Python 推測ゲーム
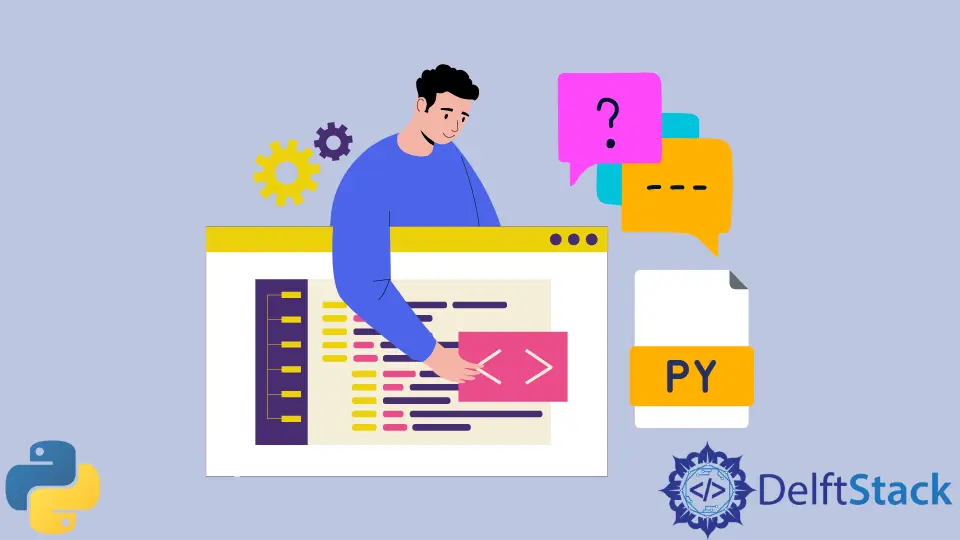
このチュートリアルでは、Python で単純な数字当てゲームを開発するプロセスを紹介します。
ゲームの仕組みを推測する
ユーザーから上限と下限を取得し、その範囲で乱数を生成し、ユーザーに数字を推測してもらい、ユーザーが正しい推測に到達するまでに何回トレイルをたどるかをカウントするゲームを開発しようとしています。 このゲームは CLI ベースのみです。
Python の random
モジュールを使った数当てゲーム
プログラムが最初に行うことは、入力としてユーザーから上限と下限を取得することです。 これは、Python の組み込みの input()
メソッドで実行できます。
input()
メソッドは、コマンド ラインから入力を読み取り、文字列として返します。 ここでの唯一の問題は、整数値を入力したいということです。
input()
メソッドを組み込みの int()
メソッド内にラップして、この問題を解決できます。 これにより、input()
メソッドによって返された入力文字列が整数値に変換されます。
次の例は、このステップの実用的な実装を示しています。
コード:
lower_limit = int(input("Please enter the Lower Limit"))
upper_limit = int(input("Please enter the Upper Limit"))
print("Lower Limit =", lower_limit)
print("Upper Limit =", upper_limit)
出力:
Please enter the Lower Limit0
Please enter the Upper Limit99
Lower Limit = 0
Upper Limit = 99
input()
メソッド内に入力パラメーターとしてデータを入力しながら、表示したいメッセージをユーザーに書き込むことができます。 下限と上限があるので、その範囲内でランダムな整数を生成するコードを簡単に書くことができます。
Python の組み込みの random
モジュールを使用して、random.randint()
メソッドと呼ばれるこのタスクを実行できます。 入力パラメーターとして下限と上限を取り、その範囲内の整数を返します。
次のコード例は、Python の random.randint()
メソッドを使用して、指定された範囲内でランダムな整数を生成する方法を示しています。
コード:
import random
number = random.randint(lower_limit, upper_limit)
print("Random Number =", number)
出力:
Random Number = 47
これまでのところ、ユーザーから制限を取得し、それらの制限内でランダムな整数を生成しました。 ユーザーの推測を取り、それをランダムに生成された数値と比較する必要があります。
これは、input()
メソッドを単純な if/else ブロックと組み合わせることで実現できます。
コード:
guess = int(input("Guess the number"))
if guess == number:
print("Yes, You are correct")
else:
print("Incorrect Answer!")
出力:
Guess the number15
Incorrect Answer!
ここでの唯一の問題は、正しい数を推測する手がかりが得られないことです。 それは私たちが正しいか間違っているかを教えてくれますが、これはゲームをプレイする楽しい方法ではありません.
複数の if ステートメントを配置し、ユーザーが正しい数を推測するまでループ内でそれらを実行することで、これを改善できます。
コード:
win = False
while win != True:
guess = int(input("Guess the number"))
if guess == number:
win = True
print("Yes, You are correct")
elif guess < number:
print("You are a little shorter")
else:
print("You are a little larger")
出力:
Guess the number5
You are a little shorter
Guess the number95
You are a little larger
Guess the number47
Yes, You are correct
ユーザーが正しい答えを得るまでに何回試行するかわからないため、while
ループを使用しました。 while ループにいつ停止するかを伝えるフラグ変数 win
を作成し、ユーザーが正しい数字を推測するまで win
変数を False
に設定します。
私たちの数当てゲームはほぼ完成しています。唯一欠けているのは、正解に到達するまでにユーザーが行った試行回数をカウントするスコア カウンターです。 前のコードを変更して、ループでカウンター変数を使用できます。
次のコード スニペットは、数当てゲームにスコアリング メカニズムを追加する方法を示しています。
コード:
win = False
steps = 0
while win != True:
guess = int(input("Guess the number"))
steps += 1
if guess == number:
win = True
print("Yes, You are correct")
print("Number of Trails =", steps)
elif guess < number:
print("You are a little shorter")
else:
print("You are a little larger")
出力:
Guess the number22
You are a little shorter
Guess the number44
You are a little shorter
Guess the number47
Yes, You are correct
Number of Trails = 3
ユーザーがゲームを完了するまでに行った試行回数を追跡する歩数カウンターを追加しました。
コード:
import random
lower_limit = int(input("Please enter the Lower Limit"))
upper_limit = int(input("Please enter the Upper Limit"))
number = random.randint(lower_limit, upper_limit)
win = False
steps = 0
while win != True:
guess = int(input("Guess the number"))
steps += 1
if guess == number:
win = True
print("Yes, You are correct")
print("Number of Trails =", steps)
elif guess < number:
print("You are a little shorter")
else:
print("You are a little larger")
出力:
Please enter the Lower Limit0
Please enter the Upper Limit10
Guess the number5
You are a little larger
Guess the number2
You are a little shorter
Guess the number3
You are a little shorter
Guess the number4
Yes, You are correct
Number of Trails = 4
出力は、ゲームが 1つのパスに対してのみ実行されることを示しています。 ユーザーが飽きるまでゲームをプレイし続けることはできません。
プログラム全体を、ユーザーがゲームを終了するまで繰り返しゲームを実行する別のループ内に含めることができます。
完全なコード:
import random
play = True
while play == True:
lower_limit = int(input("Please enter the Lower Limit"))
upper_limit = int(input("Please enter the Upper Limit"))
number = random.randint(lower_limit, upper_limit)
win = False
steps = 0
while win != True:
guess = int(input("Guess the number"))
steps += 1
if guess == number:
win = True
print("Yes, You are correct")
print("Number of Trails =", steps)
elif guess < number:
print("You are a little shorter")
else:
print("You are a little larger")
replay = int(input("Enter -1 to replay the game."))
if replay != -1:
play = False
出力:
Please enter the Lower Limit1
Please enter the Upper Limit3
Guess the number2
You are a little larger
Guess the number1
Yes, You are correct
Number of Trails = 2
Enter -1 to replay the game.-1
Please enter the Lower Limit1
Please enter the Upper Limit3
Guess the number2
You are a little larger
Guess the number1
Yes, You are correct
Number of Trails = 2
Enter -1 to replay the game.0
別のフラグ変数 play
を作成し、外側またはメイン ループにいつ実行を停止するかを伝えます。 ユーザーが -1
以外の数値を指定すると、ユーザーがこのゲームを繰り返しプレイすることに飽きたと想定して、外側のループが実行を停止します。
実装するのは非常に簡単なゲームです。 random
モジュールのみをインポートして、コードで乱数を生成しました。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn