How to Find First Occurrence in a String in Python
-
Use the
find()
Function to Find First Occurrence in Python -
Use the
index()
Function to Find First Occurrence in Python -
Use the
rfind()
andrindex()
Functions to Find Last Occurrence in Python - Conclusion
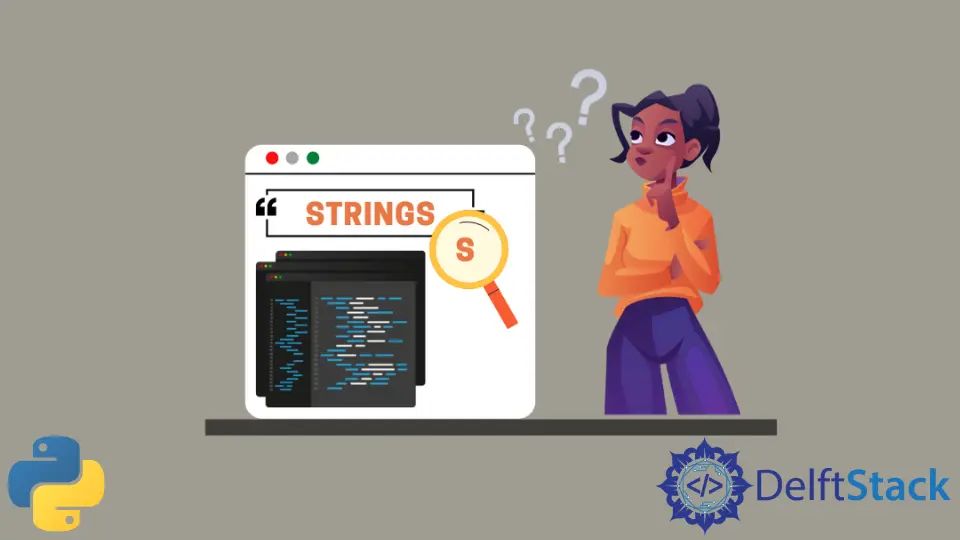
Effective manipulation of strings is a fundamental aspect of Python programming, and finding the first occurrence of a substring can be a common and essential task.
This tutorial will discuss the methods to find the first occurrence of a substring inside a string in Python.
Use the find()
Function to Find First Occurrence in Python
We can use the find()
function in Python to find the first occurrence of a substring inside a string. The find()
function takes the substring as an input parameter and returns the first starting index of the substring inside the main string.
This function returns -1
if the substring isn’t present in the main string.
Syntax:
index = string.find(substring)
Parameter:
substring
: This is the substring you are searching for within the main string. It’s the text you want to find the first occurrence of.
Code Example:
string = "This guy is a crazy guy."
print(string.find("guy"));
In the code, we start by defining a variable called string
and assign a value "This guy is a crazy guy."
. Then, we use the find()
function to search for the first occurrence of the substring "guy"
within the string
.
The find()
function returns the index (position) where the first occurrence of the substring begins within the main string. It does not modify the original string; it’s a read-only operation.
Output:
5
The find()
function finds the first occurrence of "guy"
in the string
, and it returns 5
. This means that the substring "guy"
starts at the 5th character position within the string
.
Note that this function also counts a blank space as a character.
Use the index()
Function to Find First Occurrence in Python
Using the index()
function is similar to the previously discussed find()
function, as it takes the substring as an input parameter and returns the first occurrence of the substring inside the main string’s starting index.
The following syntax executes to find the first occurrence of the substring
within the string
and to return its position or index. If the substring
is not found, this function raises a ValueError
exception to signal that the search term is not present in the string
.
Syntax:
index = string.index(substring)
Parameter:
substring
: This is the substring you are searching for within the main string. It’s the specific text you want to find the first occurrence of.
Code Example:
string = "This guy is a crazy guy."
print(string.index("guy"));
In the code, we start by defining a variable called string
and assign a value "This guy is a crazy guy."
. Next, we use the index()
function to find the first occurrence of the substring "guy"
within the string
.
The index()
function returns the position or index where this substring first appears within the string
.
Output:
5
Like the find()
function, the index()
function also returns 5
as the starting index of the first occurrence of the string "guy"
inside the "This guy is a crazy guy"
string.
Use the rfind()
and rindex()
Functions to Find Last Occurrence in Python
The two functions discussed previously locate the substring inside the main string from left to right. If we want to locate the substring from right to left, also called the last occurrence of the substring, we can use the rfind()
and rindex()
functions.
These functions are similar to their counterparts discussed in the previous examples, except they look from right to left. The following code snippets show the utilization of both functions in Python.
Syntax of rfind()
:
index = string.rfind(substring)
Parameter:
substring
: This is the substring you are searching for within the main string. It’s the specific text you want to find the last occurrence of within the string.
Code example using rfind()
:
string = "This guy is a crazy guy."
print(string.rfind("guy"));
In the code, we start by defining a variable called string
and assign a value "This guy is a crazy guy."
. Next, we used the print()
function to display the result of the rfind()
function.
We’re applying the rfind()
function to our string
variable to determine the position of the last occurrence of the substring "guy"
within the string
.
Output:
20
The rfind()
function returns 20
. This means that the last occurrence of the substring "guy"
in our string
is at character position 20
.
Now, let’s have an example of using the rindex()
function.
Syntax of rindex()
:
index = string.rindex(substring)
Parameter:
substring
: This is the substring you are searching for within the main string. It’s the specific text you want to find the last occurrence of within the string.
Code example using rindex()
:
string = "This guy is a crazy guy."
print(string.rindex("guy"));
In the code, we start by defining a variable called string
and assign a value "This guy is a crazy guy."
. Then, we utilize the print()
function to display the result of the rindex()
function.
We’re applying the rindex()
function to our string
variable to determine the position of the last occurrence of the substring "guy"
within the string
.
Output:
20
The rindex()
function returns 20
. This means that the last occurrence of the substring "guy"
in our string
is at character position 20
.
The main difference between rfind()
and rindex()
is how they handle cases where the substring is not found. rfind()
returns -1
, while rindex()
raises a ValueError
.
Conclusion
In Python, knowing how to find the first occurrence of a specific word or phrase within a bigger piece of text is an important skill for programmers. This article has explored different ways to do this, mainly using the find()
and index()
functions to locate the first instance and the rfind()
and rindex()
functions to find the last one.
By using these techniques in your Python programs, you can efficiently work with text, making it easier to create versatile and sturdy applications.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn