Python Determinant
-
Use
numpy.linalg.det()
to Calculate the Determinant of Matrix in Python -
Use the
symPy
Library to Calculate the Determinant of Matrix in Python
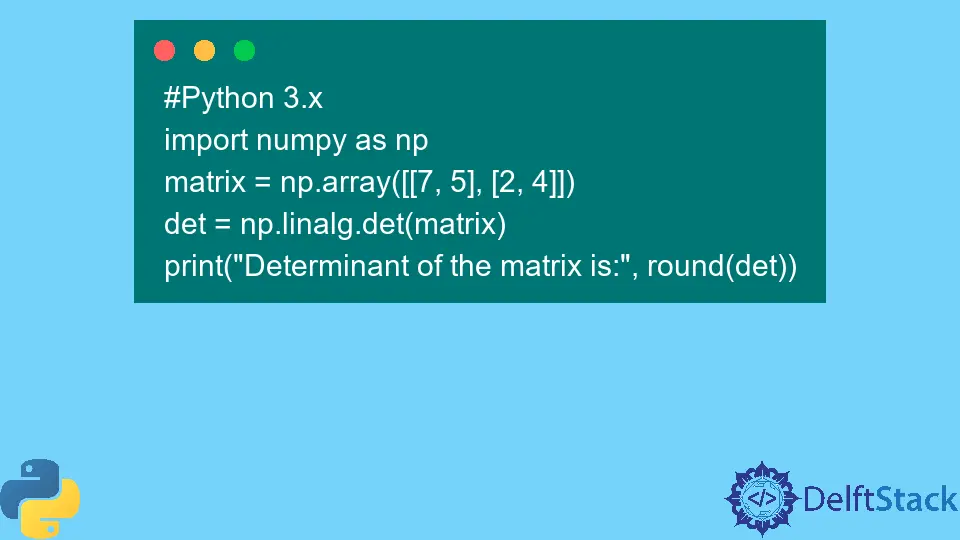
The determinant
of a matrix is a scalar number that is only associated with square matrices. For a square matrix [[1,2], [3,4]]
, the determinant is calculated as (1x4) - (2x3)
.
Use numpy.linalg.det()
to Calculate the Determinant of Matrix in Python
The NumPy
package has a module named linalg
which stands for linear algebra. This module provides a built-in method det()
to calculate the determinant of a matrix in Python.
To use the NumPy
package, we must install it first using the following command.
#Python 3.x
pip install numpy
After the installation, we can find the determinant of any square matrix using the following syntax.
Syntax:
# Python 3.x
numpy.linalg.det(matrix)
Determinant of a 2x2
Matrix in Python
In the following code, we have created a 2x2
NumPy array and calculated the determinant of the matrix using the det()
method. Finally, we have rounded off the determinant because this method returns the determinant as a float data type.
Example Code:
# Python 3.x
import numpy as np
matrix = np.array([[7, 5], [2, 4]])
det = np.linalg.det(matrix)
print("Determinant of the matrix is:", round(det))
Output:
#Python 3.x
Determinant of the matrix is: 18
Determinant of a 3x3
Matrix in Python
We can calculate the determinant of a 3x3
or any dimension of a square matrix using the same procedure. In the following code, we constructed a 3x3
NumPy array and used the det()
method to determine the determinant of the matrix.
Example Code:
# Python 3.x
import numpy as np
matrix = np.array([[7, 5, 3], [2, 4, 1], [5, 8, 6]])
det = np.linalg.det(matrix)
print("Determinant of the matrix is:", round(det))
Output:
#Python 3.x
Determinant of the matrix is: 65
Use the symPy
Library to Calculate the Determinant of Matrix in Python
The symPy
is an open-source library in Python for symbolic computation. We can perform various algebraic and other mathematical operations using this library.
To use symPy
, we have to install it first using the following command.
#Python 3.x
pip install sympy
Determinant
of a 2x2
Matrix in Python
We have created a 2x2
matrix using the sympy.Matrix()
method in the following code. Then we have found the determinant by calling the det()
method with the matrix.
Example Code:
# Python 3.x
import sympy as sp
matrix = sp.Matrix([[7, 5], [2, 4]])
determinant = matrix.det()
print("Determinant of the matrix is:", determinant)
Output:
#Python 3.x
Determinant of the matrix is: 18
Determinant
of a 3x3
Matrix in Python
The procedure is the same for a 3x3
matrix or a square matrix of any dimension to find the determinant. In the following code, we have created a 3x3
matrix and found its determinant using the det()
method with the matrix.
Example Code:
# Python 3.x
import sympy as sp
matrix = sp.Matrix([[7, 5, 3], [2, 4, 1], [5, 8, 6]])
determinant = matrix.det()
print("Determinant of the matrix is:", determinant)
Output:
#Python 3.x
Determinant of the matrix is: 65
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn