Determinante de Python
-
Use
numpy.linalg.det()
para calcular el determinante de Matrix en Python -
Use la biblioteca
symPy
para calcular el determinante de Matrix en Python
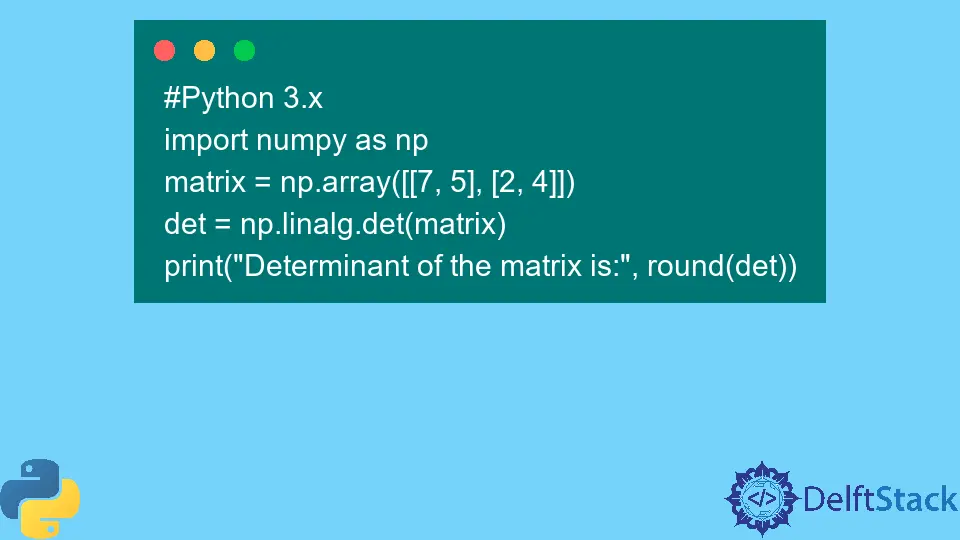
El determinante
de una matriz es un número escalar que sólo se asocia a matrices cuadradas. Para una matriz cuadrada [[1,2], [3,4]]
, el determinante se calcula como (1x4) - (2x3)
.
Use numpy.linalg.det()
para calcular el determinante de Matrix en Python
El paquete NumPy
tiene un módulo llamado linalg
que significa álgebra lineal. Este módulo proporciona un método integrado det()
para calcular el determinante de una matriz en Python.
Para usar el paquete NumPy
, primero debemos instalarlo usando el siguiente comando.
#Python 3.x
pip install numpy
Después de la instalación, podemos encontrar el determinante de cualquier matriz cuadrada usando la siguiente sintaxis.
Sintaxis:
# Python 3.x
numpy.linalg.det(matrix)
Determinante de una matriz 2x2
en Python
En el siguiente código, hemos creado una matriz NumPy 2x2
y calculado el determinante de la matriz usando el método det()
. Finalmente, hemos redondeado el determinante porque este método devuelve el determinante como un tipo de datos flotante.
Código de ejemplo:
# Python 3.x
import numpy as np
matrix = np.array([[7, 5], [2, 4]])
det = np.linalg.det(matrix)
print("Determinant of the matrix is:", round(det))
Producción :
#Python 3.x
Determinant of the matrix is: 18
Determinante de una matriz 3x3
en Python
Podemos calcular el determinante de un 3x3
o cualquier dimensión de una matriz cuadrada utilizando el mismo procedimiento. En el siguiente código, construimos una matriz NumPy 3x3
y usamos el método det()
para determinar el determinante de la matriz.
Código de ejemplo:
# Python 3.x
import numpy as np
matrix = np.array([[7, 5, 3], [2, 4, 1], [5, 8, 6]])
det = np.linalg.det(matrix)
print("Determinant of the matrix is:", round(det))
Producción :
#Python 3.x
Determinant of the matrix is: 65
Use la biblioteca symPy
para calcular el determinante de Matrix en Python
El symPy
es una biblioteca de código abierto en Python para el cálculo simbólico. Podemos realizar varias operaciones algebraicas y otras operaciones matemáticas utilizando esta biblioteca.
Para usar symPy
, primero tenemos que instalarlo usando el siguiente comando.
#Python 3.x
pip install sympy
Determinante
de una matriz 2x2
en Python
Hemos creado una matriz 2x2
usando el método sympy.Matrix()
en el siguiente código. Entonces hemos encontrado el determinante llamando al método det()
con la matriz.
Código de ejemplo:
# Python 3.x
import sympy as sp
matrix = sp.Matrix([[7, 5], [2, 4]])
determinant = matrix.det()
print("Determinant of the matrix is:", determinant)
Producción :
#Python 3.x
Determinant of the matrix is: 18
Determinante
de una matriz 3x3
en Python
El procedimiento es el mismo para una matriz 3x3
o una matriz cuadrada de cualquier dimensión para encontrar el determinante. En el siguiente código, creamos una matriz 3x3
y encontramos su determinante usando el método det()
con la matriz.
Código de ejemplo:
# Python 3.x
import sympy as sp
matrix = sp.Matrix([[7, 5, 3], [2, 4, 1], [5, 8, 6]])
determinant = matrix.det()
print("Determinant of the matrix is:", determinant)
Producción :
#Python 3.x
Determinant of the matrix is: 65
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn