How to Clear the Contents of a File in Python
-
Use the
truncate()
Function to Clear the Contents of a File in Python -
Use the
w
Mode to Clear the Contents of a File in Python -
Use the
os.truncate()
Function to Clear the Contents of a File in Python -
Use the
shutil.copyfileobj()
Function to Clear the Contents of a File in Python -
Use
io.StringIO()
to Clear the Contents of a File in Python - Conclusion
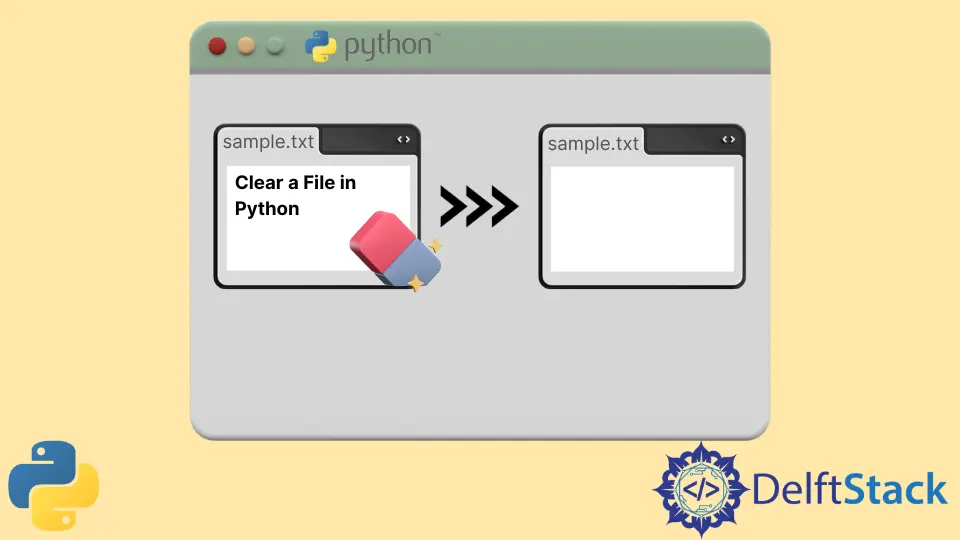
Files are an integral part of programming, serving as a means of storing and retrieving data. There are situations where you may need to clear a file, removing all existing data to start with a clean slate.
Clearing a file in Python involves removing its content, leaving it empty or ready for new data. This can be essential in various scenarios, such as logging or preparing a file for new information.
In this tutorial, we will introduce how to clear a file in Python.
Use the truncate()
Function to Clear the Contents of a File in Python
The truncate()
method in Python file handling allows us to set the size of the current file to a specific number of bytes. We can pass the desired size to the function as arguments.
Syntax:
file.truncate(size=None)
If size
is not provided, it defaults to the current position of the file pointer. When size
is specified, the file is truncated to that size.
truncate()
Function With Arguments
To clear the contents of a file up to a specific point, you can provide a size
argument to truncate()
. This will cut off the file at the specified size.
We need to open a file in append or read mode to truncate it. The code below resizes the sample file to 4 bytes.
with open("sample.txt", "r+") as f:
f.truncate(4)
truncate()
Function With No Arguments
When called without arguments, truncate()
cuts off all data from the current position of the file pointer to the end of the file. This effectively clears the contents of the file beyond the current position.
To clear all the contents of a file, we pass 0
to the function, as shown below.
with open("sample.txt", "r+") as f:
f.truncate(0)
This method is handy for reading a file and removing its contents afterward. Also, note that if we need to write to this file after erasing its elements, add f.seek(0)
to move to the beginning of the file after the truncate()
function.
Use the w
Mode to Clear the Contents of a File in Python
The 'w'
mode in file handling is a powerful tool that allows you to open a file for writing. If the file already exists, it will truncate (clear) its contents. If the file does not exist, it will create a new file.
Syntax:
with open('example.txt', 'w') as file:
# Perform write operations
with
: Ensures that the file is properly closed after operations are complete. This helps prevent resource leaks and ensures safe file handling.open('example.txt', 'w')
: Opens a file namedexample.txt
in write mode ('w'
).as file
: Assigns the opened file object to the variablefile
. This variable will be used to interact with the file within the context of thewith
block.
The simplest method to clear a file in Python is by opening it in write mode ('w'
). This will truncate the file, removing its content and preparing it for new data.
Example 1:
with open("sample.txt", "w") as f:
pass
When we open the file in write mode, it automatically clears all the file content. The pass
keyword here specifies that there is no operation executed.
Another method of achieving this is shown below.
Example 2:
f = open("sample.txt", "w")
f.close()
Both code snippets achieve the same result in this specific case, which is opening a file in write mode and immediately closing it.
The first code snippet uses the with
statement, which is considered more Pythonic and is often used for file handling. It ensures that the file is closed even if an error occurs during the execution of the code inside the with
block.
The second code snippet achieves the same result, but it explicitly calls close()
on the file object. While this is perfectly valid, it requires an extra line of code.
Use the os.truncate()
Function to Clear the Contents of a File in Python
The os.truncate()
function is used to resize a file to a specified size. This function is particularly useful for clearing the contents of a file by effectively cutting down or removing all its data.
In the syntax below, os.truncate()
works with file paths directly, allowing you to specify the file using its path name.
Syntax:
os.truncate(path, length)
path
: This is a string representing the file path (including the file name) of the file you want to truncate.length
: This is the new size you want the file to be. Any data beyond this size will be removed. Iflength
is greater than the current file size, the file will be extended and filled with null bytes.
Here’s an example of using the os.truncate()
function to set the size of the file named 'my_file.txt'
to 0 bytes, effectively clearing its contents without explicitly opening it.
import os
os.truncate("my_file.txt", 0)
This code snippet is particularly useful when you want to clear the contents of a file without completely deleting or recreating the file. It’s a quick and efficient way to start with an empty file while keeping the file itself intact.
Use the shutil.copyfileobj()
Function to Clear the Contents of a File in Python
The shutil.copyfileobj()
function is primarily used for copying the contents of one file-like object (such as a file, socket, or stream) to another. However, by strategically using this function, we can effectively clear the contents of a file.
Syntax:
shutil.copyfileobj(src, dst, length=16 * 1024)
src
: This is the source file-like object that you want to copy from.dst
: This is the destination file-like object that you want to copy to.length
(optional): This parameter specifies the buffer size used for copying data. The default value is 16KB.
With an understanding of the shutil.copyfileobj()
function, we can leverage it to clear the contents of a file effectively. The code below involves using shutil.copyfileobj()
to copy an empty file-like object to the target file.
import shutil
import io
with open("my_file.txt", "w") as file:
shutil.copyfileobj(io.StringIO(""), file)
In this code, we first import the shutil
and io
modules. Then we use io.StringIO("")
to create an empty in-memory file-like object.
The shutil.copyfileobj()
is then used to copy the content of the empty object to my_file.txt
, effectively clearing it.
Use io.StringIO()
to Clear the Contents of a File in Python
io.StringIO()
is a class in the Python io
module that provides an in-memory file-like object. It allows you to treat strings as file-like objects, enabling you to perform file operations without the need for actual files on the disk. By leveraging io.StringIO()
, we can efficiently clear the contents of a file.
The basic syntax for creating an io.StringIO()
object is as follows:
io.StringIO(initial_value="", newline="\n")
initial_value
(optional): This is an optional string that can be used to initialize theio.StringIO()
object with some initial content.newline
(optional): This parameter specifies the newline character used in the file-like object. The default is'\n'
.
If you’re working with data in memory, you can use io.StringIO()
to simulate file-like operations. You can clear the content by seeking the beginning and truncating.
The code below demonstrates the io.StringIO()
creating an in-memory file-like object and writing some content to it. The seek(0)
moves the cursor to the beginning of the file, and the truncate(0)
clears the content.
import io
# Create an instance of io.StringIO
data = io.StringIO()
# Perform operations on the data
data.write("Hello, world!")
# Clear the contents of the data
data.seek(0)
data.truncate(0)
# Retrieve the cleared content
cleared_content = data.getvalue()
In this example, we first create an instance of io.StringIO()
named data
. We then perform the write operations on the data.
To clear its contents, we use the truncate()
method with an argument of 0
, which sets the size to zero. Finally, we retrieve the cleared content using data.getvalue()
.
Conclusion
In conclusion, this tutorial has covered various methods for clearing the contents of a file in Python.
We explored the use of the truncate()
function, the 'w'
mode when opening files, the os.truncate()
function, and the shutil.copyfileobj()
function. Additionally, we introduced io.StringIO()
as a powerful tool for simulating file-like operations in memory.
Each method has its use cases and advantages that allow you to choose the most suitable approach based on your specific requirements. Whether you need to clear a file for logging, preparing it for new data, or any other purpose, these techniques provide flexible solutions for efficient file handling in Python.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn