Python codecs.open() Function
-
Understanding
codecs.open()
in Python -
Use Cases and Examples of Python
codecs.open()
Function -
Advantages of
codecs.open()
-
Difference Between
open()
andcodecs.open()
in Python - Conclusion
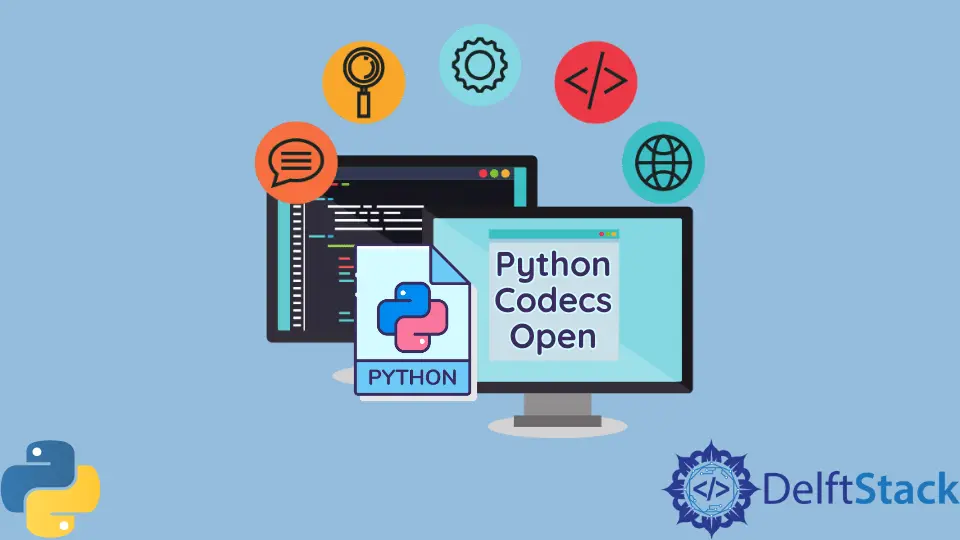
In the realm of Python programming, handling text data often involves encoding and decoding to ensure compatibility across different systems and applications. The codecs.open()
function is a powerful tool provided by the codecs
module, offering a versatile and comprehensive approach to working with various text encodings.
This article aims to provide an in-depth exploration of the codecs.open()
function in Python, covering its functionality, use cases, and practical examples.
Understanding codecs.open()
in Python
The codecs.open()
function is a part of the codecs
module, which is designed to handle various character encodings in Python. This function is specifically tailored for reading and writing text files with different encodings, offering more flexibility and control than the built-in open()
function.
The codecs.open()
function works in parallel with the in-built open()
function in Python and opens up files with a specific encoding. By default, it opens a file in the read mode.
The codecs.open()
function opens all files in binary mode, even if it isn’t manually mentioned in the syntax of the code. This avoids data loss that may occur when dealing with 8-bit encoding.
Syntax of Python codecs.open()
Function
The syntax for codecs.open()
is similar to the built-in open()
function, with additional parameters for specifying the encoding and error handling.
codecs.open(filename, mode="r", encoding=None, errors="strict", buffering=1)
filename
: The name of the file to be opened.mode
: The mode in which the file is opened ('r'
for reading,'w'
for writing, etc.).encoding
: The character encoding to be used. If set toNone
, the system default encoding is used.errors
: Specifies how encoding errors are handled. It can take values such as'strict'
,'ignore'
,'replace'
, etc.buffering
: An optional integer that sets the buffering policy. The default is1
(line buffering).
The arguments in the syntax of the function depicted above contain their default values.
Key Parameters:
encoding
Parameter:- If specified, this parameter determines the character encoding used to interpret the file’s contents.
- Common encodings include
'utf-8'
,'latin-1'
,'ascii'
, etc. - If set to
None
, the system default encoding is used.
errors
Parameter:- Specifies how encoding errors are handled during file operations.
- Options include
'strict'
(raiseUnicodeError
),'ignore'
(ignore errors),'replace'
(replace with a suitable replacement character), and more.
The codecs.open()
function became obsolete after version 2.6 of Python was released. Python added another io.open()
function that was utilized to enhance the in-built open()
function’s capabilities.
The syntax of the io.open()
function, which is mostly compared to the codecs.open()
function, is relatively different from the codecs.open()
function, which is as follows.
io.open(
file,
mode="r",
buffering=-1,
encoding=None,
errors=None,
newline=None,
closefd=True,
opener=None,
)
The codecs.open()
function, although still existing in the newer versions, has no real value and is mostly utilized for backward compatibility.
Use Cases and Examples of Python codecs.open()
Function
Reading a File
import codecs
# Open a file for reading with UTF-8 encoding
with codecs.open("example.txt", "r", encoding="utf-8") as file:
content = file.read()
print(content)
In this example, the codecs.open()
function is used to open a file named 'example.txt'
in read mode with UTF-8 encoding. The content of the file is then read and printed.
Writing to a File
import codecs
# Open a file for writing with Latin-1 encoding
with codecs.open("output.txt", "w", encoding="latin-1") as file:
file.write("Hello, Latin-1!")
print("File 'output.txt' has been written.")
Output in the console:
File 'output.txt' has been written.
output.txt
file:
Hello, Latin-1!
In this example, the file named 'output.txt'
is opened in write mode with 'latin-1'
encoding. The string "Hello, Latin-1!"
is then written to the file.
The 'latin-1'
encoding supports a wide range of characters and is suitable for scenarios where Unicode is not required.
Handling Encoding Errors
import codecs
# Open a file with Latin-1 encoding, ignoring errors
with codecs.open("data.txt", "r", encoding="latin-1", errors="ignore") as file:
content = file.read()
print(content)
Here, the codecs.open()
function opens a file with Latin-1 encoding and specifies to ignore encoding errors. This can be useful when dealing with files that may contain characters not compatible with the chosen encoding.
Advantages of codecs.open()
- Explicit Encoding Specification:
- The ability to explicitly specify the encoding ensures that developers have control over how the file is interpreted.
- Robust Error Handling:
- The
errors
parameter provides options for handling encoding errors, allowing developers to choose between raising errors, ignoring errors, or replacing problematic characters.
- The
- Support for Multiple Encodings:
- The
codecs
module supports a wide range of encodings, making it versatile for handling text data in various formats.
- The
- Consistency Across Platforms:
- By explicitly specifying the encoding, developers can ensure consistent behavior across different platforms and avoid potential issues related to system default encodings.
Difference Between open()
and codecs.open()
in Python
In Python, both the open
function and the codecs.open
function are used for file I/O operations. However, there are some differences between the two, particularly in their handling of character encodings.
open
Function in Python
- The built-in
open
function is used to open a file and return a file object. - It supports a limited set of character encodings, primarily focusing on ASCII and UTF-8.
- By default, it opens files in text mode (
't'
), which means it performs newline translation and returns strings. - Binary mode (
'b'
) can be specified to handle non-text files, such as images or executables.
Example:
with open("example.txt", "r", encoding="utf-8") as file:
content = file.read()
codecs.open
Function in Python
- The
codecs.open
function is part of thecodecs
module, which provides additional support for character encodings. - It extends the capabilities of the
open
function by allowing the specification of a wider range of encodings. - This function is especially useful when dealing with non-standard encodings or legacy systems.
Example:
import codecs
with codecs.open("example.txt", "r", encoding="latin-1") as file:
content = file.read()
Key Differences:
- Character Encoding:
- The primary difference lies in the handling of character encodings. While the
open
function supports a limited set of encodings,codecs.open
provides a broader range of encoding options.
- The primary difference lies in the handling of character encodings. While the
- Text Mode:
- The
open
function defaults to text mode ('t'
), which performs newline translation and returns strings. In contrast,codecs.open
doesn’t automatically perform newline translation.
- The
- Unicode Support:
codecs.open
has better support for Unicode encodings and legacy character sets.
- Compatibility:
- The
codecs
module is designed to provide additional functionality beyond what is available in the built-inopen
function. It is more suitable for cases where specialized encoding handling is required.
- The
When to Use Each:
- Use the built-in
open
function when working with standard encodings like UTF-8 and ASCII. - If you need to work with a broader range of encodings, especially non-standard or legacy encodings, or if you need more control over encoding-related aspects, then
codecs.open
is a better choice.
In general, for most everyday use cases, the built-in open
function is sufficient. Use codecs.open
when you encounter specific encoding challenges that the standard open
function cannot handle adequately.
Conclusion
The codecs.open()
function in Python’s codecs
module is a powerful tool for handling text files with different encodings. Its ability to explicitly specify encoding, handle errors, and support a variety of encodings makes it a valuable asset for working with diverse datasets.
Whether reading or writing files, the codecs.open()
function provides the flexibility and control needed to ensure accurate interpretation and manipulation of text data in Python. Understanding its capabilities empowers developers to handle text encodings effectively, promoting robust and interoperable code.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn