Python Function Parameter Type
- Python Function Parameter Types
- What is a Function in Python
- Default and Flexible Arguments in Python
- Conclusion
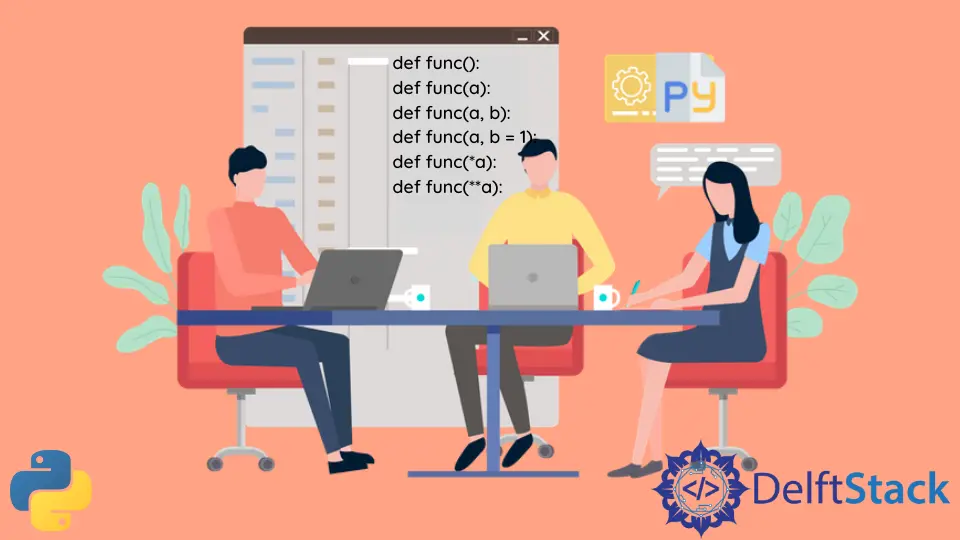
In this Python article, we will learn the function parameter types used in Python. We will also learn how to write a Python function without a parameter.
We will see how we can write a function in Python with one or multiple parameters. We need to understand a function and how to write a function in Python.
Let’s begin by understanding the concepts of parameter types in Python.
Python Function Parameter Types
Python function parameters provide data that the functions use when they are executed. We can specify parameters differently, but not all methods are required in every function.
It depends on the functionalities of the function, and we decide according to the circumstances.
When we do specify parameters, they may be assigned data types, and these types must match with what is expected, or the code will not run properly.
The parameter declaration methods default
, **kwargs
, and **args
are the parameters we work with when working on any function in Python.
But before diving into our main topic, we will first look into the basics of functions so it’s easier to work with function parameters.
What is a Function in Python
A function in Python can be defined as a list of statements that will be executed when the function is called. We can define a function by using the keyword def
and assigning it a name.
In this article, we will be covering the below-mentioned areas one by one with examples.
- Writing a function
- Function without parameters
- Functions with one parameter
- A function that returns a value
- Multiple arguments, multiple return values
Writing a Function
First, we will write a function of our need, which will work according to our requirements whenever we call it.
Suppose we want a function that takes any number and gives us the square of the number. Know that defining function is easy.
Now, all we need to do is to use the def
keyword, followed by the name of our function, followed by parentheses ()
, and followed by a colon :
.
Example code:
def my_first_function():
Function Without Parameters
In our scenario, we want a function that squares numbers to name our function square
. This is how we can define our square
function.
Example code:
def square():
Now, if we want our function to square the number we provide, we will have to give this functionality to our function square
.
- We will define the function
- Multiply the number with 2 and assign it to a variable
- Print the variable inside the function
- Call the function because if we do not call the function, we will not be able to get the output.
Example code:
def square():
value = 2 ** 2
print(value)
Remember that we never call the function within the function; we call it outside of the function.
square()
Output:
4
But, what if we want to give different numbers to the function so we can find the square of different numbers according to the situation?
Functions With One Parameter
This is where the parameter comes in handy. We will pass a parameter in the function.
So, whenever we want to know the square of any number, we will call the function and hand over that number to the function.
Within nanoseconds, we will have the square of any value. Let’s try it with an example, and let’s define the square
function again with a parameter.
Example code:
def square(number):
value = number ** 2
print(value)
Now, we will call the function and give it some random numbers to see if it gives back the correct squares.
square(5)
Output:
25
To find the square of 25, we will call our function square
and give it the value 25.
square(25)
Output:
625
Function That Returns a Value
If we do not print the value directly, we want to return it and assign it to another variable. We use the return
keyword.
See the example below.
def square(number):
values = number ** 2
return values
Now we will assign our function to a variable we have outside the function.
values = square(3)
To see the output of the above code, we will print the variable we have created.
print(values)
Output:
9
Multiple Arguments and Multiple Return Values
Now we will see:
- How a function accepts more than one parameter
- How can we pass multiple arguments to a function
- How can we get multiple return values
Let’s do all these with an example. We will define a function that accepts more than one parameter and takes multiple arguments and multiple return values.
Example code:
def raise_to_power(val, val2):
return val ** val2
Now we call the function and pass two values for the parameters mentioned in the function val, val2
.
print(raise_to_power(3, 5))
Output:
243
Default and Flexible Arguments in Python
Let’s say we are writing a function that takes multiple parameters, and there is also a common value for some of these parameters.
In this case, we would like to call a function without specifying every function. Sometimes, we would like some parameters to have default arguments used when it is not specified otherwise.
Now we will learn:
- How to write a function with a default argument
*args
- Flexible arguments allow us to pass any number of arguments in the function.**kwargs
- Keyword arguments
Define a function with a default argument value; in the function header, follow the parameter of interest with an equal sign and a default argument value.
Example code:
def power(word, pow=1):
words = word ** pow
return words
Now we will call our function power
, and we will only pass an argument for the parameter word
.
print(power(3))
Output:
3
The second parameter in the function has a default value of 1
and 3 power 1
= 3
.
Flexible Argument *args
in Python Function
When unsure of the number of arguments given to our function, we add a *
before the parameter name in the function specification.
Let’s suppose we want to write a function but aren’t sure how many arguments a user will pass. We will define a function that takes int
and floats
and adds them.
Example code:
def add_all(*number):
sum_all = 0
# apply for loop to sum all the parameter
for num in number:
sum_all += num
return sum_all
Now we will call the function and pass some arguments.
print(add_all(2, 45, 3.2, 45))
Output:
95.2
Keyword Arguments **kwargs
In the function, before the parameter name declaration, we add two asterisks, **
, when we are unsure of the number of keyword arguments that will be provided in our function.
This will allow the function to access the items appropriately after receiving a dictionary of arguments.
Example code:
def my_function(**name):
print("The child's last name is " + name["last_name"])
Now we call the function and pass some arguments.
my_function(first_name="Abid", last_name="Orakzai")
Output:
The child's last name is Orakzai
Conclusion
Thus, we get to know that there are three different kinds of parameters that the Python function can accept. These are the arbitrary *args
, keyword **kwargs
, and default parameters
.
While keyword arguments allow us to employ any order, default arguments assist us in dealing with the absence of values. Finally, Python’s arbitrary arguments *args
come in handy when we don’t know how many arguments we’ll get.
We hope you find this article helpful in understanding the basic idea of function parameter types used in Python.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn