How to Convert Python Datetime to Epoch
-
Use the
total_seconds()
Method to ConvertDatetime
toepoch
in Python -
Use the
timestamp()
Function to ConvertDatetime
toepoch
in Python -
Use the
strftime(format)
Function to ConvertDatetime
toepoch
in Python -
Using
strftime
andstrptime
to Convert PythonDatetime
toepoch
-
Use the
timegm
Function to ConvertDateTime
toepoch
in Python -
Using External Libraries (
arrow
) to Convert PythonDatetime
toepoch
-
Using the
time
Module to Convert PythonDatetime
toepoch
- Conclusion
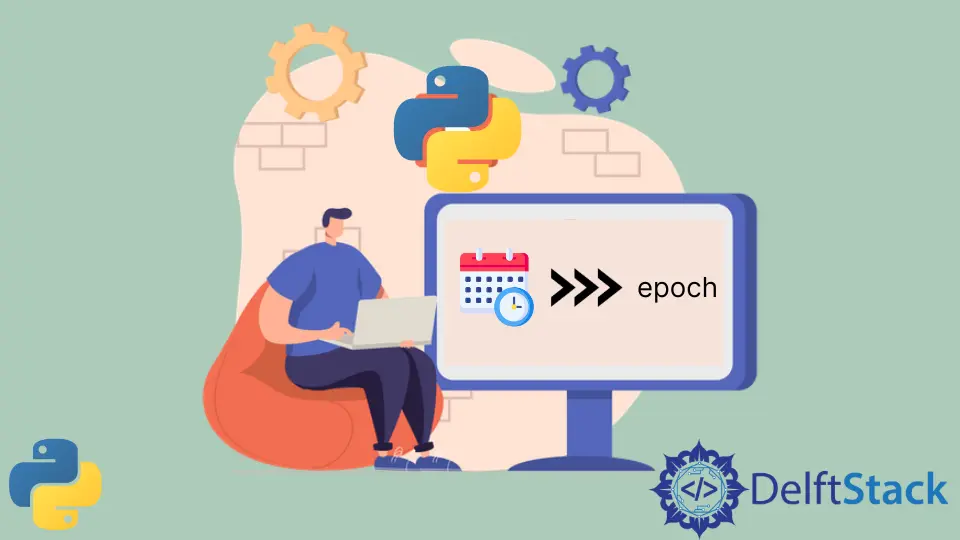
Working with dates and times is a common task in Python development, and there are situations where it becomes necessary to convert DateTime
objects to epoch
timestamps. The epoch
time, often represented as the number of seconds or milliseconds since January 1, 1970
(Unix epoch
), is a standard way to handle time across different systems.
The datetime
library can be imported into a Python program. It provides classes used in manipulating date and time in the Python code.
epoch
is the starting point of measuring the time elapsed, and its value usually varies and depends on the platform being used.
In this tutorial, we will demonstrate various methods to convert DateTime
to epoch
in Python, each method offering unique advantages and use cases.
Use the total_seconds()
Method to Convert Datetime
to epoch
in Python
The total_seconds()
method is part of the timedelta
class in Python’s datetime
module. This method returns the total number of seconds contained in a timedelta
object.
A timedelta
represents the difference between two dates or times and is commonly used for performing arithmetic with DateTime
objects.
The formula for converting a DateTime
object to epoch
time using total_seconds()
involves calculating the difference between the DateTime
object and the Unix epoch
(January 1, 1970
). This difference, represented as a timedelta
, can then be passed to total_seconds()
to obtain the total seconds.
The code below uses the explicit method to convert datetime
to epoch
in Python.
import datetime
ts = (
datetime.datetime(2024, 1, 23, 0, 0) - datetime.datetime(1970, 1, 1)
).total_seconds()
print(ts)
Output:
1705968000.0
In this code, we take the current date and manually subtract it from the starting date and then convert it into seconds by using the total_seconds()
function and displaying it. The initial date here is 1970/1/1
.
Handling Time Zones With pytz
When dealing with DateTime
objects that include time zone information, it’s recommended to use the pytz
library for accurate and reliable conversions.
Here’s an example:
from datetime import datetime
import pytz
# Creating a DateTime object with time zone information
current_time_utc = datetime.now(pytz.utc)
# Calculating the difference from the Unix epoch
epoch_difference = current_time_utc - datetime(1970, 1, 1, tzinfo=pytz.utc)
# Converting the difference to total seconds
epoch_time = epoch_difference.total_seconds()
print("Epoch Time (UTC):", epoch_time)
Output:
Epoch Time (UTC): 1705949990.479682
In this example, pytz.utc
is used to represent Coordinated Universal Time (UTC), ensuring proper handling of time zones during the conversion.
Use the timestamp()
Function to Convert Datetime
to epoch
in Python
The timestamp()
method is a built-in feature of the datetime
class in Python. This method returns the POSIX timestamp, which is the number of seconds that have passed since the Unix epoch
(January 1, 1970, at 00:00:00 UTC
).
The timestamp()
method is a straightforward and efficient way to obtain a numeric representation of a DateTime
object, making it a commonly used approach for converting DateTime
to epoch
in Python.
A timestamp is a series of characters that dictates the value of when a particular event occurred. Python provides a function timestamp()
, which can be used to get the timestamp of datetime
since the epoch
.
The code below uses the timestamp()
function to convert datetime
to epoch
in Python.
import datetime
ts = datetime.datetime(2024, 1, 23, 0, 0).timestamp()
print(ts)
Output:
1705968000.0
This is a fairly easy method and provides us with accurate outputs.
Note that the timestamp()
function only works on Python 3.3+ and is not applicable for use in the older versions of Python.
Handling Time Zones With pytz
When working with DateTime
objects that include time zone information, it’s recommended to use the pytz
library for accurate and reliable conversions. Here’s an example:
from datetime import datetime
import pytz
# Creating a DateTime object with time zone information
current_time_utc = datetime.now(pytz.utc)
# Convert DateTime to epoch using timestamp()
epoch_time = current_time_utc.timestamp()
print("Epoch Time (UTC):", epoch_time)
Output:
Epoch Time (UTC): 1705950328.425532
In this example, pytz.utc
is used to represent Coordinated Universal Time (UTC), ensuring proper handling of time zones during the conversion.
Use the strftime(format)
Function to Convert Datetime
to epoch
in Python
The strftime
method is part of the datetime
class in Python and is used for formatting DateTime
objects as strings. The name strftime
stands for string format time
.
It takes a format string as an argument, where various format codes are used to represent different components of the DateTime
object (such as year, month, day, hour, etc.).
The strftime(format)
method is used to convert an object to a string based on the format specified by the user. For the reverse of this process, the strptime()
method is used.
The code below uses the strftime(format)
method to convert datetime
to epoch
in Python.
import datetime
ts = datetime.datetime(2024, 1, 23, 0, 0).strftime("%s")
print(ts)
Output:
1705968000
strftime(format)
might not always provide the correct solution. This method uses the %s
directive as an argument of strftime
, which Python doesn’t actually support. It could work because Python forwards %s
to the system’s strftime
method.
This method is not the most accurate method for the conversion of datetime
to epoch
. It is not recommended to use this method simply because there are better and more accurate methods.
Using strftime
and strptime
to Convert Python Datetime
to epoch
Another method involves formatting the DateTime
object as a string and then parsing it back to a DateTime
object. Afterward, you can use the timestamp()
method.
Conversely, the strptime
method, which stands for string parse time
, is used for parsing strings into DateTime
objects. It is also part of the datetime
class and requires a format string that specifies how the input string is structured.
Together, the strftime
and strptime
methods provide a powerful mechanism for converting DateTime
objects to strings and parsing strings back into DateTime
objects.
Example Code:
from datetime import datetime
# Creating a DateTime object
current_time = datetime.now()
# Convert DateTime to epoch using strftime and strptime
formatted_time = current_time.strftime("%Y-%m-%d %H:%M:%S")
parsed_time = datetime.strptime(formatted_time, "%Y-%m-%d %H:%M:%S")
epoch_time = parsed_time.timestamp()
print("Epoch Time:", epoch_time)
Output:
Epoch Time: 1705950763.0
In this code example, we first create a DateTime
object representing the current date and time. We then format the DateTime
object as a string using strftime
with a format specifier.
Next, we parse the formatted string back into a DateTime
object using strptime
. We use the timestamp()
method on the parsed DateTime
object to get the epoch
time.
Lastly, we print the resulting epoch
time.
Use the timegm
Function to Convert DateTime
to epoch
in Python
The timegm()
function takes a specific time value and returns its corresponding Unix timestamp value. The epoch
is taken as 1970, and the POSIX encoding is assumed. time.gmtime()
and timegm()
functions are the inverse of each other.
Both calendar
and time
libraries need to be imported into the Python program to use these functions. The calendar
module provides us with the ability to output calendars and some additional and useful functions related to it.
The following code uses the timegm()
function to convert the Python datetime
to epoch
.
import datetime
import calendar
d = datetime.datetime(2024, 1, 23, 0, 0)
print(calendar.timegm(d.timetuple()))
Output:
1705968000
Using External Libraries (arrow
) to Convert Python Datetime
to epoch
There are external libraries, such as arrow
, that provide additional functionalities for handling DateTime
objects, including direct conversion to epoch
time.
Arrow
is an external Python library designed to simplify DateTime
operations. It extends the functionality provided by the built-in datetime
module, offering additional features such as easy formatting, parsing, time zone handling, and precise arithmetic.
Installation
Before diving into examples, ensure that arrow
is installed. You can install it using the following command:
pip install arrow
Now, let’s explore how arrow
can be employed for DateTime
to epoch
conversion.
Let’s begin with a simple example demonstrating how arrow
can be used to convert a DateTime
object to epoch
time.
import arrow
# Creating an Arrow object
current_time = arrow.utcnow()
# Convert Arrow to epoch using timestamp()
epoch_time = current_time.timestamp()
print("Epoch Time:", epoch_time)
Output:
Epoch Time: 1705951506.729986
In the above code example, we start by installing the Arrow
library using the pip install arrow
. We then import the arrow
module.
We create an Arrow
object representing the current UTC using arrow.utcnow()
. Next, we use the timestamp()
method on the Arrow
object to obtain the epoch
time.
Lastly, we print the resulting epoch
time.
Time Zone Handling With Arrow
One of Arrow
’s notable features is its robust support for time zones. Let’s explore an example that incorporates time zone handling during DateTime
to epoch
conversion.
import arrow
# Creating an Arrow object with time zone information
current_time = arrow.now("US/Eastern")
# Convert Arrow to epoch using timestamp()
epoch_time = current_time.timestamp()
print("Original DateTime (Eastern Time):", current_time)
print("Epoch Time:", epoch_time)
Output:
Original DateTime (Eastern Time): 2024-01-22T14:27:01.584380-05:00
Epoch Time: 1705951621.58438
In this example, arrow.now('US/Eastern')
creates an Arrow
object representing the current time in the Eastern Time zone. The resulting epoch
time takes the time zone into account, providing accurate and reliable conversions.
Using the time
Module to Convert Python Datetime
to epoch
The time
module in Python provides various time-related functions, and it includes a method named time()
that is particularly useful for obtaining the current time in epoch
format. The epoch
time is defined as the number of seconds that have elapsed since the Unix epoch
(January 1, 1970, 00:00:00 UTC
).
Let’s dive into an example to illustrate the process of converting a DateTime
object to epoch
time using the time
module.
from datetime import datetime
import time
# Creating a DateTime object
current_time = datetime.now()
# Convert DateTime to epoch using time.mktime()
epoch_time = time.mktime(current_time.timetuple())
print("Epoch Time:", epoch_time)
Output:
Epoch Time: 1705951705.0
We first import the datetime
and time
modules. Next, we create a DateTime
object representing the current date and time.
We then use time.mktime()
to convert the DateTime
object to epoch
time. This method requires a struct_time
, which is obtained from the DateTime
object using timetuple()
.
Finally, we print the resulting epoch
time.
Handling Time Zones With datetime
When working with DateTime
objects that include time zone information, the datetime
module provides a more comprehensive solution. Let’s explore an example:
from datetime import datetime, timezone
# Creating a DateTime object with time zone information
current_time = datetime.now(timezone.utc)
# Convert DateTime to epoch using timestamp()
epoch_time = current_time.timestamp()
print("Original DateTime (UTC):", current_time)
print("Epoch Time:", epoch_time)
Output:
Original DateTime (UTC): 2024-01-22 19:31:16.303222+00:00
Epoch Time: 1705951876.303222
In this example, datetime.now(timezone.utc)
creates a DateTime
object representing the current time in UTC. The resulting epoch
time takes the time zone into account, providing accurate and reliable conversions.
Conclusion
Handling dates and times is a fundamental aspect of many programming tasks, and Python offers several methods for converting DateTime
objects to epoch
timestamps. In this comprehensive tutorial, we explored various techniques, each catering to different use cases.
-
Using
total_seconds()
Method:- Utilizes the
total_seconds()
method of thetimedelta
class to calculate the difference between aDateTime
object and the Unixepoch
. - Ensures accurate conversion and is suitable for scenarios requiring precise time differences.
- Utilizes the
-
Using
timestamp()
Function:- Leverages the built-in
timestamp()
method of thedatetime
class, providing a straightforward and efficient way to obtainepoch
time. - Offers a simple approach for converting
DateTime
objects toepoch
timestamps.
- Leverages the built-in
-
Using
strftime
andstrptime
Functions:- Demonstrates the use of
strftime
to formatDateTime
objects as strings andstrptime
to parse them back intoDateTime
objects. - Useful for scenarios where intermediate string representation is required before conversion.
- Demonstrates the use of
-
Using
timegm
Function:- Explores the
timegm()
function from thecalendar
andtime
modules to convertDateTime
objects toepoch
timestamps. - Useful when fine-grained control over
epoch
time calculation is needed.
- Explores the
-
Using External Library (
arrow
):- Introduces the
arrow
library, an external Python library providing enhanced functionality for handlingDateTime
objects. - Showcases straightforward methods for direct conversion to
epoch
time, with added support for time zones.
- Introduces the
-
Using
time
Module:- Utilizes the
time
module’smktime()
function to convertDateTime
objects toepoch
timestamps. - Offers a direct approach, especially when dealing with the current time.
- Utilizes the
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn