How to Find Maximum and Minimum Value Using Lambda Expression in Python
- Understanding Lambda Expressions in Python
- Finding Maximum Value in a List Using Lambda
- Finding Minimum Value in a List Using Lambda
- Conclusion
- FAQ
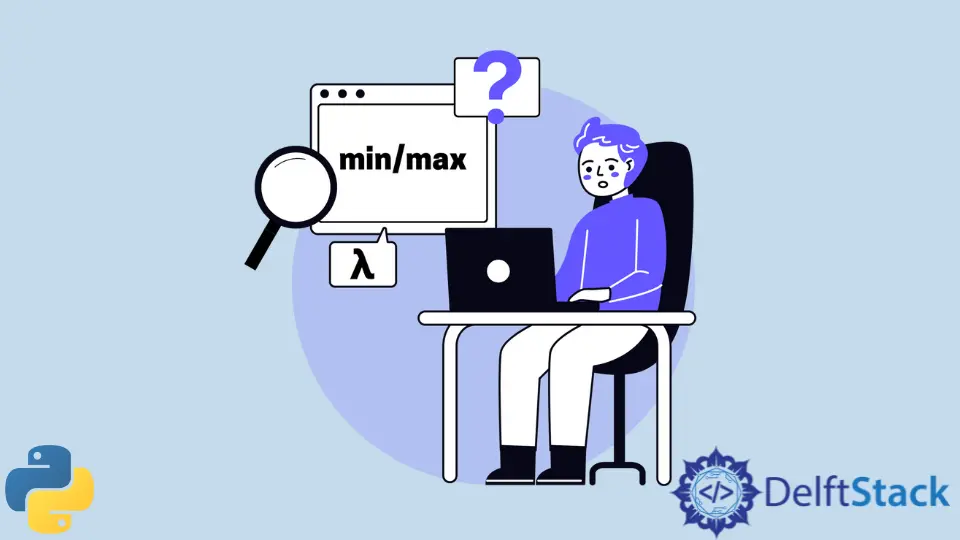
Finding maximum and minimum values in a dataset is a common task in programming, especially when dealing with lists. In Python, lambda expressions provide a concise way to perform this task, allowing you to write cleaner and more efficient code.
This tutorial will guide you through the process of using lambda expressions to find maximum and minimum values in a list. By the end of this article, you will understand how to leverage the power of lambda functions combined with the key property to extract these values effectively. Whether you’re a beginner or looking to refine your skills, this guide will be invaluable.
Understanding Lambda Expressions in Python
Lambda expressions, often referred to as anonymous functions, are a key feature in Python that allows you to create small, one-time-use functions without formally defining them using the def
keyword. The syntax is simple: you use the keyword lambda
followed by parameters, a colon, and an expression.
For example, a basic lambda function that adds two numbers looks like this:
add = lambda x, y: x + y
You can call this function just like a regular function:
result = add(3, 5)
Output:
8
Lambda functions are particularly useful when you want to pass a simple function as an argument to higher-order functions like map()
, filter()
, and reduce()
. They are also commonly used with the key
parameter in functions like max()
and min()
, which is crucial for this tutorial.
Finding Maximum Value in a List Using Lambda
To find the maximum value in a list, you can use the max()
function in combination with a lambda expression. The max()
function takes an iterable and an optional key
parameter, which allows you to specify a custom function to determine the maximum value based on specific criteria.
Here’s an example of how to find the maximum value in a list of dictionaries based on a specific key:
data = [{'name': 'Alice', 'score': 85}, {'name': 'Bob', 'score': 92}, {'name': 'Charlie', 'score': 88}]
max_value = max(data, key=lambda x: x['score'])
Output:
{'name': 'Bob', 'score': 92}
In this example, we have a list of dictionaries where each dictionary contains a name and a score. The max()
function iterates through the list and uses the lambda function to extract the score from each dictionary. The result is the dictionary with the highest score, which in this case belongs to Bob.
Using lambda expressions in this way allows for flexibility and clarity, especially when dealing with complex data structures. You can easily modify the lambda function to accommodate different keys or criteria, making this approach highly adaptable.
Finding Minimum Value in a List Using Lambda
Just as you can find the maximum value, you can also find the minimum value using the min()
function along with a lambda expression. The process is similar to finding the maximum value, and it can be applied to various data structures.
Here’s an example of how to find the minimum value in the same list of dictionaries based on the score:
min_value = min(data, key=lambda x: x['score'])
Output:
{'name': 'Alice', 'score': 85}
In this case, the min()
function scans through the list of dictionaries and applies the lambda function to retrieve the score. The result is the dictionary with the lowest score, which is Alice’s in this example.
Using lambda expressions with the min()
function is particularly useful when you need to quickly determine the lowest value based on specific criteria. It allows you to write concise and readable code while still achieving powerful results. This method is applicable to any iterable, making it a versatile tool in your programming toolkit.
Conclusion
In summary, using lambda expressions in Python to find maximum and minimum values is a straightforward yet powerful technique. By leveraging the max()
and min()
functions along with the key
parameter, you can efficiently extract values based on custom criteria in various data structures. This approach not only enhances the readability of your code but also allows for greater flexibility when working with complex datasets. Whether you are handling lists of dictionaries or other iterable types, mastering lambda expressions will undoubtedly enhance your programming skills.
FAQ
-
What is a lambda expression in Python?
A lambda expression is an anonymous function defined with thelambda
keyword, allowing you to create small, one-time-use functions. -
How do I find the maximum value in a list of numbers using lambda?
You can use themax()
function with a lambda expression as the key to determine the maximum value based on specific criteria. -
Can I use lambda expressions with other functions besides max and min?
Yes, lambda expressions can be used with a variety of functions, includingfilter()
,map()
, andreduce()
.
-
What are the benefits of using lambda expressions?
Lambda expressions allow for more concise and readable code, especially when you need to create simple functions for short-term use. -
Are there any limitations to using lambda expressions?
Lambda expressions are limited to a single expression and cannot contain statements or annotations, which may restrict their use in more complex scenarios.
Abdul is a software engineer with an architect background and a passion for full-stack web development with eight years of professional experience in analysis, design, development, implementation, performance tuning, and implementation of business applications.
LinkedIn