파이썬 추측 게임
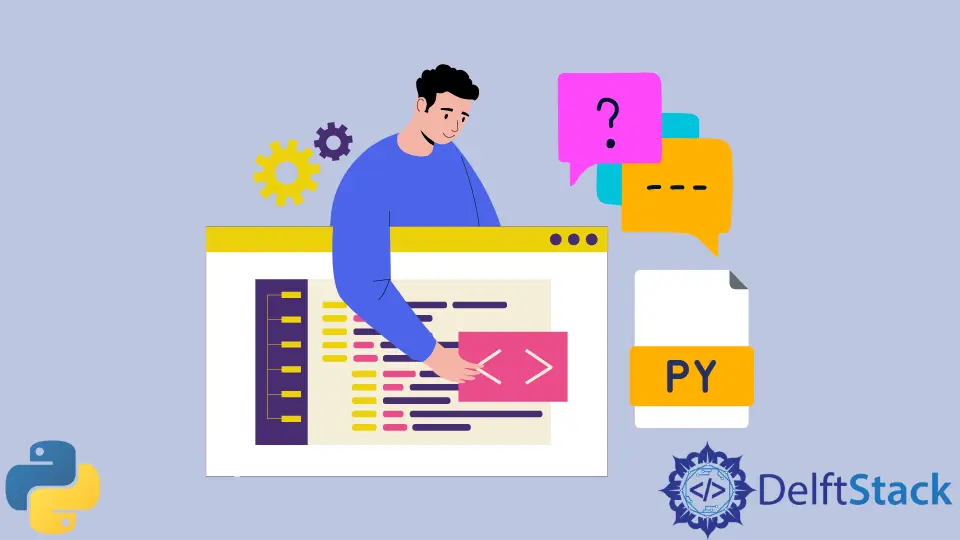
이 튜토리얼은 Python에서 간단한 숫자 추측 게임을 개발하는 과정을 보여줍니다.
추측 게임 역학
우리는 사용자로부터 상한과 하한을 취하고, 그 범위에서 임의의 숫자를 생성하고, 사용자에게 숫자를 추측하도록 요청하고, 사용자가 올바른 추측에 도달하기까지 얼마나 많은 트레일을 거치는지 세는 게임을 개발하려고 합니다. 이 게임은 CLI 기반일 뿐입니다.
Python의 random
모듈을 사용한 숫자 추측 게임
우리 프로그램이 하는 첫 번째 일은 사용자로부터 상한과 하한을 입력으로 받는 것입니다. 이것은 Python의 내장 input()
메서드를 사용하여 수행할 수 있습니다.
input()
메서드는 명령줄에서 입력을 읽고 문자열로 반환합니다. 여기서 유일한 문제는 정수 값을 입력해야 한다는 것입니다.
이 문제를 해결하기 위해 input()
메서드를 내장된 int()
메서드로 래핑할 수 있습니다. 그러면 input()
메서드가 반환한 입력 문자열이 정수 값으로 변환됩니다.
다음 예제는 이 단계의 작업 구현을 보여줍니다.
암호:
lower_limit = int(input("Please enter the Lower Limit"))
upper_limit = int(input("Please enter the Upper Limit"))
print("Lower Limit =", lower_limit)
print("Upper Limit =", upper_limit)
출력:
Please enter the Lower Limit0
Please enter the Upper Limit99
Lower Limit = 0
Upper Limit = 99
input()
메서드 내부에 데이터를 입력 매개변수로 입력하면서 사용자에게 보여주고 싶은 메시지를 작성할 수 있습니다. 하한과 상한이 있으므로 해당 범위 내에서 임의의 정수를 생성하는 코드를 쉽게 작성할 수 있습니다.
Python에 내장된 random
모듈을 사용하여 random.randint()
메서드라는 이 작업을 수행할 수 있습니다. 하한과 상한을 입력 매개변수로 사용하고 해당 범위 내의 정수를 반환합니다.
다음 코드 예제는 Python의 random.randint()
메서드를 사용하여 지정된 범위 내에서 임의의 정수를 생성하는 방법을 보여줍니다.
암호:
import random
number = random.randint(lower_limit, upper_limit)
print("Random Number =", number)
출력:
Random Number = 47
지금까지 우리는 사용자로부터 제한을 받고 해당 제한 내에서 임의의 정수를 생성했습니다. 우리는 사용자의 추측을 받아서 임의로 생성된 숫자와 비교해야 합니다.
이것은 input()
메서드를 간단한 if/else 블록과 결합하여 달성할 수 있습니다.
암호:
guess = int(input("Guess the number"))
if guess == number:
print("Yes, You are correct")
else:
print("Incorrect Answer!")
출력:
Guess the number15
Incorrect Answer!
여기서 유일한 문제는 올바른 숫자를 추측할 수 있는 단서를 제공하지 않는다는 것입니다. 그것은 우리가 옳은지 그른지를 말해줍니다. 이것은 게임을 하는 재미있는 방법이 아닙니다.
여러 if 문을 배치하고 사용자가 올바른 숫자를 추측할 때까지 루프 내에서 실행함으로써 이를 개선할 수 있습니다.
암호:
win = False
while win != True:
guess = int(input("Guess the number"))
if guess == number:
win = True
print("Yes, You are correct")
elif guess < number:
print("You are a little shorter")
else:
print("You are a little larger")
출력:
Guess the number5
You are a little shorter
Guess the number95
You are a little larger
Guess the number47
Yes, You are correct
우리는 사용자가 정답을 얻기 위해 얼마나 많은 시행착오를 겪을지 모르기 때문에 while
루프를 사용했습니다. 우리는 while 루프에 언제 중지할지 알려주는 플래그 변수 win
을 만들었고 win
변수는 사용자가 올바른 숫자를 추측할 때까지 False
로 설정됩니다.
우리의 숫자 추측 게임은 거의 완성되었으며, 여기서 빠진 유일한 것은 정답에 도달하는 동안 사용자가 시도한 횟수를 세는 점수 카운터입니다. 이전 코드를 수정하고 루프에서 카운터 변수를 사용할 수 있습니다.
다음 코드 스니펫은 숫자 추측 게임에 채점 메커니즘을 추가하는 방법을 보여줍니다.
암호:
win = False
steps = 0
while win != True:
guess = int(input("Guess the number"))
steps += 1
if guess == number:
win = True
print("Yes, You are correct")
print("Number of Trails =", steps)
elif guess < number:
print("You are a little shorter")
else:
print("You are a little larger")
출력:
Guess the number22
You are a little shorter
Guess the number44
You are a little shorter
Guess the number47
Yes, You are correct
Number of Trails = 3
사용자가 게임을 완료하기 위해 시도한 횟수를 추적하는 걸음 수 카운터를 추가했습니다.
암호:
import random
lower_limit = int(input("Please enter the Lower Limit"))
upper_limit = int(input("Please enter the Upper Limit"))
number = random.randint(lower_limit, upper_limit)
win = False
steps = 0
while win != True:
guess = int(input("Guess the number"))
steps += 1
if guess == number:
win = True
print("Yes, You are correct")
print("Number of Trails =", steps)
elif guess < number:
print("You are a little shorter")
else:
print("You are a little larger")
출력:
Please enter the Lower Limit0
Please enter the Upper Limit10
Guess the number5
You are a little larger
Guess the number2
You are a little shorter
Guess the number3
You are a little shorter
Guess the number4
Yes, You are correct
Number of Trails = 4
출력은 게임이 한 패스에 대해서만 실행됨을 보여줍니다. 사용자가 지루할 때까지 게임을 계속할 수 없습니다.
사용자가 게임을 종료하기를 원할 때까지 게임을 반복적으로 실행하는 또 다른 루프 안에 전체 프로그램을 묶을 수 있습니다.
전체 코드:
import random
play = True
while play == True:
lower_limit = int(input("Please enter the Lower Limit"))
upper_limit = int(input("Please enter the Upper Limit"))
number = random.randint(lower_limit, upper_limit)
win = False
steps = 0
while win != True:
guess = int(input("Guess the number"))
steps += 1
if guess == number:
win = True
print("Yes, You are correct")
print("Number of Trails =", steps)
elif guess < number:
print("You are a little shorter")
else:
print("You are a little larger")
replay = int(input("Enter -1 to replay the game."))
if replay != -1:
play = False
출력:
Please enter the Lower Limit1
Please enter the Upper Limit3
Guess the number2
You are a little larger
Guess the number1
Yes, You are correct
Number of Trails = 2
Enter -1 to replay the game.-1
Please enter the Lower Limit1
Please enter the Upper Limit3
Guess the number2
You are a little larger
Guess the number1
Yes, You are correct
Number of Trails = 2
Enter -1 to replay the game.0
우리는 또 다른 플래그 변수 play
를 생성하여 외부 또는 메인 루프의 실행 중지 시점을 알려줍니다. 사용자가 -1
이외의 숫자를 제공하면 사용자가 이 게임을 반복적으로 플레이하는 것이 지루하다고 가정하여 외부 루프가 실행을 중지합니다.
구현하기 매우 간단한 게임입니다. 코드에서 난수를 생성하기 위해 random
모듈만 가져왔습니다.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn