How to Iterate Backwards in Python
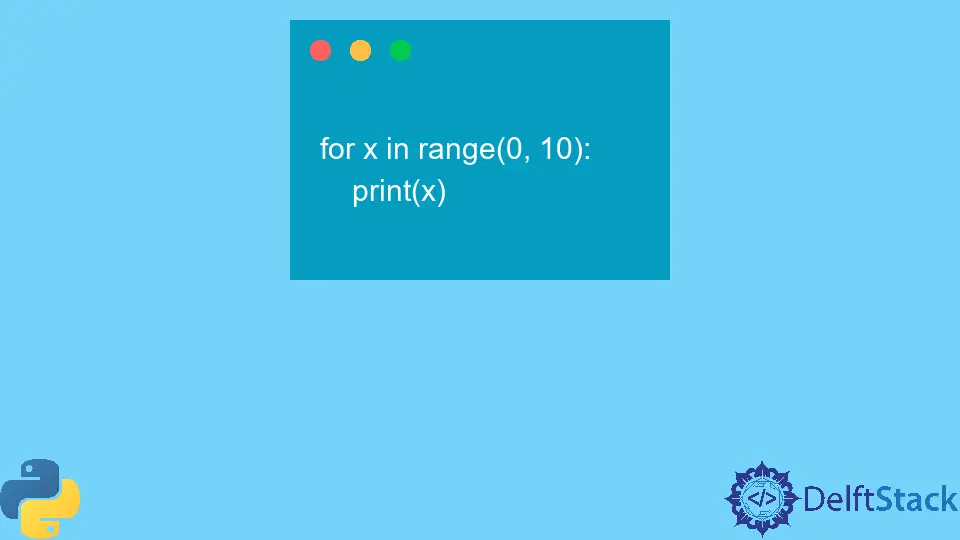
The range()
function in the for
loop can also be used to iterate backward by just setting the third parameter to -1 in Python. In this tutorial, we discuss this function further so that you can execute this program too.
Iterate Backwards With the range()
Function in Python
The traditional use of the range()
function with for
loop is demonstrated in the following coding example:
for x in range(0, 10):
print(x)
Output:
0
1
2
3
4
5
6
7
8
9
This usage of the range()
function gives us values that start with 0 and end with 9 with increments of 1 each time. But what if we want to start with 10 and end with 1 with decrements of 1 each time. Luckily for us, the range()
function already has a solution to this problem.
The range()
function has a third parameter determining the iterative change in the first value until it reaches the second value. This third parameter is known as a step. We can specify -1 in the step parameter if we want to decrement the first parameter by 1 in each iteration. You can use the following program snippet to iterate backward using the step parameter in the range()
function:
for x in range(10, 0, -1):
print(x)
Output:
10
9
8
7
6
5
4
3
2
1
We iterated through 10 to 1 while decrementing by 1 each time with the range()
function in the code above. This approach is so easy to use and straightforward that there is no need for another approach to achieve this goal.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn