Python Iterable
-
Use the
Iteration
in Python -
Use the
Iterable
to Run a Loop in Python -
Use the
Iterator
to Store the State of an Iteration in Python -
the
Built-in Iterator
in Python -
the
Custom Iterator
in Python
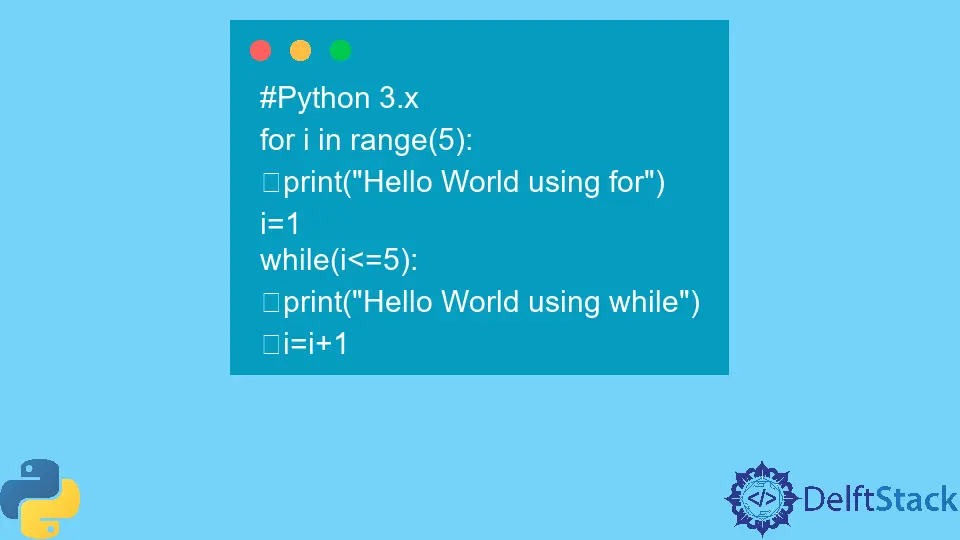
The Iteration
means repeatedly executing a group of statements until the condition is true
. Whenever we use a loop, we are performing iterations.
In python, we can perform iterations using a for
or while
loop.
Use the Iteration
in Python
We will perform five iterations using both the for
and while
loops because they will execute five times.
# Python 3.x
for i in range(5):
print("Hello World using for")
i = 1
while i <= 5:
print("Hello World using while")
i = i + 1
Output:
#Python 3.x
Hello World using for
Hello World using for
Hello World using for
Hello World using for
Hello World using for
Hello World using while
Hello World using while
Hello World using while
Hello World using while
Hello World using while
Use the Iterable
to Run a Loop in Python
An iterable
is an object in python to run a loop. Anything on the right side in the for
loop is iterable.
For example, string
, tuple
, dictionary
, sets
, etc. A List
is iterable
on which we have run a for
loop.
# Python 3.x
colors = {"Blue", "Pink", "Orange", "Yellow"}
for c in colors:
print(c)
Output:
#Python 3.x
Yellow
Orange
Pink
Blue
Use the Iterator
to Store the State of an Iteration in Python
An Iterator
is an object which performs the iteration. Iterator stores the state of an iteration, and it returns the next value to loop on.
An iterable
object is initialized through the __iter__()
method, returning the object. The __next__()
method returns the next value associated with the object to iterate upon.
the Built-in Iterator
in Python
The __iter__()
and __next__()
method explicitly are built-in iterators
. We will call the built-in iter()
method and pass it to our object to make it iterable.
Then we will iterate
over the next elements of an object through the built-in next()
method. The while
loop will break we have completed the iterations upon all the elements associated with the object.
# Python 3.x
mystring = "Orange"
iterable = iter(mystring)
while True:
try:
char = next(iterable)
print(char)
except StopIteration:
break
Output:
#Python 3.x
O
r
a
n
g
e
the Custom Iterator
in Python
The __iter__()
and __next__()
method in our custom class to make it iterable. The __iter__()
method will create and return an iterable object.
The __next__()
method will return the next element associated with its object. The __iter__()
method will be called when the iteration is initialized, and the __next__()
method will be called upon the next iteration to iterate over the next value.
Through a custom
iterator, we will handle the iterations independently.
# Python 3.x
class MyIterator:
def __init__(self, limit):
self.limit = limit
def __iter__(self):
self.n = 1
return self
def __next__(self):
n = self.n
if n > self.limit:
raise StopIteration
self.n = n + 1
return n
for i in MyIterator(10):
print(i)
Output:
#Python 3.x
1
2
3
4
5
6
7
8
9
10
In the __iter__()
method, we had initialized and returned the value of n
, a class attribute
. In the __next__()
method, we first saved the current value of n
.
Then we had to check if the next value is greater than the range defined in iteration, we would raise a StopIteration
exception. Else, we will increment the value of n
and return it.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn