How to Create a File if Not Exists in Python
-
Python Create File if Not Exists Using the
open()
Function -
Python Create File if Not Exists Using the
touch()
Method of thepathlib
Module -
Python Create File if Not Exists Using the
path.exists()
Method of theos
Module - Conclusion
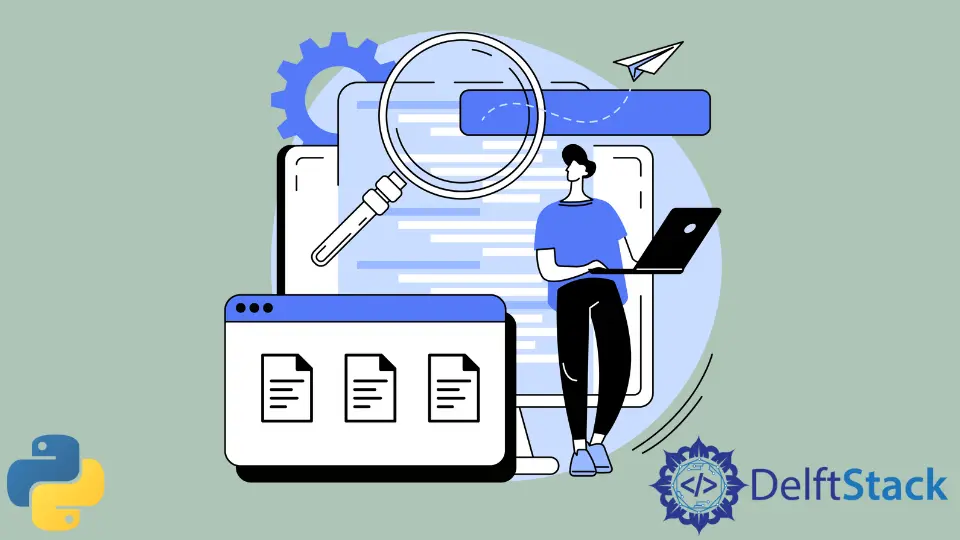
In the realm of Python programming, managing file operations with precision and safety is crucial. This article delves into three distinct methods for creating files only if they do not already exist, highlighting the open()
function with x
mode, the touch()
method from the pathlib
module, and the os.path.exists()
method from the os
module.
Each method caters to specific scenarios where data integrity and non-duplication are paramount. Whether it’s for logging, generating unique files, or handling configuration data, these methods provide Python developers with reliable tools to ensure that new files are created without the risk of overwriting existing ones.
Python Create File if Not Exists Using the open()
Function
The open()
function, when used with the x
mode, is designed to create a new file and open it for writing. The key feature of this mode is that it will fail if the file already exists, thereby preventing unintentional data overwriting.
This functionality is particularly important in scenarios where data integrity and uniqueness are crucial, such as logging events, generating unique data files, or creating configuration files.
Syntax and Parameters
The usage is straightforward:
open(file, mode="x")
file
: The path to the file (string).mode
: Here,x
is used for creating a new file. If the file already exists, the operation fails.
Example
def create_unique_file(filename):
try:
with open(filename, "x") as f:
f.write("New file created.\n")
print(f"File '{filename}' created successfully.")
except FileExistsError:
print(f"File '{filename}' already exists.")
create_unique_file("example.txt")
In our code, we define a function create_unique_file
that attempts to create a file with a given filename. We use a try-except
block to handle the possibility of the file already existing.
Inside the try
block, open(filename, 'x')
is used to create and open the file. If the file example.txt
does not exist, it is created, and a message New file created.\n
is written to it.
We then print a confirmation message to the console.
If the file already exists, an exception of type FileExistsError
is raised. This exception is caught in the except
block, and we print a message informing the user that the file already exists.
Output:
Python Create File if Not Exists Using the touch()
Method of the pathlib
Module
The Path
method is used for various file system operations, including file creation. One common use case is creating a file only if it does not already exist, which is essential to avoid overwriting existing data.
This approach is beneficial in situations like logging, where you might want to create a log file on the first run of a program and then append to it on subsequent runs.
Syntax and Parameters
The syntax for using Path
to create a file is straightforward:
from pathlib import Path
my_file = Path("/path/to/file")
if not my_file.exists():
my_file.touch()
Path("/path/to/file")
: Creates aPath
object for the specified file path.exists()
: Checks if the file at the given path exists.touch()
: Creates the file at the path represented by thePath
object.
from pathlib import Path
def create_file_if_not_exists(file_path):
file = Path(file_path)
if not file.exists():
file.touch()
print(f"File '{file_path}' created.")
else:
print(f"File '{file_path}' already exists.")
create_file_if_not_exists("example.txt")
In our function create_file_if_not_exists
, we first create a Path
object representing the file path. We then use the exists()
method to check if the file already exists.
If it does not (not file.exists()
), we use the touch()
method to create the file and print a confirmation message. If the file already exists, we print a different message to inform the user.
This approach is advantageous as it uses the more modern pathlib
module, which is part of Python’s standard library and provides a more intuitive way of handling file paths and file system operations.
Output:
Python Create File if Not Exists Using the path.exists()
Method of the os
Module
The primary purpose of using os.path.exists()
is to verify the presence of a file or directory at a given path. This function is particularly useful in scenarios where you need to ensure that creating a new file won’t overwrite an existing one, such as in data logging, temporary file creation, or when handling configuration files in an application.
Syntax and Parameters
The syntax of os.path.exists()
is simple:
import os
os.path.exists(path)
path
: A string representing the file or directory path you want to check.
import os
def create_file_if_not_exists(file_path):
if not os.path.exists(file_path):
with open(file_path, "w") as file:
file.write("This is a new file.\n")
print(f"File '{file_path}' created.")
else:
print(f"File '{file_path}' already exists.")
create_file_if_not_exists("example.txt")
In the create_file_if_not_exists
function, we first check if the file exists using os.path.exists(file_path)
.
If the file does not exist (not os.path.exists(file_path)
), we proceed to create it using a with
statement and the open
function in write mode ('w'
). This block writes a line of text to the new file and prints a confirmation message.
If the file already exists, we print a message stating that the file already exists. This ensures that we do not overwrite any existing files, thus preventing potential data loss.
Output:
Conclusion
In conclusion, the article successfully explores three robust methods in Python for creating files while ensuring they don’t already exist. These methods – using open()
with x
mode, pathlib
’s touch()
, and os.path.exists()
– are essential in a programmer’s toolkit, each serving specific requirements and use cases.
By understanding and utilizing these methods, Python programmers can elegantly handle file creation tasks in a variety of applications, from simple logging to complex data management systems. This article not only guides through the technicalities of each method but also demonstrates their practical implementation, ensuring that readers are well-equipped to apply these solutions in real-world scenarios effectively.