How to Generate Password in Python
- Generate Password in Python
- Generate Password Using the Random Module in Python
- Generate Password Using the Secrets Module in Python
- Conclusion
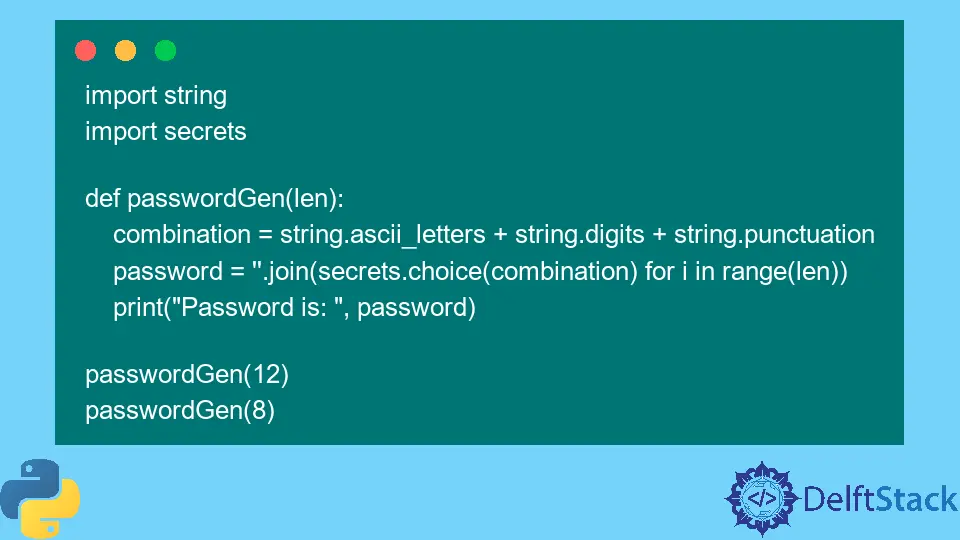
We often hear about data breaches, cyber threats, and cyber attacks on systems; therefore, the security and confidentiality of the data have become a very important part of our lives. Now, one of how the data can be secured is by securing them with passwords.
In this article, we will be discussing password generation in Python.
Generate Password in Python
Passwords often come into the picture as we talk about security. Now, as these passwords are used to secure so much important data, therefore they must be difficult to crack by hackers.
The attackers often try to crack such passwords by randomly trying out different combinations of characters and guessing the right password.
When we humans manually generate passwords, they are often easy to crack. Many try combining their names with digits and symbols that are a cakewalk for hackers to crack.
Therefore, these passwords should be created such that they are not easy to crack. We have some password generation methods in Python which are difficult to crack and easy to make.
Now, let us discuss some of them in detail.
Generate Password Using the Random Module in Python
The random
module in Python generates random elements from a list. Moreover, we will use the choice()
method from the random
module of Python.
The choice()
method chooses the random elements from a list. Therefore, the random.choice()
method will be our main catch in generating a password using this method.
However, now you must be wondering what this list is that we are talking about. So, the list from which the random.choice()
method will choose will contain all the lowercase and uppercase letters, digits, and punctuation symbols.
Therefore, the passwords that will be generated will contain a random order of these characters that would be difficult to crack.
Now, we will use the string
module in Python to get all the lowercase, uppercase letter, digits, and punctuation symbols. The string
module’s ascii.lowercase
method is used to get all the lowercase letters from the English alphabet, and ascii.uppercase
is used to get all the uppercase letters of the English alphabet.
For getting the digits and punctuation symbols, we use the string.digits
and string.punctuation
methods from the string
module in Python, respectively.
Let us see the code to generate a password using the random
and string
modules in Python.
import random
import string
def passwordGen(length):
lower = string.ascii_lowercase
upper = string.ascii_uppercase
digits = string.digits
symbols = string.punctuation
comb = lower + upper + digits + symbols
result_str = "".join(random.choice(comb) for i in range(length))
print("Password of length ", length, "is:", result_str)
passwordGen(12)
passwordGen(10)
passwordGen(5)
Output:
Password of length 12 is: ^)n34W6~0Wdx
Password of length 10 is: g&#'G-T\vn
Password of length 5 is: &g/S3
Therefore, as you can see in the above output, we have three different-length passwords, which are unique and difficult to crack.
However, we have imported the random
and the string
module in the code example. Afterward, we made a function passwordGen
that takes a length as a parameter and prints a password of that specific length.
Inside the function, we have stored all the letters, digits, and symbols in different variables. Now, we have combined all these characters into a single string and stored it in a variable comb
.
Now, we have used random.choice(comb)
to randomly choose characters from the string comb
for each index up to the specified length and then print the results.
Generate Password Using the Secrets Module in Python
One more module in Python is used to generate strong cryptographic random numbers for security purposes. This module is known as the secrets
module; however, this module is available from Python version 3.6 and more.
This secrets
module provides an operating system’s most secure source of randomness; therefore, the passwords generated are more secure and effective. Now let us discuss the program to generate the password using Python’s secrets
module.
The program is similar to the one we have previously discussed but using the secrets
module is more secure and effective in securing our systems.
import string
import secrets
def passwordGen(len):
combination = string.ascii_letters + string.digits + string.punctuation
password = "".join(secrets.choice(combination) for i in range(len))
print("Password is: ", password)
passwordGen(12)
passwordGen(8)
Output:
Password is: Gz!*j3p4xd$Z
Password is: 5#;CSIa7
Therefore, as you can see in the output, we have made a method passwordGen
in which we have a variable combination
that combines all the letters, digits, and punctuation marks for creating a password using the methods of the string
module in Python.
Afterward, we used the secrets.choice(combination)
method and gave it all the characters to choose from to make a password of a given length of len
and then print it. Therefore, we perform password generation in Python using the secrets
module.
Conclusion
In this article, we discussed how to generate passwords in Python. Since security is a major requirement in this era, the generation of safe and secured passwords is very important.
For this purpose, Python has two modules, namely, random
and secrets
, that help generate difficult and not-so-easy-to-crack passwords. However, both modules take help from the string
module to take up a combination of characters to choose from.
However, the secrets
module is implemented from Python version 3.6 but is still considered a more secure and safe way to generate passwords.