Property Decorator in Python
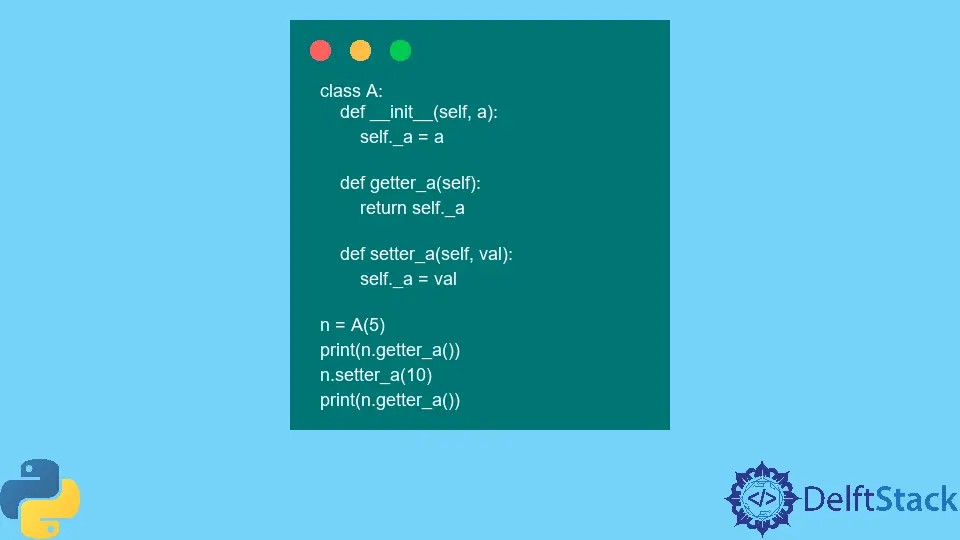
We can create our classes and define different data members and functions in Python. Everything is an object, and we can instantiate objects of our own-defined class.
This tutorial will demonstrate using the Python property decorator (@property
).
Properties in Python
Sometimes, we use Python’s getter and setter functions to return a non-public class attribute.
We create a function within the class that returns this attribute, the getter
function.
The function that can set the value for such an attribute is called the setter
function.
Example:
class A:
def __init__(self, a):
self._a = a
def getter_a(self):
return self._a
def setter_a(self, val):
self._a = val
n = A(5)
print(n.getter_a())
n.setter_a(10)
print(n.getter_a())
Output:
5
10
In the above example, we created the getter and setter functions that return and change the attribute value of a
.
This method is not considered the Pythonic way to deal with attributes. We can remove the need to cluster the class with getter and setter methods.
The Python approach involves using properties that can establish functionalities for a given class attribute.
Using the property()
function, we create objects of the property
class. We attach the getter, setter, and deleter methods as properties for a data member.
For this, we use the fget
, fset
, fdel
, and doc
arguments within the property function.
The function associated with the fget
will return the attribute’s value. Similarly, the fset
method will alter the attribute’s value, and the fdel
function deletes the value.
The doc
method provides the documentation for a given attribute.
We will use these in the code below.
class A:
def __init__(self, a):
self._a = a
def get_a(self):
return self._a
def set_a(self, val):
self._a = val
def del_a(self):
del self._a
print("Deleted")
a = property(fget=get_a, fset=set_a, fdel=del_a, doc="Documenttion for a")
n = A(5)
print(n.a)
n.a = 10
print(n.a)
del n.a
print(n.a)
Output:
5
10
Deleted
Traceback (most recent call last):
File "<string>", line 21, in <module>
File "<string>", line 6, in get_a
AttributeError: 'A' object has no attribute '_a'
In the above example, we created the property a
for the class and added the necessary properties. As you can observe, after using the deleted
property, we get the AttributeError
which indicates that the attribute has been removed.
Use the @property
Decorator in Python
Decorators are used in Python to add additional functionality to a function. A function is taken as an argument, and another function is returned.
After introducing decorators in Python, the use of the property()
function to set the properties died down, and the decorator syntax for the same was preferred.
The decorators were introduced in Python v2.4. The @property
decorator became popular to create properties.
We will use the @property
decorator in the previous example below.
class A:
def __init__(self, a):
self._a = a
@property
def a(self):
return self._a
@a.setter
def a(self, val):
self._a = val
@a.deleter
def a(self):
del self._a
print("Deleted")
n = A(5)
print(n.a)
n.a = 10
print(n.a)
del n.a
print(n.a)
Output:
5
10
Deleted
Traceback (most recent call last):
File "<string>", line 23, in <module>
File "<string>", line 7, in a
AttributeError: 'A' object has no attribute '_a'
As you can observe, we got the same output as we did in the previous example. This method is relatively easy to use than the property()
function.
With the @property
decorator, we use the function that returns the attribute as the property name. After that, the @<property-name>.setter
and @<property-name>.deleter
will add the setter and deleter methods.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn