Python Lambda Closure
- Syntax to Use Lambda Function in Python
- Use Lambda Function in Python
- Use Closure Function in Python
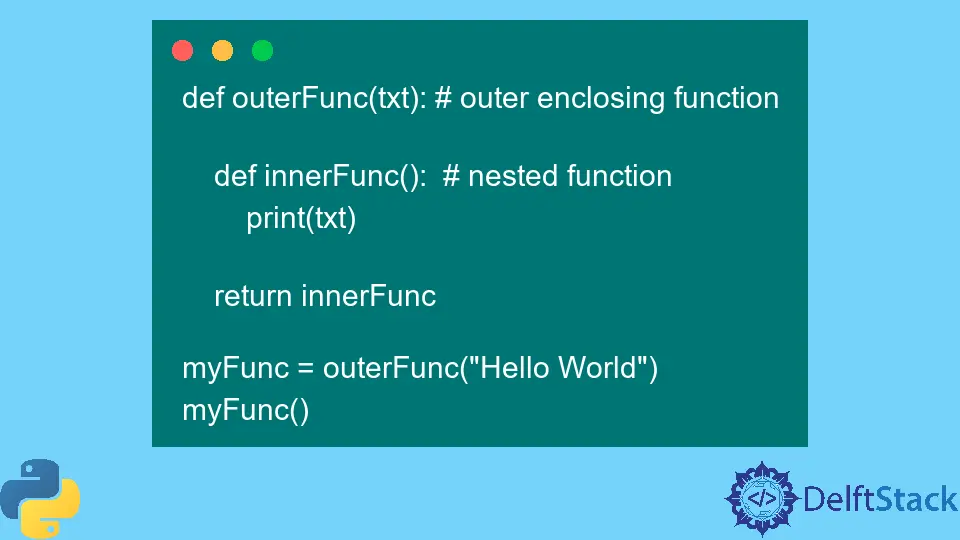
A lambda function is defined without a name. It is also known as an anonymous function in Python.
We use a def
keyword to define a regular function in Python, whereas the lambda
keyword is used to define a lambda function.
This tutorial will teach you to use lambda function and closure in Python.
Syntax to Use Lambda Function in Python
The syntax for lambda function in Python is:
lambda arguments: expression
A lambda function can have only multiple arguments but only one expression. It evaluates the expression and returns the result.
Use Lambda Function in Python
The following example adds 5 to the given value and prints the result.
def total(a):
return a + 5
print(total(10))
Output:
15
Add multiple arguments and return the result.
def total(a, b, c):
return a + b + c
print(total(10, 15, 20))
Output:
45
In the above example, lambda a,b,c: a+b+c
is a lambda function where a,b,c
are arguments and a+b+c
is an expression. The expression is calculated and displayed in the output.
The lambda function has no name and is assigned to a variable total
.
This line:
def total(a, b, c):
return a + b + c
It is equivalent to this:
def total(a, b, c):
return a + b + c
Use Closure Function in Python
Before learning closure, you must first understand Python’s concept of a nested function and non-local variable.
A nested function is defined inside another function and can access variables of the enclosing scope.
Such non-local variables can only be accessed within their scope and not outside their scope in Python.
The following example shows a nested function accessing a non-local variable.
def outerFunc(txt): # outer enclosing function
def innerFunc(): # nested function
print(txt)
innerFunc()
outerFunc("Hello World")
Output:
Hello World
As you can see, the nested function innerFunc
has accessed the non-local variable txt
of the enclosing function outerFunc
.
A closure is a function object that can remember values in enclosing scopes even if they go out of scope.
def outerFunc(txt): # outer enclosing function
def innerFunc(): # nested function
print(txt)
return innerFunc
myFunc = outerFunc("Hello World")
myFunc()
Output:
Hello World
In this example, the nested function returns the innerfunc()
function instead of calling it. The outerFunc()
function is called with the text Hello World
and assigned to a variable myFunc
.
And when calling myFunc()
, the value was remembered outside the scope.
If you remove the original function, the value in the enclosing scope will still be remembered.
del outerFunc
myFunc()
Output:
Hello World
The following is a simple demonstration of using lambda function and closure in Python.
def sum(x):
def total():
def calc(x):
return x + 5
print(calc(x))
return total
myFunc = sum(5)
myFunc()
Output:
10
Now you should know how to use lambda functions and closures in Python. We hope you find this tutorial helpful.