How to Read and Write INI File in Python
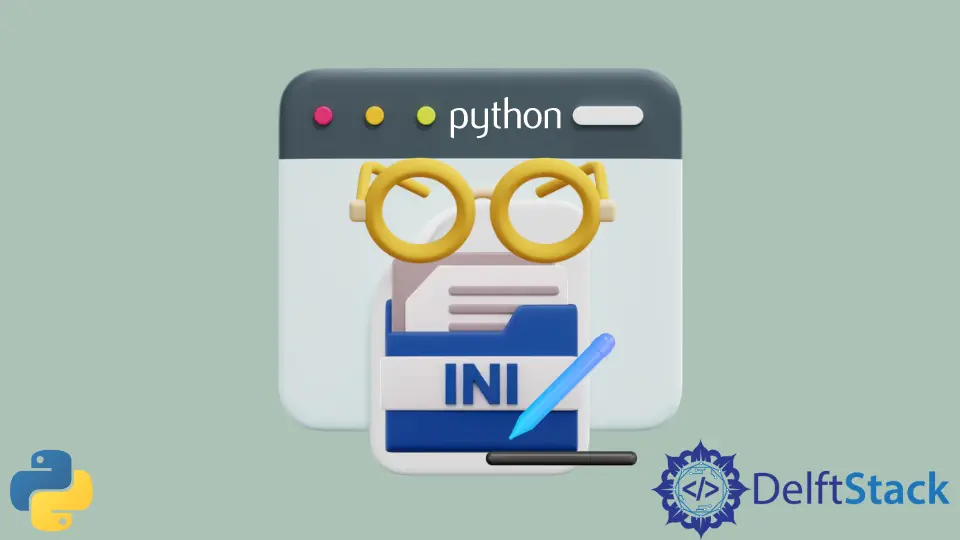
An INI
file is a configuration file for computer software.
It contains key-value pairs that represent properties and their values. These key-value pairs are organized under sections.
An INI
file has .ini
as its extension. An INI
file is used by Microsoft Windows and Microsoft Windows-based applications, and the software uses INI
files to store their configuration.
Python is a robust and versatile programming language that can build desktop applications. Since desktop applications include Microsoft Windows-based applications and software, Python developers should use INI
files to store configurations and read them whenever necessary.
This article will teach how to read and write INI
files using Python.
Read and Write INI
File in Python
To read and write INI
files, we can use the configparser
module. This module is a part of Python’s standard library and is built for managing INI
files found in Microsoft Windows.
This module has a class ConfigParser
containing all the utilities to play around with INI
files. We can use this module for our use case.
Let us understand the usage of this library with the help of a simple example. Refer to the following Python code for this.
import configparser
filename = "file.ini"
# Writing Data
config = configparser.ConfigParser()
config.read(filename)
try:
config.add_section("SETTINGS")
except configparser.DuplicateSectionError:
pass
config.set("SETTINGS", "time", "utc")
config.set("SETTINGS", "time_format", "24h")
config.set("SETTINGS", "language", "english")
config.set("SETTINGS", "testing", "false")
config.set("SETTINGS", "production", "true")
with open(filename, "w") as config_file:
config.write(config_file)
# Reading Data
config.read(filename)
keys = ["time", "time_format", "language", "testing", "production"]
for key in keys:
try:
value = config.get("SETTINGS", key)
print(f"{key}:", value)
except configparser.NoOptionError:
print(f"No option '{key}' in section 'SETTINGS'")
Output:
time: utc
time_format: 24h
language: english
testing: false
production: true
Python Code Explanation
The Python script first creates an object of the ConfigParser
class and reads the INI
file specified by the filename
. There will be no issues if the file does not exist at the specified path.
try:
config.add_section("SETTINGS")
except configparser.DuplicateSectionError:
pass
Next, it tries to create a SETTINGS
section in the INI
file using the add_section()
method.
The add_section()
method throws an configparser.DuplicateSectionError
exception when the section already exists in the INI
file. That is why the function call is surrounded by try
and except
blocks.
config.set("SETTINGS", "time", "utc")
config.set("SETTINGS", "time_format", "24h")
config.set("SETTINGS", "language", "english")
config.set("SETTINGS", "testing", "false")
config.set("SETTINGS", "production", "true")
Next, using the set()
function, we write data to the INI
file.
The set()
function demands three arguments, namely, section
, option
, and value
. This means that a key-value pair will be added under the section
, where the key is the option
, and the value is the value
.
The above Python script adds 5
such pairs.
with open(filename, "w") as config_file:
config.write(config_file)
Next, the INI
file is opened, data is written to it using the write()
method of the configparser
library, and lastly, saved.
config.read(filename)
keys = ["time", "time_format", "language", "testing", "production"]
for key in keys:
try:
value = config.get("SETTINGS", key)
print(f"{key}:", value)
except configparser.NoOptionError:
print(f"No option '{key}' in section 'SETTINGS'")
The Python script again reads the INI
file to read the content of the INI
file, and using the get()
method, it retrieves all the written information. The get()
method accepts two parameters: section
and option
.
It will find the option
under the section
. The get()
method throws a NoOptionError
exception when it fails to find the specified option under the specified section.
So, to avoid any runtime exceptions, the get()
method call and the print()
statement are enclosed inside the try...except
block.