How to Convert Datetime to Date in Python
-
Use the
datetime.date()
Function to Convert Datetime to Date in Python - Use Pandas to Convert DateTime to Date in Python
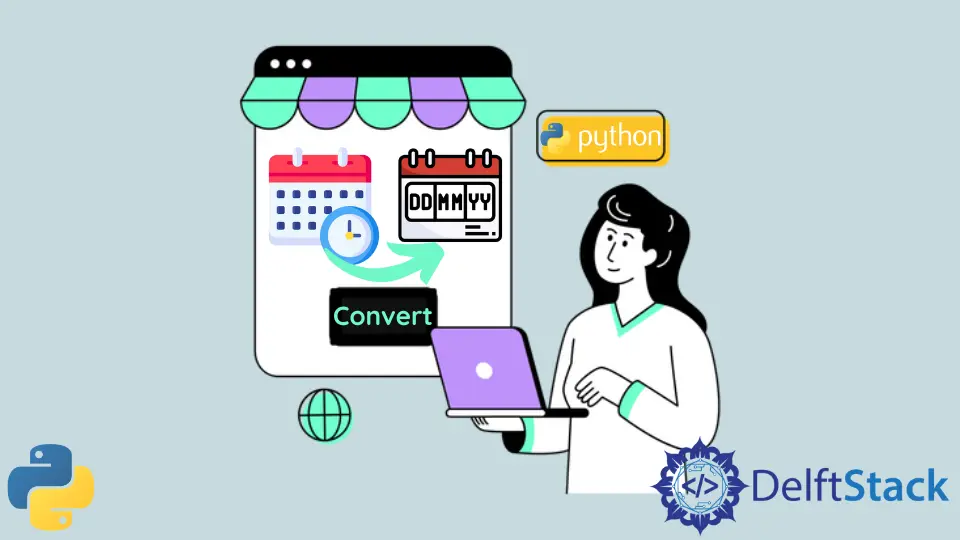
Python does not contain any specific in-built data type for storing date and time. However, it provides a datetime
module that supplies the user with classes that alter and manipulate the date and time in the Python code.
In this tutorial, we will learn the different ways available to convert DateTime to date in Python.
Before listing the different ways of implementing this process, let us understand the difference between a datetime
and a date
object.
A datetime
object is capable of returning the time and date. Usually, it contains a vast variety of values, namely the microsecond, second, minute, hour, day, month, and year values. On the other hand, an object of the type date
only contains the date.
Use the datetime.date()
Function to Convert Datetime to Date in Python
The datetime()
constructor can be utilized to generate a date.
The datetime()
constructor has three parameters: day, month, and year.
These three parameters are required to generate a date with the constructor.
The programmer can simply use the date()
function that returns an object of the date
type.
The following code uses the date()
function to convert DateTime to date in Python.
import datetime
print(datetime.datetime.now())
print(datetime.datetime.now().date())
Output:
2021-10-17 21:27:46.018327
2021-10-17
Explanation
- The
datetime
module is imported to the python code - The current date and time are provided as a
datetime
object by thedatetime.datetime.now()
function. - The current date as an object of the type
date
is supplied with the help of thedate()
function along with thedatetime.datetime.now()
function.
The datetime.date.today()
function can be utilized when there is a need to find the current date directly. However, this alternative does not work if we need anything else except the current date.
The following code uses the datetime.date.today()
function.
import datetime
print(datetime.datetime.now())
print(datetime.date.today())
Output:
2021-10-17 21:27:46.018327
2021-10-17
For this particular case, both the methods provide the same output as we are working on the current date.
Use Pandas to Convert DateTime to Date in Python
Pandas DataFrame
can be utilized to generate arrays that accommodate time and date values.
To use pandas DataFrame
and generate an array containing the time
column, we need to import the pandas
module.
The following code uses pandas DataFrame
to convert DateTime to date in Python.
import pandas as pd
dataf = pd.DataFrame(
{"EMP": [3, 6], "time": ["2021-10-15 16:05:00", "2021-10-17 20:00:30"]}
)
print(dataf)
dataf["time"] = pd.to_datetime(dataf["time"]).dt.date
print(dataf)
Output:
EMP time
0 3 2021-10-15 16:05:00
1 6 2021-10-17 20:00:30
EMP time
0 3 2021-10-15
1 6 2021-10-17
It is clearly visible in the above code that after the execution of the dt.date
function, the time column only displays the time and the date.
Explanation
- Firstly, we create a pandas
DataFrame
and add the DateTime values into a column. - Then, the
dt.date()
function is utilized to convert the DateTime value to a date value. - The
print
command is utilized to display both the date value and the DateTime value in the output.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn