Python 可迭代物件
-
在 Python 中使用
迭代
-
在 Python 中使用
Iterable
執行迴圈 -
在 Python 中使用
Iterator
儲存迭代的狀態 -
Python 中的
內建迭代器
-
Python 中的
自定義迭代器
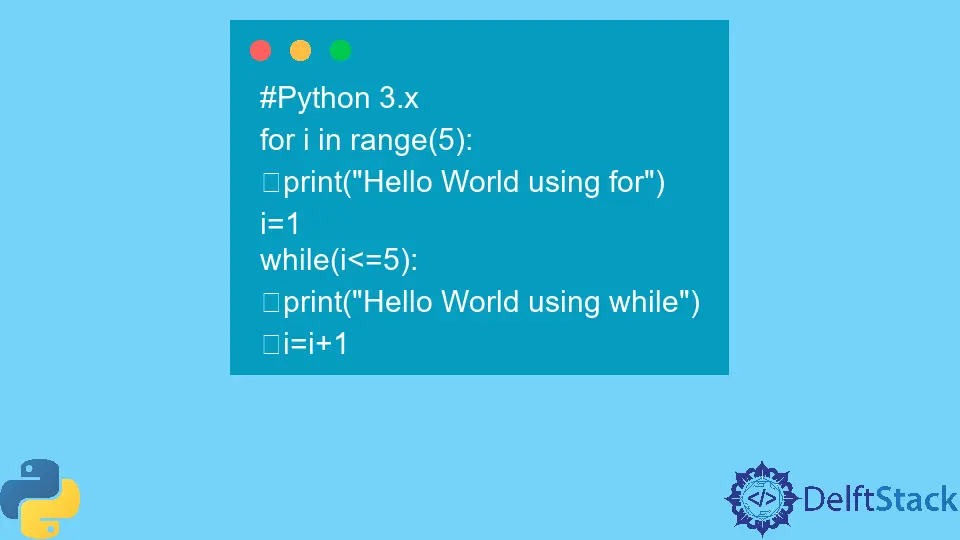
Iteration
意味著重複執行一組語句,直到條件為 true
。每當我們使用迴圈時,我們都在執行迭代。
在 python 中,我們可以使用 for
或 while
迴圈來執行迭代。
在 Python 中使用迭代
我們將使用 for
和 while
迴圈執行五次迭代,因為它們將執行五次。
# Python 3.x
for i in range(5):
print("Hello World using for")
i = 1
while i <= 5:
print("Hello World using while")
i = i + 1
輸出:
#Python 3.x
Hello World using for
Hello World using for
Hello World using for
Hello World using for
Hello World using for
Hello World using while
Hello World using while
Hello World using while
Hello World using while
Hello World using while
在 Python 中使用 Iterable
執行迴圈
iterable
是 Python 中用於執行迴圈的物件。for
迴圈右側的任何內容都是可迭代的。
例如,string
、tuple
、dictionary
、sets
等。List
是 iterable
,我們在其上執行 for
迴圈。
# Python 3.x
colors = {"Blue", "Pink", "Orange", "Yellow"}
for c in colors:
print(c)
輸出:
#Python 3.x
Yellow
Orange
Pink
Blue
在 Python 中使用 Iterator
儲存迭代的狀態
Iterator
是執行迭代的物件。Iterator 儲存迭代的狀態,並返回要迴圈的下一個值。
iterable
物件通過 __iter__()
方法初始化,返回該物件。__next__()
方法返回與要迭代的物件關聯的下一個值。
Python 中的內建迭代器
__iter__()
和 __next__()
方法明確地是內建迭代器
。我們將呼叫內建的 iter()
方法並將其傳遞給我們的物件以使其可迭代。
然後我們將通過內建的 next()
方法迭代
物件的下一個元素。while
迴圈將中斷我們已完成對與物件關聯的所有元素的迭代。
# Python 3.x
mystring = "Orange"
iterable = iter(mystring)
while True:
try:
char = next(iterable)
print(char)
except StopIteration:
break
輸出:
#Python 3.x
O
r
a
n
g
e
Python 中的自定義迭代器
我們自定義類中的 __iter__()
和 __next__()
方法使其可迭代。__iter__()
方法將建立並返回一個可迭代物件。
__next__()
方法將返回與其物件關聯的下一個元素。__iter__()
方法將在迭代初始化時呼叫,而 __next__()
方法將在下一次迭代時呼叫以迭代下一個值。
通過 custom
迭代器,我們將獨立處理迭代。
# Python 3.x
class MyIterator:
def __init__(self, limit):
self.limit = limit
def __iter__(self):
self.n = 1
return self
def __next__(self):
n = self.n
if n > self.limit:
raise StopIteration
self.n = n + 1
return n
for i in MyIterator(10):
print(i)
輸出:
#Python 3.x
1
2
3
4
5
6
7
8
9
10
在 __iter__()
方法中,我們已經初始化並返回了 n
的值,一個類屬性
。在 __next__()
方法中,我們首先儲存了 n
的當前值。
然後我們必須檢查下一個值是否大於迭代中定義的範圍,我們將引發 StopIteration
異常。否則,我們將增加 n
的值並返回它。
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn