How to Get Class Name in Python
-
Use the
type()
Function and__name__
to Get the Type or Class of the Object/Instance -
Use the
__class__
and__name__
Properties to Get the Type or Class of an Object/Instance -
Use the
inspect
Module to Get the Type or Class of an Object/Instance - Conclusion
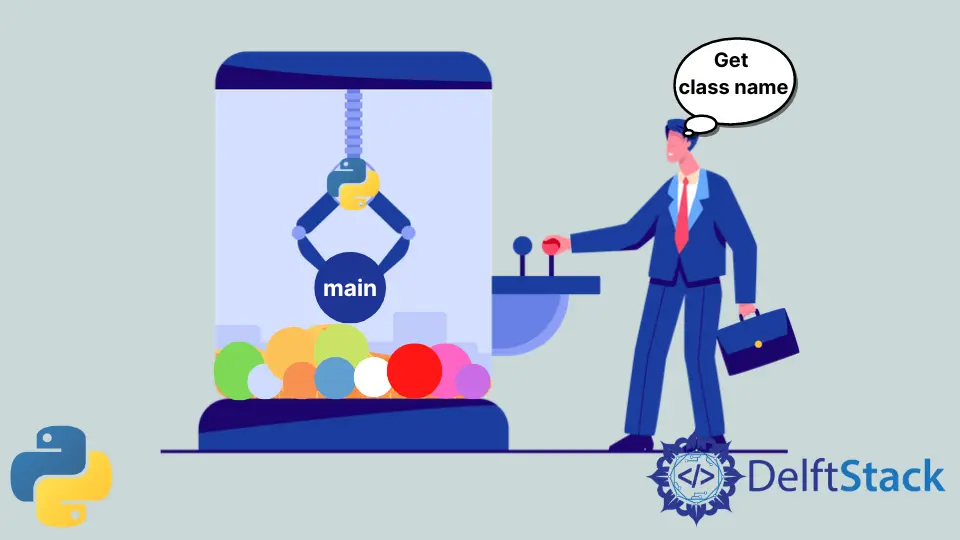
This article delves into three distinct methods of retrieving class names in Python, each offering unique insights and applications. The use of the type()
function combined with the __name__
attribute, the direct access of __class__.__name__
, and the application of the inspect
module represent different facets of Python’s versatile approach to object introspection.
Each method, while serving the same end goal of identifying the class of an object, caters to varying levels of complexity and specificity in programming needs. From simple class identification to deeper introspective analysis, these methods embody the adaptability and depth of Python, catering to a wide spectrum of programming scenarios - from basic scripts to complex, large-scale applications.
Use the type()
Function and __name__
to Get the Type or Class of the Object/Instance
type()
is a predefined function that can be used in finding the type or class of an object.
__name__
is a special built-in variable that basically gives the name of the current module where it is used. Since Python has no main()
function like the other languages like C/C++, Java, and other similar languages, if it is the source file that is executed as the main program, then the interpreter sets the value of __main__
to __name__
.
At the same time, when a file is imported from any other module, __name__
is then set to that module’s name.
The type()
function and the __name__
variable are used to get the type or class of the object in the following code.
Example:
class Animal:
pass
class Dog(Animal):
pass
my_dog = Dog()
# Using type() to get the class name
class_name = type(my_dog).__name__
print(f"The class name is: {class_name}")
In the provided code, we define two classes, Animal
and Dog
, with the latter inheriting from the former. An instance of the Dog
class, named my_dog
, is then created.
The key part of the code lies in the utilization of the type()
function, where we obtain the type of my_dog
. The returned type object is a detailed representation including the class name, and by accessing its __name__
attribute, we extract and store the class name as a string.
In this case, the print
statement confirms that the class name of our instance is Dog
. This concise code analysis highlights the simplicity and effectiveness of using type()
for extracting class names, showcasing its relevance in scenarios where a dynamic understanding of object types is essential.
Output:
Use the __class__
and __name__
Properties to Get the Type or Class of an Object/Instance
The __class__
property can also be used to find the class or type of an object. It basically refers to the class the object was created in.
The __name__
can also be used along with __class__
to get the class of the object.
The following code uses both __class__
and __name__
to get the class of an object.
Example:
class Vehicle:
pass
class Car(Vehicle):
pass
my_car = Car()
# Getting the class name using __class__.__name__
class_name = my_car.__class__.__name__
print(f"The class name is: {class_name}")
In this example, we have two classes: Vehicle
and Car
, with Car
inheriting from Vehicle
. We create an instance of Car
named my_car
.
The focal point of our code is the expression my_car.__class__.__name__
. Here, my_car.__class__
accesses the class Car
, and __name__
then retrieves the name of this class as a string.
The print
statement outputs the class name, confirming the class of our my_car
instance.
Output:
Use the inspect
Module to Get the Type or Class of an Object/Instance
Python’s inspect
module is a part of the standard library, which provides several useful functions to gather information about live objects, including classes. While other methods like __class__.__name__
are more straightforward for getting a class’s name, inspect
offers a more robust and informative approach, especially in complex scenarios.
Example:
import inspect
class Animal:
pass
class Dog(Animal):
pass
my_dog = Dog()
# Using inspect to get the class name
class_info = inspect.getmro(type(my_dog))
class_name = class_info[0].__name__
print(f"The class name is: {class_name}")
In our code, we define two classes, Animal
and Dog
, with Dog
inheriting from Animal
. We create an instance of Dog
named my_dog
.
To find the class name, we first use type(my_dog)
to get the type object of my_dog
, and then pass this to inspect.getmro()
. This function returns a tuple containing the method resolution order, where the first element is the class of my_dog
itself.
By accessing the __name__
attribute of this first element, we successfully retrieve the name of the class. The print statement then confirms the class name of our object.
Output:
Conclusion
Throughout this article, we’ve explored three powerful methods in Python for obtaining the class name of an object: using the type()
function with the __name__
attribute, directly accessing __class__.__name__
, and employing the inspect
module. Each method offers its unique advantages, ranging from straightforward class identification with type()
and __class__.__name__
, to more comprehensive introspection using the inspect
module.
These techniques are not just vital for understanding the type of objects in Python but also form a fundamental aspect of debugging and developing more complex, dynamic Python applications.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn