How to Get the Current Year in Python
-
Get the Current Year in Python With
strftime
in thedatetime
Module -
Get the Current Year in Python With
date.year
in thedatetime
Module
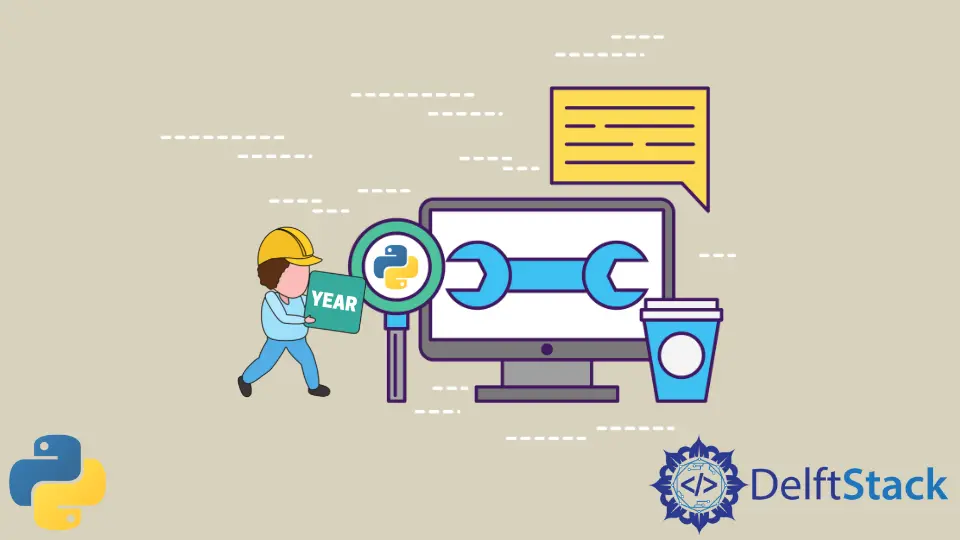
Date and time are essential metrics that we all see daily. As developers, we need to play around with date and time for several tasks such as storing birth dates and finding the difference between dates in days. This article will introduce how to get the current year in Python.
Python has many in-built libraries at our service and tons of external libraries made by developers to get things done pretty fast and with understandable and straightforward syntax. One such module is datetime
- an in-build python module to deal with date and time.
The datetime
makes it very easy to deal with dates, and it provides a simple syntax. Let’s look at some possible ways to get the current year using this module.
Get the Current Year in Python With strftime
in the datetime
Module
The datetime
module, as the name suggests, allows us to deal with both date and time. For this article, we’ll only focus on the date.
The following code demonstrates one way to print the current year.
import datetime
currentDateTime = datetime.datetime.now()
date = currentDateTime.date()
year = date.strftime("%Y")
print(f"Current Year -> {year}")
Output:
Current Year -> 2021
We first import the module and then get the current date and time using the dateime.now()
. To get the date from this result, we call the date()
function on this result and store the returned date in a variable date
. Next, we have to filter the year out from this date. For that, we use the strftime()
function, which stands for string from time
. This function takes a string argument that defines the kind of output, in the form of a string, we want from this object. In simple terms, it accepts a format in the form of a string and returns a string in the same format.
In line 5, the string format %Y
means that we want the year from this date object. The string format %A
refers to the weekday of the date object. We can also club multiple formats into a single string like this, %A %Y
. This format returns a string with a weekday and a year like Wednesday 2021
.
Lastly, we store the string into a variable year
and print it.
Refer to the official documents, here, for more possible formats.
Another way to get the current year is as follows.
import datetime
date = datetime.date.today()
year = date.strftime("%Y")
print(f"Current Year -> {year}")
Output:
Current Year -> 2021
This time we are using the date.today()
method to get today’s date. Next, we use the strftime()
function to get the year from the date and finally, print it.
Get the Current Year in Python With date.year
in the datetime
Module
If you don’t wish to use the strftime()
function, you can use the following code to get the current year.
import datetime
currentDateTime = datetime.datetime.now()
date = currentDateTime.date()
print(f"Current Year -> {date.year}")
date = datetime.date.today()
print(f"Current Year -> {date.year}")
Output:
Current Year -> 2021
Current Year -> 2021
The date()
function in line 3 returns an object of type datetime.date
. And this object has year
as an attribute. So, we can access it and print out the result. Same goes with the today()
function. It also returns an object of type datetime.date
.
You can confirm that by running the print(type(date))
statement. It will print <class 'datetime.date'>