How to Create Getter and Setter in Python
- Getter and Setter in Python
- Use a Function to Create Getter and Setter in Python
-
Use the
property()
Function to Create Getter and Setter in Python -
Use the
@property
Decorator to Create Getter and Setter in Python
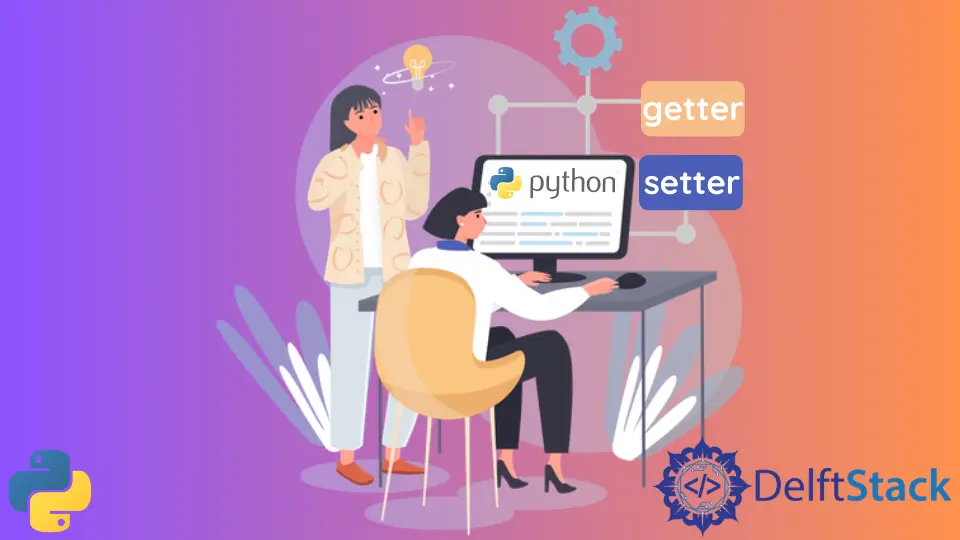
Object-Oriented Programming (OOP), a programming paradigm, makes a lot of things easily, from composability to inheritance and allows us to build features and program parts faster. This paradigm has different features; two are getters
and setters
.
Classes, the foundation of OOP, often have variables that are unique to each instance, and these variables (often called properties) are set or obtained via methods. These methods are called getters
and setters
.
These behaviors are popular in programming languages that support OOP, and Python supports it too. This article will discuss how to create a getter
and setter
in Python.
Getter and Setter in Python
Getters and Setters are methods that help us set class variables or properties without direct access, defeating the purpose of abstraction and encapsulation. So, with getters
and setters
, we are afforded the ability to work with class properties.
Before we create getters
and setters
, it is important to know that, unlike other programming languages, Python doesn’t have hidden fields so you will have access to the variables within a class directly via dot notation.
We can achieve getters
and setters
using normal functions, property()
function, and @property
decorators.
Use a Function to Create Getter and Setter in Python
Typical class functions called methods are useful to create getters
and setters
, and we can easily set them up using the self
concept.
The method returns property for the getter
, and for the setters
, the method binds the argument to the property. To showcase, we will use an Employee
which holds a position
property, the getter
method called getPosition
, and the setter
method called setPosition
.
class Employee:
def __init__(self) -> None:
self.position = None
def getPosition(self):
return self.position
def setPosition(self, position):
self.position = position
Jacob = Employee()
Jinku = Employee()
Jacob.setPosition("Engineer II")
Jinku.setPosition("Senior Engineer")
print(Jacob.position)
print(Jinku.getPosition())
Output:
Engineer II
Senior Engineer
But, this setup or approach doesn’t have much special behavior.
Use the property()
Function to Create Getter and Setter in Python
To get some special behavior, we can make use of the property()
function which creates and returns a property object, which holds three methods, getter()
, setter()
, and delete()
.
It helps provides an interface to instance attributes. This functionality allows us to easily create all the getter
and setter
abilities.
To use the property()
function, we need to set four argument values which are functions making property()
, a higher-order function.
The getter
, setter
, and delete
methods are its arguments (which are all optional), and it returns the property object.
property(fget, fset, fdel, doc)
Let’s use the property()
function in our OOP code.
class Employee:
def __init__(self):
self.position = None
def getPosition(self):
return self.position
def setPosition(self, position):
self.position = position
pos = property(getPosition, setPosition)
Jacob = Employee()
Jinku = Employee()
Jacob.position = "Engineer II"
Jinku.position = "Senior Engineer"
print(Jacob.position)
print(Jinku.position)
Output:
Engineer II
Senior Engineer
With the introduction of the property()
function, we have the pos
binding, which holds the property object that helps to keep access to private properties safe.
Use the @property
Decorator to Create Getter and Setter in Python
We can implement the property()
function using the @property
decorator, and with this, there is no need to use the get
and set
names within our method.
With the @property
decorator, we can reuse the position
name that will define the getter
and setter
functions.
With the @property
, we create the getter
, and with the @position.setter
, we create the setter
, and that’s obvious with the print
statement added to the code and visible within the output.
class Employee:
def __init__(self):
self.position = None
@property
def position(self):
print("Get Employee Position: ")
return self._position
@position.setter
def position(self, value):
print("Set Position")
self._position = value
Jacob = Employee()
Jinku = Employee()
Jacob.position = "Engineer II"
Jinku.position = "Senior Engineer"
print(Jacob.position)
print(Jinku.position)
Output:
Set Position
Set Position
Set Position
Set Position
Get Employee Position:
Engineer II
Get Employee Position:
Senior Engineer
There are four Set Position
because of the __init__
method, which sets the initial position
property when the class instance is called, which leads to the first two. When we set the value to the respective ones for the next two, it prints again.
For the getter
, it prints the statement Get Employee Position
when it gets property value, and the getter
is only called twice. Hence, the two statements.
We can add validation to our OOP code when we use the setter
method.
class Employee:
def __init__(self):
self.position = None
@property
def position(self):
print("Get Employee Position: ")
return self._position
@position.setter
def position(self, value):
print("Set Position")
if value != None and len(value) <= 2:
raise ValueError("Position name is less than two and is deemed invalid")
self._position = value
Jacob = Employee()
Jinku = Employee()
Jacob.position = "OS"
Jinku.position = "Senior Engineer"
print(Jacob.position)
print(Jinku.position)
Output:
Set Position
Set Position
Set Position
Traceback (most recent call last):
File "c:\Users\akinl\Documents\Python\getterSetter.py", line 20, in <module>
Jacob.position = "OS"
File "c:\Users\akinl\Documents\Python\getterSetter.py", line 14, in position
raise ValueError("Position name is less than two and is deemed invalid")
ValueError: Position name is less than two and is deemed invalid
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn