How to Reverse a Dictionary in Python
-
Use
items()
to Reverse a Dictionary in Python -
Use
collections.defaultdict()
to Reverse a Dictionary in Python
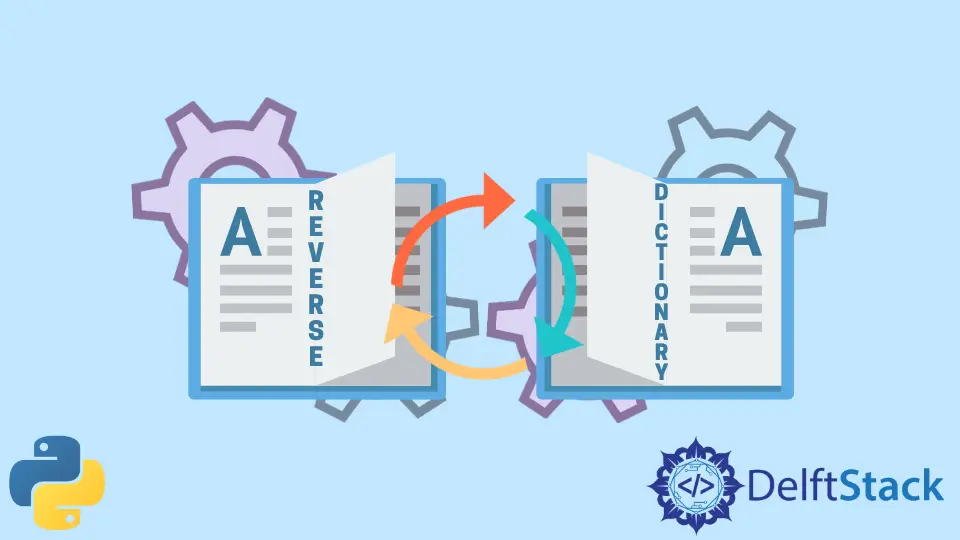
This article demonstrates different methods to invert a dictionary in Python.
Reversing a dictionary is different from reversing a list, it means to invert or switch the key and value elements of the dictionary, essentially swapping them for whatever purpose the developer might use it for.
Use items()
to Reverse a Dictionary in Python
Python dictionaries have a built-in function called items()
which returns an iterable object that will display the dictionaries’ key-value pairs in the form of a tuple.
For example, declare a dictionary of random categories and print it out using items()
.
dct = {
"Animal": "Dog",
"Fruit": "Pear",
"Vegetable": "Cabbage",
"Tree": "Maple",
"Flower": "Daisy",
}
print(dct.items())
Output:
dict_items([('Animal', 'Dog'), ('Fruit', 'Pear'), ('Vegetable', 'Cabbage'), ('Tree', 'Maple'), ('Flower', 'Daisy')])
Printing a dictionary using items()
will output an iterable list of tuple values that we can manipulate using the for
loop for dictionaries.
Reverse the key-value pairs by looping the result of items()
and switching the key and the value.
dct = {v: k for k, v in dct.items()}
print(dct)
The k
and v
in the for
loop stands for key and value respectively. v: k
are the placeholders for the key and value in each iteration, although we set the previous value v
as the new key and the previous key k
as the new value.
Output:
{'Dog': 'Animal', 'Pear': 'Fruit', 'Cabbage': 'Vegetable', 'Maple': 'Tree', 'Daisy': 'Flower'}
The key and the value elements are now reversed in the output.
Same Valued Keys in a Reversed Dictionary
Another example scenario when inverting a dictionary is if there are multiple keys or values with the same value. This is a more likely scenario to invert a dictionary.
For example, a dictionary of people’s favorite animals.
favAnimal = {
"John": "Dog",
"Jane": "Cat",
"Jerome": "Lion",
"Jenny": "Dog",
"Jared": "Giraffe",
"James": "Dog",
}
If the dictionary is inverted using the above code, the output would not be as you’d expect.
favAnimal = {v: k for k, v in favAnimal.items()}
print(favAnimal)
Output:
{'Dog': 'James', 'Cat': 'Jane', 'Lion': 'Jerome', 'Giraffe': 'Jared'}
Here, 2 elements from the original dictionary are missing because every time a key or a value repeats in the loop, the existing record will be overwritten.
The dictionary contains 3 people with the value pair Dog
but only James
was copied because this was the last record with the value of Dog
and has overwritten the 2 other dictionary items.
To solve this problem, we would have to store the values in a list so they would be assigned to a single key.
The complete example code:
favAnimal = {
"John": "Dog",
"Jane": "Cat",
"Jerome": "Lion",
"Jenny": "Dog",
"Jared": "Giraffe",
"James": "Dog",
}
favAnimal = {v: k for k, v in favAnimal.items()}
print(favAnimal)
Use collections.defaultdict()
to Reverse a Dictionary in Python
The module collections
has a function defaultdict()
which can manipulate the values in a dictionary in Python.
defaultdict()
mainly is used for creating default values of non-existent keys. If you access a non-existent key, it can declare a default value for it, even if it doesn’t exist.
This function is useful for our case because we want to instantiate a new dictionary with values that are of a list data type.
Using the same example favAnimal
, invert the dictionary and convert the values to a list.
First, initialize the new dictionary using defaultdict()
from collections import defaultdict
favAnimal_inverted = defaultdict(list)
Next, use the list comprehension for inversion and store the inverted values into the new dictionary.
{favAnimal_inverted[v].append(k) for k, v in favAnimal.items()}
Lastly, since defaultdict()
creates a sort of a subclass of a dictionary, re-convert it to the base dictionary type by wrapping it in dict()
.
result = dict(favAnimal_inverted)
print(result)
Output:
{'Dog': ['John', 'Jenny', 'James'], 'Cat': ['Jane'], 'Lion': ['Jerome'], 'Giraffe': ['Jared']}
John, Jenny, and James now are on the same list with the key Dog
instead of the other elements being overwritten by the last element that had Dog
as their value.
The complete example code:
from collections import defaultdict
favAnimal_inverted = defaultdict(list)
result = dict(favAnimal_inverted)
print(result)
In summary, use items()
to loop over the dictionary and invert the keys and values. If by any chance your data set is likely to have duplicates, then make sure to convert the values into a list by using defaultdict()
and manipulate it in a way that the values of the dictionary will append into the list instead of replacing the existing values.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn