How to Escape Curly Braces Using format() in Python
-
Understanding the
format()
Method -
Print Curly Braces in a String Using the
format()
Method in Python
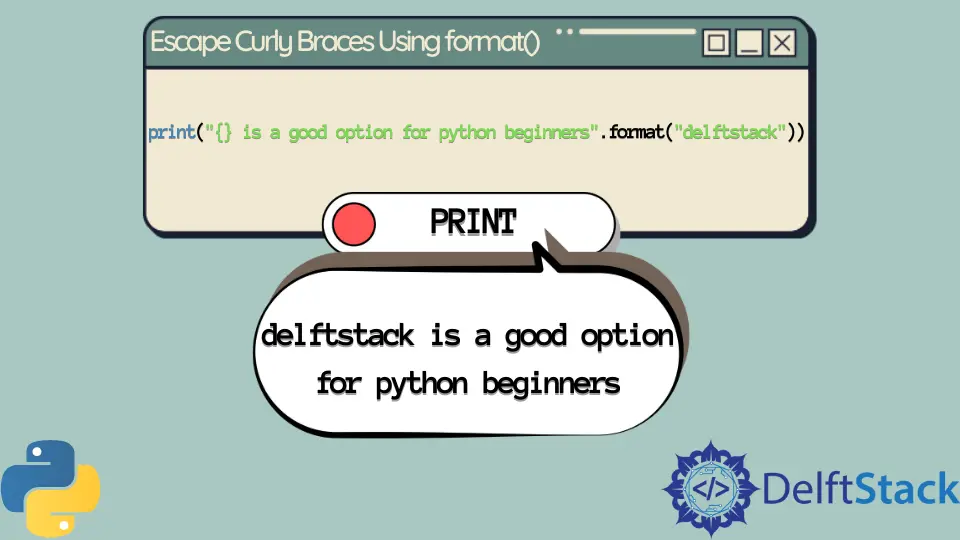
Escape in programming language means to print the characters in the form of literal text, which is unprintable in the code. In this article, we will learn about the format()
method and how we can escape curly brace characters {}
using the format()
function.
Understanding the format()
Method
The format()
method is an essential built-in function in Python, primarily used for formatting strings and producing formatted output according to our desired specifications. Its syntax is straightforward and consists of a string containing placeholders within curly braces {}
.
Syntax:
"{}".format(value)
Let’s break down the components of this syntax:
- Curly Braces
{}
: These serve as formatters or placeholders within the string. The content inside these braces is subject to replacement when theformat()
method is called. value
: This represents the value or values that will replace the placeholders within the string. It can be any string, character, or variable that you want to incorporate into the final formatted output.
Example:
# Python 3.x
print("{} is a good option for Python beginners".format("DelftStack"))
Output:
DelftStack is a good option for Python beginners
Print Curly Braces in a String Using the format()
Method in Python
In this example, the format()
function is employed to replace the placeholder {}
with the value "DelftStack"
. The resulting output reflects this substitution, showcasing how the format()
method can transform a template string into a formatted and informative message.
Now, what if you want to print curly braces {}
as part of your string while using the format()
method? To achieve this, you need to use double curly braces {{
and }}
within the placeholder. In other words, you use three pairs of curly braces instead of one.
Example 1:
# Python 3.x
print("{{{0}}} is a good option for Python beginners".format("DelftStack"))
Output:
{DelftStack} is a good option for Python beginners
In this code snippet, we’ve used triple curly braces {{{
, {0}
, and }}}
. The inner pair of curly braces {0}
acts as a placeholder for the value "DelftStack"
, and the outer curly braces {{
and }}
escape the inner curly braces, treating them as literal characters.
Example 2:
myname = "Jessica Frank"
myage = 24
print("Name: {{{0}}} Age:{{{1}}}".format(myname, myage))
Output:
Name: {Jessica Frank} Age:{24}
In this second example, we’ve applied the same principle. The triple curly braces {{{
, {0}
, and }}}
allow us to include both the name and age as literal text within the formatted string.
In summary, when you need to include curly braces {}
as part of your formatted string while using the format()
method, use triple curly braces {{{
, {}
, and }}}
as placeholders. This technique enables you to achieve the desired output format and includes literal curly braces in your string.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn