Python Destructor
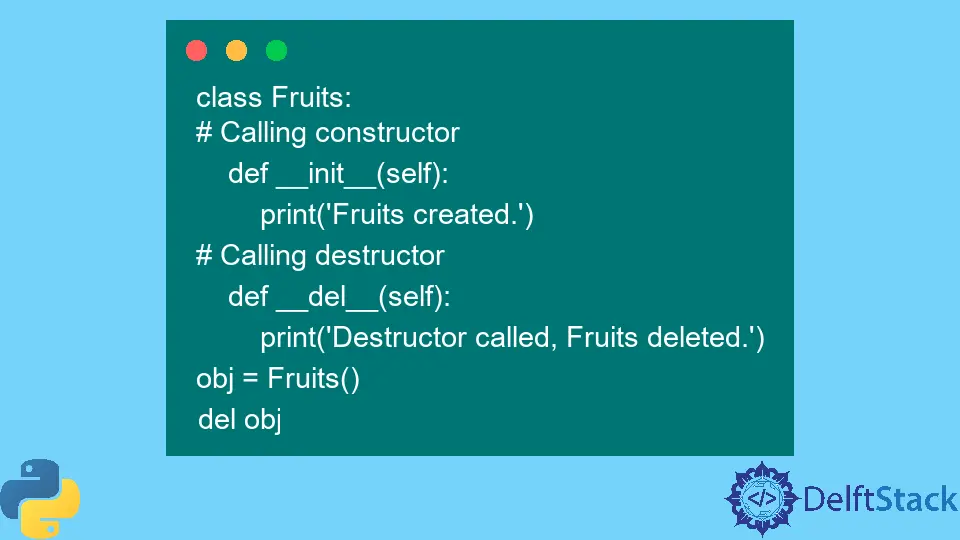
Destructors are called upon when a Python object needs to be cleaned up. It basically has a completely opposite role of a constructor and is used to reverse the operations that a constructor performs. Destructors are mainly deployed to organize in a program and to implement the standards for coding.
This tutorial demonstrates the use of a destructor in Python.
The need for destructors in Python is not as much as it is in other programming languages like C++ as Python’s garbage collector automatically handles memory management. However, destructors do exist in Python, and this article explains their use and functioning.
We use the __del__()
function as the destructor in Python. When the programmer calls the __del__()
function, all the object references get removed, which is known as garbage collection.
The syntax for the destructor is as follows:
def __del__(self):
# Write destructor body here
The advantages of using destructor in Python programs are below.
- Memory space is freed as unnecessary space-consuming objects are deleted automatically.
- It is easy as it gets invoked automatically.
The following code uses a destructor in Python.
class Fruits:
# Calling constructor
def __init__(self):
print("Fruits created.")
# Calling destructor
def __del__(self):
print("Destructor called, Fruits deleted.")
obj = Fruits()
del obj
The above code provides the following output:
Fruits created.
Destructor called, Fruits deleted.
The above code shows that the destructor has been called after the program was ended or when all the given references to the object are deleted. This means that the value of the reference count for the given object, after a point of time, becomes zero and not when the given object shifts out of scope.
Here is another code that explains the destructor further.
class Fruits:
def __del__(self):
print("Fruits deleted")
a = Fruits()
del a
a = Fruits()
b = a
del b
del a
In the above code, a class Fruits
is defined, and the object a
is a reference to the class while the object b
is a local copy of that reference a
. When b
is deleted, the function is not called because it only holds the local copy and nothing else.
Both constructors and destructors are essential in the world of programming, no matter which language the programmer uses. Destructors play an important role in garbage collection. We might not see big changes in small chunks of code, but in complex programs and production-level codes, where memory usage is a big priority, the importance of destructors is visible quite clearly.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn