How to Convert DateTime to String With Milliseconds in Python
-
Use the
strftime()
Method to Format DateTime to String -
Use the
isoformat()
Method to Format DateTime to String -
Use the
str()
Function to Format DateTime to String - Conclusion
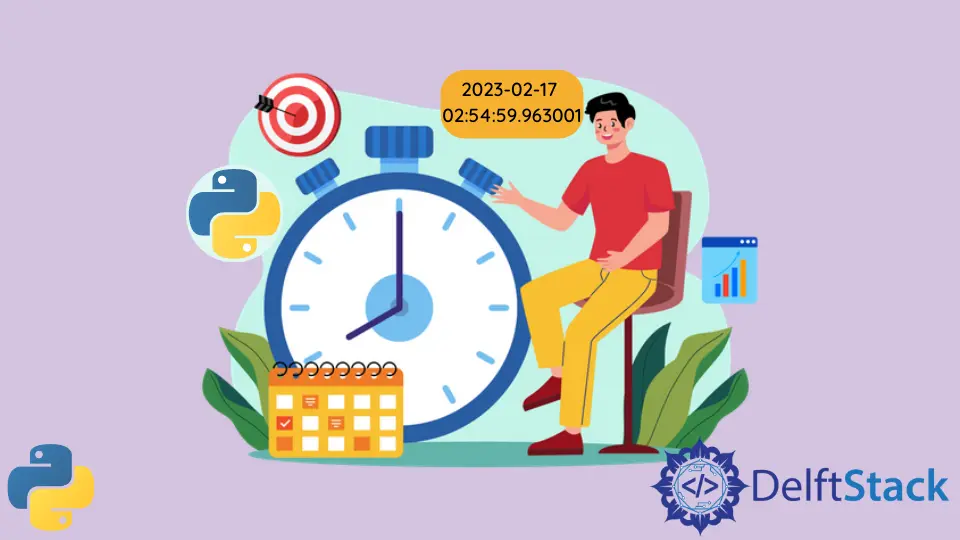
In the intricate landscape of Python programming, the manipulation of date and time is a fundamental aspect. A common challenge arises when developers need to convert a datetime
object to a string representation, a need that becomes even more crucial when precision down to milliseconds is required.
The datetime
module in Python allows us to create date and time objects easily manipulated and converted to different formats. This tutorial will cover how to convert a datetime
object to a string containing the milliseconds.
Use the strftime()
Method to Format DateTime to String
The strftime()
method returns a string based on a specific format specified as a string in the argument. In this section, we’ll delve into the concept of converting a datetime
object to a string representation with milliseconds using Python’s strftime
method with the %f
specifier.
Let’s consider a practical scenario where you need to capture the current date and time, presenting it as a string with milliseconds. Python provides a straightforward way to achieve this using the strftime
method.
from datetime import datetime
# Get current date and time
current_datetime = datetime.now()
# Convert to string with milliseconds
formatted_datetime = current_datetime.strftime("%Y-%m-%d %H:%M:%S.%f")[:-3]
print(formatted_datetime)
We start by importing the datetime
module, which contains the datetime
class. This class is essential for dealing with date and time values in Python.
Now, let’s capture the current date and time. We use the datetime.now()
method, which returns a datetime
object representing the current date and time.
On to the core of our task, the strftime
method is employed. This method allows us to format the datetime
object into a string according to a specified format.
In our case, the format string is '%Y-%m-%d %H:%M:%S.%f'
. Here, %Y
represents the year, %m
the month, %d
the day, %H
the hour, %M
the minute, %S
the second, and %f
signifies microseconds.
The resulting string includes the date and time in the specified format, with microseconds appended. To convert this to milliseconds, we slice the string using [:-3]
, excluding the last three characters.
Lastly, we print the formatted datetime string with milliseconds.
Output:
2023-12-14 15:30:45.678
import datetime
, we would have to use datetime.datetime.now()
to get the current date-time.Use the isoformat()
Method to Format DateTime to String
The second method explores the usage of Python’s isoformat
method, providing an alternative approach to converting a datetime
object to a string with milliseconds. From importing the datetime
module to creatively using string formatting with isoformat
, this section unravels the steps involved.
Consider a scenario where you need to capture the current date and time, transforming it into a string with milliseconds for further processing or display. Python provides an elegant solution using the isoformat
method.
from datetime import datetime
# Get current date and time
current_datetime = datetime.now()
# Convert to string with milliseconds using isoformat
formatted_datetime = "{}.{:03d}".format(
current_datetime.isoformat(), current_datetime.microsecond // 1000
)
print(formatted_datetime)
To begin, we import the datetime
module, which includes the datetime
class essential for handling date and time objects in Python.
Next, we capture the current date and time using datetime.now()
, creating a datetime
object named current_datetime
.
Now, let’s focus on the core of our task—the isoformat
method. This method returns a string representation of the datetime
object in the ISO 8601 format.
To include milliseconds, we utilize string formatting. The string template is composed of the ISO-formatted datetime obtained from current_datetime.isoformat()
and milliseconds derived from current_datetime.microsecond // 1000
.
The division by 1000 converts microseconds to milliseconds.
Finally, we print the formatted datetime string with milliseconds.
Output:
2023-12-14T11:48:34.693183.693
Use the str()
Function to Format DateTime to String
We can directly pass the datetime
object to the str()
function to get the string in the standard date and time format. This method is faster than the above methods, but we could specify the string format.
We can also simply remove the last three digits from the string to get the final result in milliseconds.
Consider a scenario where you need to capture the current date and time, transforming it into a string with milliseconds for further processing or display. Python provides a built-in solution using the str()
method.
from datetime import datetime
# Get current date and time
current_datetime = datetime.now()
# Convert to string with milliseconds using str()
formatted_datetime = str(current_datetime)
print(formatted_datetime)
To begin, we import the datetime
module, which contains the datetime
class necessary for working with date and time objects in Python.
Next, we capture the current date and time using datetime.now()
, creating a datetime
object named current_datetime
.
Now, let’s focus on the key aspect of our code—the str()
method. This built-in method is used to convert the datetime
object to its string representation.
The resulting string includes the date and time in a default format that includes milliseconds.
Finally, we print the formatted datetime string with milliseconds.
Output:
2023-12-14 11:54:03.419049
Conclusion
This tutorial has meticulously explored three distinct methods—strftime
with %f
specifier, isoformat
method, and the built-in str()
method—each offering unique strengths. Whether developers seek meticulous precision with %f
, adherence to the ISO 8601 standard using isoformat
, or the simplicity of str()
, these tools enrich their arsenal.
With this knowledge, Python developers can seamlessly navigate datetime intricacies, ensuring accurate representation and meeting the diverse demands of applications. Empowered by these versatile techniques, developers are poised to elevate the precision and effectiveness of their datetime handling in Python projects.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn