Python 可迭代对象
-
在 Python 中使用
迭代
-
在 Python 中使用
Iterable
运行循环 -
在 Python 中使用
Iterator
存储迭代的状态 -
Python 中的
内置迭代器
-
Python 中的
自定义迭代器
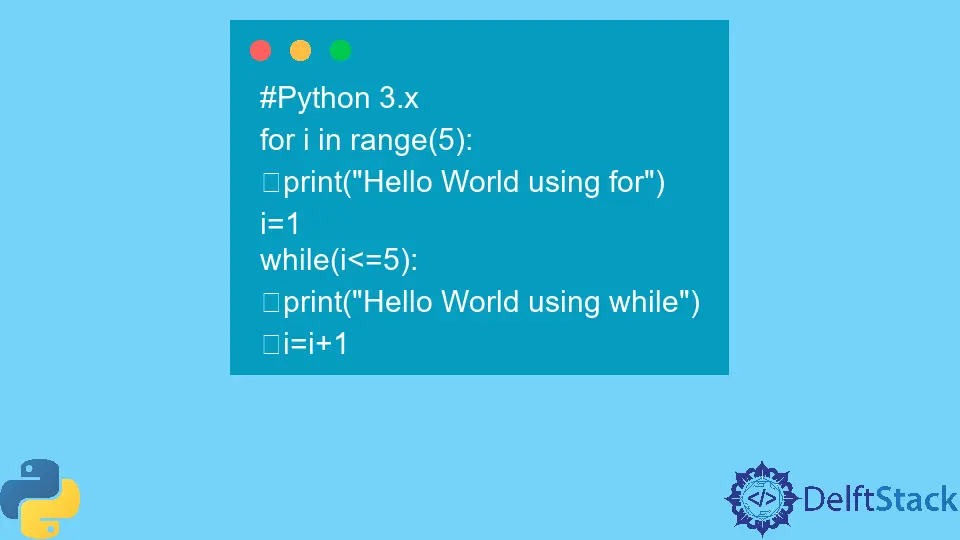
Iteration
意味着重复执行一组语句,直到条件为 true
。每当我们使用循环时,我们都在执行迭代。
在 python 中,我们可以使用 for
或 while
循环来执行迭代。
在 Python 中使用迭代
我们将使用 for
和 while
循环执行五次迭代,因为它们将执行五次。
# Python 3.x
for i in range(5):
print("Hello World using for")
i = 1
while i <= 5:
print("Hello World using while")
i = i + 1
输出:
#Python 3.x
Hello World using for
Hello World using for
Hello World using for
Hello World using for
Hello World using for
Hello World using while
Hello World using while
Hello World using while
Hello World using while
Hello World using while
在 Python 中使用 Iterable
运行循环
iterable
是 Python 中用于运行循环的对象。for
循环右侧的任何内容都是可迭代的。
例如,string
、tuple
、dictionary
、sets
等。List
是 iterable
,我们在其上运行 for
循环。
# Python 3.x
colors = {"Blue", "Pink", "Orange", "Yellow"}
for c in colors:
print(c)
输出:
#Python 3.x
Yellow
Orange
Pink
Blue
在 Python 中使用 Iterator
存储迭代的状态
Iterator
是执行迭代的对象。Iterator 存储迭代的状态,并返回要循环的下一个值。
iterable
对象通过 __iter__()
方法初始化,返回该对象。__next__()
方法返回与要迭代的对象关联的下一个值。
Python 中的内置迭代器
__iter__()
和 __next__()
方法明确地是内置迭代器
。我们将调用内置的 iter()
方法并将其传递给我们的对象以使其可迭代。
然后我们将通过内置的 next()
方法迭代
对象的下一个元素。while
循环将中断我们已完成对与对象关联的所有元素的迭代。
# Python 3.x
mystring = "Orange"
iterable = iter(mystring)
while True:
try:
char = next(iterable)
print(char)
except StopIteration:
break
输出:
#Python 3.x
O
r
a
n
g
e
Python 中的自定义迭代器
我们自定义类中的 __iter__()
和 __next__()
方法使其可迭代。__iter__()
方法将创建并返回一个可迭代对象。
__next__()
方法将返回与其对象关联的下一个元素。__iter__()
方法将在迭代初始化时调用,而 __next__()
方法将在下一次迭代时调用以迭代下一个值。
通过 custom
迭代器,我们将独立处理迭代。
# Python 3.x
class MyIterator:
def __init__(self, limit):
self.limit = limit
def __iter__(self):
self.n = 1
return self
def __next__(self):
n = self.n
if n > self.limit:
raise StopIteration
self.n = n + 1
return n
for i in MyIterator(10):
print(i)
输出:
#Python 3.x
1
2
3
4
5
6
7
8
9
10
在 __iter__()
方法中,我们已经初始化并返回了 n
的值,一个类属性
。在 __next__()
方法中,我们首先保存了 n
的当前值。
然后我们必须检查下一个值是否大于迭代中定义的范围,我们将引发 StopIteration
异常。否则,我们将增加 n
的值并返回它。
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn