How to Find All Indexes of a Character in Python String
- Find All Indexes of a Character Inside a String With Regular Expressions in Python
-
Find All Indexes of a Character Inside a String With the
yield
Keyword in Python - Find All Indexes of a Character Inside a String With List Comprehensions in Python
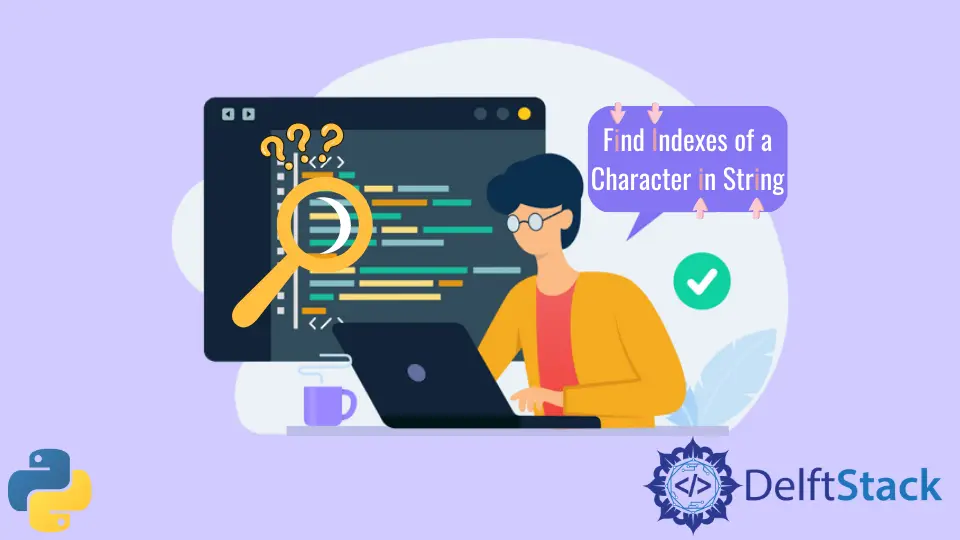
This tutorial will discuss the methods to get all the indexes of a character inside a string in Python.
Find All Indexes of a Character Inside a String With Regular Expressions in Python
We can use the finditer()
function inside the re
module of Python for our specific problem. The finditer()
function takes the pattern and the string as input parameters. It reads the string from left to right and returns all the indexes where the pattern occurs. We can use this function with list comprehensions to store the result inside a list in Python. The following code snippet shows us how to find all indexes of a character inside a string with regular expressions.
import re
string = "This is a string"
char = "i"
indices = [i.start() for i in re.finditer(char, string)]
print(indices)
Output:
[2, 5, 13]
We used the re.finditer()
function to iterate through each character inside the string
and find all the characters that matched char
. We returned the index i.start()
of all the characters that matched char
in the form of a list and stored them inside indices
. In the end, we displayed all the elements of indices
.
Find All Indexes of a Character Inside a String With the yield
Keyword in Python
We can also use the yield
keyword inside a function to solve our specific problem. The yield
keyword is used to return multiple values from a function without destroying the state of the local variables of that function. When the function is called again, the execution starts from the previous yield
statement. We can use this keyword to return all the instances of a character inside a string. The following code example shows us how to find all the indexes of a character inside a string with the yield
keyword.
def find(string, char):
for i, c in enumerate(string):
if c == char:
yield i
string = "This is a string"
char = "i"
indices = list(find(string, char))
print(indices)
Output:
[2, 5, 13]
We defined the find(string, char)
function that iterates through each character inside the string
and yields the index i
if the character matches char
. While calling the find()
function, we stored all the returned values inside a list and displayed all the elements of the list.
Find All Indexes of a Character Inside a String With List Comprehensions in Python
We can also use list comprehensions to solve our problem. List comprehensions are used to create new lists based on previously existing lists. We can use list comprehensions to iterate through each character inside the string variable and return the index if the character matches the desired character. List comprehensions return values in the form of lists. We can store these index values inside a list and display the results. The following code snippet shows us how to find all indexes of a character inside a string with list comprehensions.
string = "This is a string"
char = "i"
indices = [i for i, c in enumerate(string) if c == char]
print(indices)
Output:
[2, 5, 13]
We used list comprehensions to iterate through each character c
inside the string
variable and returned its index i
if the character c
is equal to our desired character char
. This is the simplest and the easiest way to find all the indexes of a character inside a string.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn