Python 行列式
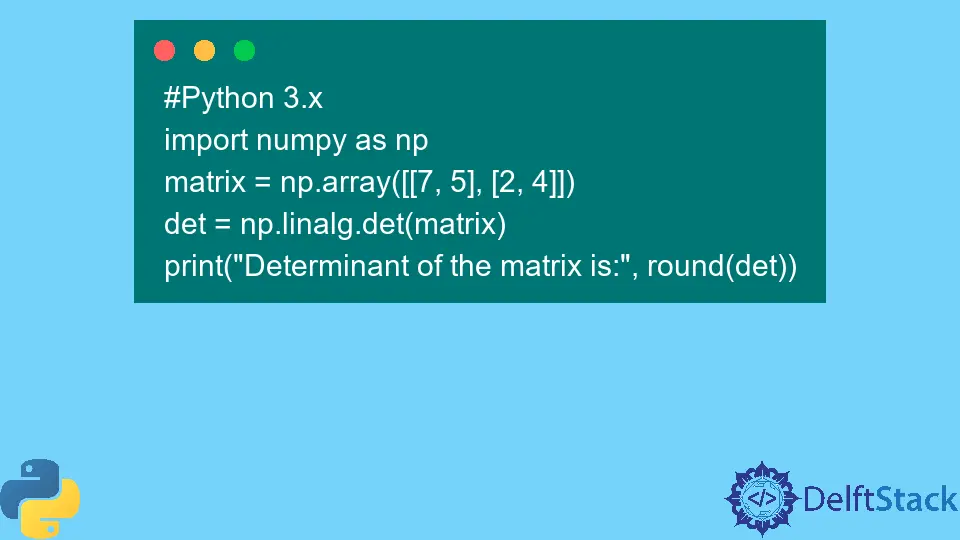
行列の行列式
は、正方行列にのみ関連付けられるスカラー数です。 正方行列 [[1,2], [3,4]]
の場合、行列式は (1x4) - (2x3)
として計算されます。
numpy.linalg.det()
を使用して Python で行列式を計算する
NumPy
パッケージには、線形代数を表す linalg
という名前のモジュールがあります。 このモジュールは、組み込みメソッド det()
を提供して、Python で行列式を計算します。
NumPy
パッケージを使用するには、最初に次のコマンドを使用してインストールする必要があります。
#Python 3.x
pip install numpy
インストール後、次の構文を使用して正方行列の行列式を見つけることができます。
構文:
# Python 3.x
numpy.linalg.det(matrix)
Python での 2x2
行列の行列式
次のコードでは、2x2
NumPy 配列を作成し、det()
メソッドを使用して行列式を計算しています。 最後に、このメソッドは行列式を float データ型として返すため、行列式を四捨五入しました。
コード例:
# Python 3.x
import numpy as np
matrix = np.array([[7, 5], [2, 4]])
det = np.linalg.det(matrix)
print("Determinant of the matrix is:", round(det))
出力:
#Python 3.x
Determinant of the matrix is: 18
Python での 3x3
行列の行列式
同じ手順を使用して、3x3
または正方行列の任意の次元の行列式を計算できます。 次のコードでは、3x3
NumPy 配列を作成し、det()
メソッドを使用して行列式を決定しています。
コード例:
# Python 3.x
import numpy as np
matrix = np.array([[7, 5, 3], [2, 4, 1], [5, 8, 6]])
det = np.linalg.det(matrix)
print("Determinant of the matrix is:", round(det))
出力:
#Python 3.x
Determinant of the matrix is: 65
symPy
ライブラリを使用して Python で行列式を計算する
symPy
は、シンボリック計算用の Python のオープンソース ライブラリです。 このライブラリを使用して、さまざまな代数演算やその他の数学演算を実行できます。
symPy
を使用するには、最初に次のコマンドを使用してインストールする必要があります。
#Python 3.x
pip install sympy
Python での2x2
行列の行列式
次のコードでは、sympy.Matrix()
メソッドを使用して 2x2
マトリックスを作成しました。 次に、行列で det()
メソッドを呼び出して行列式を見つけました。
コード例:
# Python 3.x
import sympy as sp
matrix = sp.Matrix([[7, 5], [2, 4]])
determinant = matrix.det()
print("Determinant of the matrix is:", determinant)
出力:
#Python 3.x
Determinant of the matrix is: 18
Python での3x3
行列の行列式
行列式を見つける手順は、3x3
行列または任意の次元の正方行列の場合と同じです。 次のコードでは、3x3
マトリックスを作成し、マトリックスで det()
メソッドを使用してその行列式を見つけました。
コード例:
# Python 3.x
import sympy as sp
matrix = sp.Matrix([[7, 5, 3], [2, 4, 1], [5, 8, 6]])
determinant = matrix.det()
print("Determinant of the matrix is:", determinant)
出力:
#Python 3.x
Determinant of the matrix is: 65
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn