How to Get Frequencies of List Elements Using Python
-
Use
collections
Module to Get Frequencies of List Elements Using Python - Manual Code to Get Frequencies of List Elements Using Python
-
Use
NumPy
to Get Frequencies of List Elements Using Python
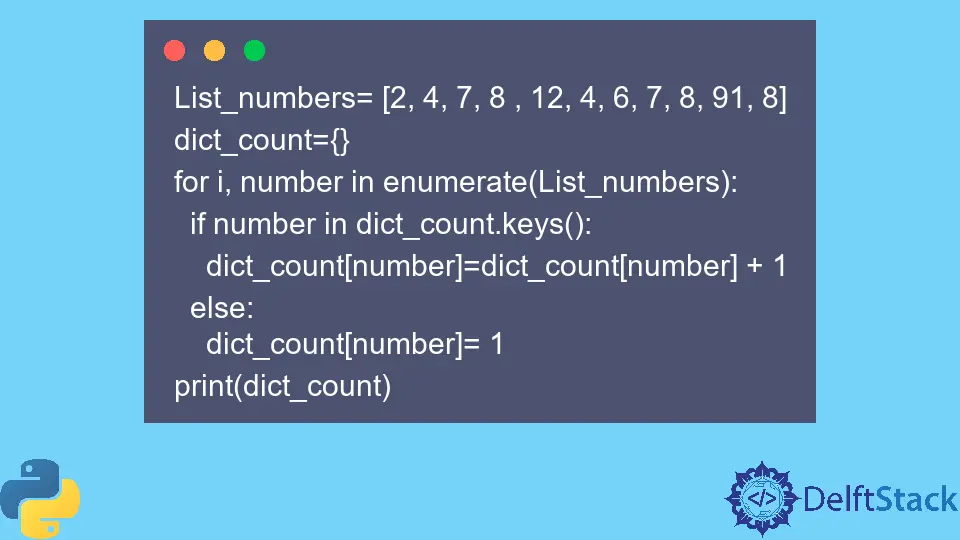
This short article will explain the possible ways to count the total occurrence of all list elements using Python. We will also see the pros and cons of doing this.
Python is a very versatile language that allows developers to focus on the key problem, not its implementation. It is comparatively easier to code in Python than in other contemporary languages like C or C++.
The item frequency problem can be solved in multiple ways through Python. First, let’s formally introduce the problem.
Consider an unordered list of numbers like List A= [2, 4, 7, 8, 12, 4, 6, 7, 8, 91, 8]
, and we want to get the frequency of each number in the list to check how many times a number appears in the list.
The answer should be like: {'2': 1, '4': 2, '6': 1, '7': 2, '8': 3, '12': 1, '91': 1 }
. It means the number 2
exists 1
time, 4
exists 2
times, 6
has a frequency of 1
, and so on.
Use collections
Module to Get Frequencies of List Elements Using Python
In this method, we will use the collections
module. The counter function of this module returns a container that keeps track of how many times a number appears in the list.
See the code below.
import collections
a = [2, 4, 7, 8, 12, 4, 6, 7, 8, 91, 8]
counter = collections.Counter(a)
print(counter)
print(type(counter))
The above code produces the following output.
Counter({8: 3, 4: 2, 7: 2, 2: 1, 12: 1, 6: 1, 91: 1})
<class 'collections.Counter'>
You can use the following code to get the unique numbers from a container.
counter.keys()
The above line of code gives the following result.
dict_keys([2, 4, 7, 8, 12, 6, 91])
Similarly, to get the count of each unique number, you can run this code:
counter.values()
The output is as follows:
dict_values([1, 2, 2, 3, 1, 1, 1])
Manual Code to Get Frequencies of List Elements Using Python
In method 1, we used the collections
module for the count. But in this method, we must do manual coding for the desired results.
The code of this approach is as follows:
List_numbers = [2, 4, 7, 8, 12, 4, 6, 7, 8, 91, 8]
dict_count = {}
for i, number in enumerate(List_numbers):
if number in dict_count.keys():
dict_count[number] = dict_count[number] + 1
else:
dict_count[number] = 1
print(dict_count)
This is a straightforward code in which we used the dictionary type to store the count against each number. A dictionary’s keys always remain unique, which means these cannot be duplicated.
The above code produces the following output.
{2: 1, 4: 2, 7: 2, 8: 3, 12: 1, 6: 1, 91: 1}
The complexity of this code is O(n)
.
Use NumPy
to Get Frequencies of List Elements Using Python
In the numpy
module, the unique()
function gives the flexibility to find the frequency of each element of the list.
import numpy as np
List_numbers = [2, 4, 7, 8, 12, 4, 6, 7, 8, 91, 8]
print(np.unique(List_numbers, return_counts=True))
The np.unique()
function is used to find the unique elements of an array. It returns the sorted unique elements of an array.
The optional parameter return_counts
allows us to get the respective element count.
The preceding code outputs:
(array([ 2, 4, 6, 7, 8, 12, 91]), array([1, 2, 1, 2, 3, 1, 1]))
To get the count, you can use:
np.unique(List_numbers, return_counts=True)[1]
In this article, we proposed different ways to get the count of list elements in Python. You can select from the discussed methods depending on the resources and time complexity.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python