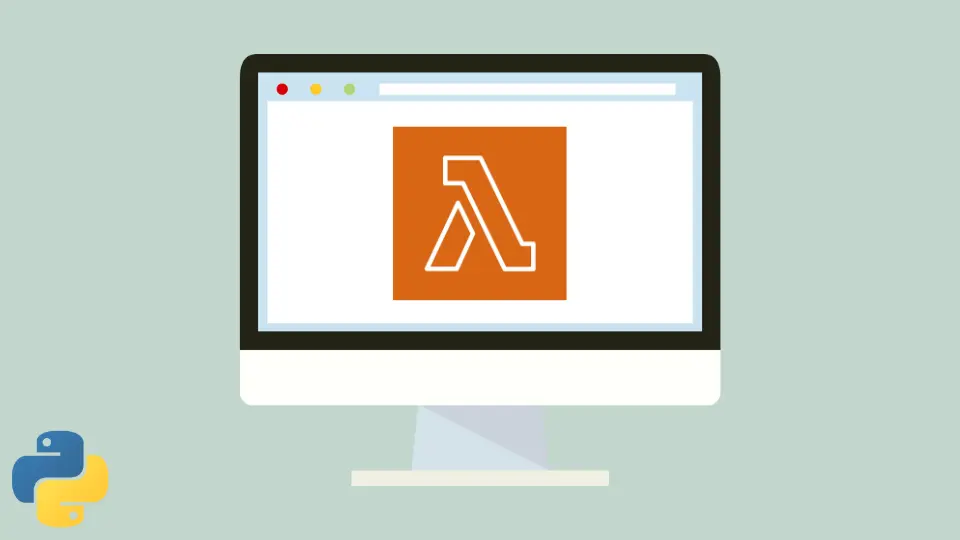
Lambda
functions, also known as anonymous functions, are a concise way to define small, one-line, and throwaway functions without a formal function definition in Python. They offer a compact and efficient approach to solving problems in Python programming.
However, there are situations where a single line isn’t enough to capture the complexity of a task. In this article, we’ll explore different methods for creating multiline lambdas
in Python, providing example codes and detailed explanations for each approach.
Understanding the lambda
Function in Python
We normally use the def
keyword to define our functions in Python, but Python provides an anonymous function known as the lambda
function. This function has no name.
The lambda
function is a small and restricted function written in one line. The lambda
function can have multiple arguments, like a normal function with one expression.
We use the lambda
function in Python to construct anonymous functions. An anonymous function consists of three main parts:
- The
lambda
keyword - Parameters
- Function body
We can use any number of parameters in a lambda
function, but the body must contain only one expression. A lambda
function is written in one line, which can be called immediately.
Syntax:
lambda x, y: x + y
In the above syntax, x
and y
are parameters, and x + y
is an expression in a lambda
function. Now, let’s discuss how we can call it immediately.
The following code snippet shows how we can use a lambda
function in Python.
def addVar(x, y):
return x + y
print(addVar(2, 3))
Output:
The above code defines a lambda
function called addVar
that takes two arguments, x
and y
, and returns their sum x + y
. The print
statement calls the addVar
function with arguments 2 and 3, resulting in an output of 5.
In other words, it adds the values 2 and 3 together and prints the result.
Why Do We Need lambda
Functions
Firstly, they provide a compact, readable way to express simple, one-line functions.
Instead of defining a separate function for a short code, we can define it inline using a lambda
function. This reduces the clutter in our code and improves its readability.
Furthermore, lambda
functions are commonly used in situations where we need to pass a function as an argument to another function, such as in the map()
, filter()
, and sort()
functions. They offer a convenient way to define small, specialized functions without a complete definition.
To better understand the practical application of lambda
functions, let’s consider a real-world example: sorting a list of dictionaries. Suppose we have a list of dictionaries representing various books, each with a title
and author
key.
If we want to sort this list based on the book titles, we can utilize a lambda
function to specify the sorting key. By passing a lambda
function to the sorted()
function, we can easily achieve this sorting operation without defining a separate named function.
the Multiline lambda
Function in Python
Python’s lambda
functions are renowned for their conciseness and convenience, allowing us to define anonymous functions effortlessly. However, there are situations where the simplicity of a single line falls short, requiring more complex logic.
This is where the multiline lambda
function steps in, empowering us with the ability to express intricate operations in a concise yet flexible manner.
By breaking free from the constraints of a single line, we can leverage the multiline lambda
function to tackle complex tasks, implement conditional statements, perform iterative operations, and unleash the full potential of functional programming paradigms in Python.
We can enclose our expression in parentheses to use a multiline lambda
function. The following code snippet shows how we can use a multiline lambda
function in Python.
def addVar(x, y):
return x + y # addition operation
print(addVar(2, 3))
Output:
In the above code, the lambda
function addVar
takes two arguments, x
and y
. Instead of defining the function on a single line, we utilize parentheses to span multiple lines.
This allows us to include comments and break down the logic into multiple steps if needed. In this case, the lambda
function adds x
and y
.
The result is then printed using the print
statement.
lambda
functions in Python is generally discouraged because it reduces code readability and goes against the purpose of lambda
functions, which is to provide concise and simple expressions.Use Parentheses and Backslashes to Write Python lambda
With Multiple Lines
One straightforward way to write a multiline lambda
is by enclosing the expression within parentheses and using backslashes to continue the code on the next line. This method is suitable for splitting a lambda
function into multiple lines for improved readability.
def multi_line_lambda(x, y):
return x + y - (x * y) / (x + y)
result = multi_line_lambda(5, 3)
print(result)
Output:
6.125
The lambda
function takes two parameters, x
and y
. The expression is enclosed within parentheses.
Backslashes \
are used to continue the expression on the next line. The result is the sum of x
and y
minus the division of x * y
by x + y
.
Use Explicit return
Statements to Write Python lambda
With Multiple Lines
Another method for creating multiline lambdas
is by using explicit return
statements. While lambdas
usually implicitly return the result of the expression, using the return
keyword allows you to have multiple lines in the lambda
body.
def multi_line_lambda(x, y):
return (x + y, x - y)
result_sum, result_diff = multi_line_lambda(8, 3)
print("Sum:", result_sum)
print("Difference:", result_diff)
Output:
Sum: 11
Difference: 5
The lambda
function takes two parameters, x
and y
. The expression now contains two calculations separated by a comma.
The lambda
returns a tuple with the sum and difference of x
and y
. Unpacking the tuple into two variables allows access to each result individually.
Use Helper Functions to Write Python lambda
With Multiple Lines
For more complex logic, you can define helper functions inside the lambda
to break down the problem into smaller, manageable parts. This method enhances readability and maintainability.
def multi_line_lambda(x, y):
return (
lambda a, b: a * b, # Helper function 1
lambda c, d: c + d, # Helper function 2
)
multiply, add = multi_line_lambda(4, 6)
result = multiply(3, 2) + add(5, 1)
print(result)
Output:
12
The lambda
function takes two parameters, x
and y
. Instead of complex expressions, two helper lambdas
are defined within the main lambda
.
The helper lambdas
perform multiplication and addition separately. The main lambda
returns the two helper lambdas
.
The results are calculated by invoking the helper lambdas
and combining the results.
Use the def
Keyword Instead of the lambda
Function in Python
The lambda
function can only be written in one line of code; it can surely have multiple variables, but the lambda
function consists of only one expression. If you want to write a function that can be written in multiple lines, you can use the def
keyword instead of lambda
.
Let’s go through an example in which we will create a new function that consists of multiple lines, as shown below.
def checkVal(x):
if x < 5:
print("X is smaller than 5")
if x > 5:
print("X is greater than 5")
checkVal(4)
Output:
From the above code example, if we want a function that can be written in one expression, we can use the lambda
function, but if we need to write a function in multiple expressions and lines, we need to use def
instead of lambda
.
Conclusion
We delved into the world of Python’s lambda
functions, which provide a concise and anonymous way to create one-line functions. While powerful for simplicity, there are cases where a single line falls short.
We explored various methods for crafting multiline lambda
functions, including the use of parentheses and backslashes, explicit return
statements, helper functions, and even a block of code within a lambda
.
These techniques, though offering flexibility, are generally discouraged due to potential readability issues.
It’s essential to prioritize clear code over brevity. Despite the versatility provided by these methods, transitioning to regular named functions with the def
keyword might be a more readable solution for intricate logic.
In practice, understanding both the simplicity of one-line lambda
functions and the approaches to handle complexity equips Python developers with a versatile toolkit for coding challenges.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn