How to Use Await in a Python Lambda
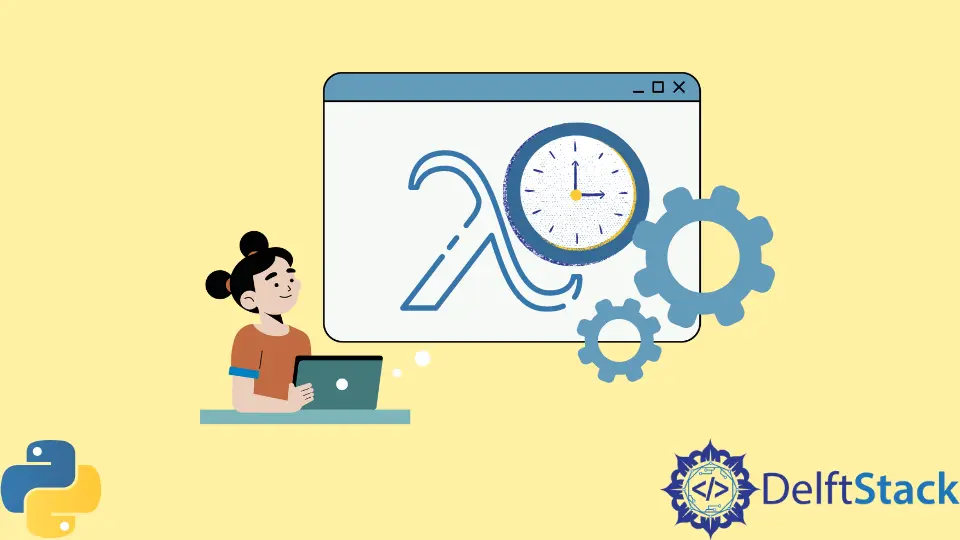
Asynchronous programming is not multithreading or multiprocessing. Instead, it is concurrent programming where we can run a potentially long-running task and allow our program to be responsive to other tasks while that task is still running instead of waiting for the completion.
With asynchronous programming, HTTP requests actions or user selection can take a lot of time, and therefore, it would be intelligent to allow other tasks to run while such actions are still being done.
In Python, to achieve asynchronous programming, you can use the async/await
features with functions, but we do such with lambda
functions. This article will discuss the possibility of using await
in a Python lambda function.
No async/await lambda
in a Python Lamda
To carry out asynchronous programming in Python, we need a built-in high-level package called asyncio
that uses the async/await
syntax to allow for concurrent programming.
To create an asynchronous operation, you can use the async
and await
keywords.
import asyncio
async def main():
print("Action One")
await asyncio.sleep(1)
print("Action Two")
asyncio.run(main())
Output:
Action One
Action Two
We applied the async
keyword to the main()
function and the await
keyword on the asyncio.sleep()
statement; however, how do we apply it to an anonymous function via lambda
? Anonymous or lambda
functions are defined without a name and use the lambda
keyword to achieve that.
Say you want to remove from your list the numbers that are not divisible by 3. We can use the filter()
method (a higher-order function) that takes another function.
So instead of defining a function binding that you might not use again, you can use an anonymous function using the lambda
keyword.
myNums = [1, 2, 3, 4, 5, 6, 7]
myNums = list(filter(lambda x: x % 3 == 0, myNums))
print(myNums)
Output:
[3, 6]
However, is there a way to add the asynchronous capabilities to the lambda
function? The simple answer is No.
The developers of Python have stated that there are no significant use cases to justify adding the async
syntax for lambda
.
Also, the naming of lambda
isn’t the proper use of lambda
functions; therefore, it would be hard to use it functionally with lambda
functions. In addition, passing an async
function via a lambda
function will be executed within a synchronous function.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn