Python Guessing Game
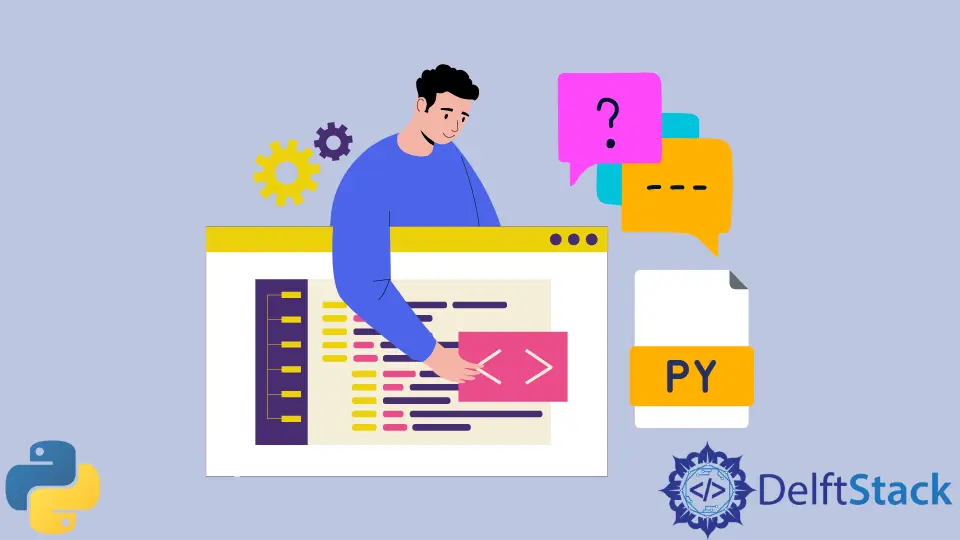
This tutorial will demonstrate the process of developing a simple number guessing game in Python.
Guessing Game Mechanics
We are trying to develop a game that takes the upper and lower limit from the user, generates a random number in that range, asks the user to guess the number, and counts how many trails the user takes to get to the right guess. This game will be CLI-based only.
Number Guessing Game With the random
Module in Python
The first thing our program does is take upper and lower limits from the user as inputs. This can be done with Python’s built-in input()
method.
The input()
method reads the input from the command line and returns it as a string. The only problem here is that we want to input integer values.
We can wrap our input()
method inside the built-in int()
method to solve that problem. This will convert the input string returned by the input()
method into an integer value.
The following example shows a working implementation of this step.
Code:
lower_limit = int(input("Please enter the Lower Limit"))
upper_limit = int(input("Please enter the Upper Limit"))
print("Lower Limit =", lower_limit)
print("Upper Limit =", upper_limit)
Output:
Please enter the Lower Limit0
Please enter the Upper Limit99
Lower Limit = 0
Upper Limit = 99
We can write the message we want to display to the user while inputting the data inside the input()
method as an input parameter. Since we have the lower and upper limits, we can easily write some code to generate a random integer within that range.
We can use the built-in random
module in Python to perform this task called random.randint()
method. It takes the lower limit and upper limit as input parameters and returns an integer within that range.
The next code example shows how to generate a random integer within a specified range using Python’s random.randint()
method.
Code:
import random
number = random.randint(lower_limit, upper_limit)
print("Random Number =", number)
Output:
Random Number = 47
So far, we have taken the limits from the user and generated a random integer within those limits. We have to take the user’s guess and compare it with the randomly generated number.
This can be achieved by combining the input()
method with a simple if/else block.
Code:
guess = int(input("Guess the number"))
if guess == number:
print("Yes, You are correct")
else:
print("Incorrect Answer!")
Output:
Guess the number15
Incorrect Answer!
The only problem here is that it doesn’t give us clues to guess the correct number. It tells us whether we are right or wrong, which isn’t a fun way to play a game.
We can improve that by placing multiple if statements and executing them inside a loop until the user guesses the right number.
Code:
win = False
while win != True:
guess = int(input("Guess the number"))
if guess == number:
win = True
print("Yes, You are correct")
elif guess < number:
print("You are a little shorter")
else:
print("You are a little larger")
Output:
Guess the number5
You are a little shorter
Guess the number95
You are a little larger
Guess the number47
Yes, You are correct
We used a while
loop because we don’t know how many trials the user will take to get the correct answer. We created a flag variable win
that tells the while loop when to stop, and the win
variable is set to False
until the user guesses the right number.
Our number guessing game is almost complete, and the only thing missing from it is the score counter that counts the number of trials a user took while reaching the correct answer. We can modify our previous code and use a counter variable in the loop.
The following code snippet shows how we can add a scoring mechanism to our number guessing game.
Code:
win = False
steps = 0
while win != True:
guess = int(input("Guess the number"))
steps += 1
if guess == number:
win = True
print("Yes, You are correct")
print("Number of Trails =", steps)
elif guess < number:
print("You are a little shorter")
else:
print("You are a little larger")
Output:
Guess the number22
You are a little shorter
Guess the number44
You are a little shorter
Guess the number47
Yes, You are correct
Number of Trails = 3
We added a steps counter that keeps track of the number of trials the user took to finish the game.
Code:
import random
lower_limit = int(input("Please enter the Lower Limit"))
upper_limit = int(input("Please enter the Upper Limit"))
number = random.randint(lower_limit, upper_limit)
win = False
steps = 0
while win != True:
guess = int(input("Guess the number"))
steps += 1
if guess == number:
win = True
print("Yes, You are correct")
print("Number of Trails =", steps)
elif guess < number:
print("You are a little shorter")
else:
print("You are a little larger")
Output:
Please enter the Lower Limit0
Please enter the Upper Limit10
Guess the number5
You are a little larger
Guess the number2
You are a little shorter
Guess the number3
You are a little shorter
Guess the number4
Yes, You are correct
Number of Trails = 4
The output shows that the game only runs for one pass. It doesn’t let the user keep playing the game until they are bored.
We can enclose our whole program inside another loop that executes the game repeatedly until the user wants to exit the game.
Full Code:
import random
play = True
while play == True:
lower_limit = int(input("Please enter the Lower Limit"))
upper_limit = int(input("Please enter the Upper Limit"))
number = random.randint(lower_limit, upper_limit)
win = False
steps = 0
while win != True:
guess = int(input("Guess the number"))
steps += 1
if guess == number:
win = True
print("Yes, You are correct")
print("Number of Trails =", steps)
elif guess < number:
print("You are a little shorter")
else:
print("You are a little larger")
replay = int(input("Enter -1 to replay the game."))
if replay != -1:
play = False
Output:
Please enter the Lower Limit1
Please enter the Upper Limit3
Guess the number2
You are a little larger
Guess the number1
Yes, You are correct
Number of Trails = 2
Enter -1 to replay the game.-1
Please enter the Lower Limit1
Please enter the Upper Limit3
Guess the number2
You are a little larger
Guess the number1
Yes, You are correct
Number of Trails = 2
Enter -1 to replay the game.0
We created another flag variable, play
, telling our outer or main loop when to stop its execution. If the user provides any number other than -1
, the outer loop will stop the execution, assuming that the user has gotten bored of playing this game repeatedly.
It’s a very simple game to implement. We only imported the random
module to generate a random number in our code.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn