Optional Arguments for a Class Constructor in Python
- Understanding Python Arguments
- Python Optional Arguments Explained
- Real-World Scenarios
- Set Optional Arguments for a Class Constructor in Python
- Multiple Optional Arguments in the Python Class
- Comparing Optional and Positional Arguments
- Advanced Optional Python Class Argument Handling
- Common Mistakes and Best Practices of Python Class Optional Arguments
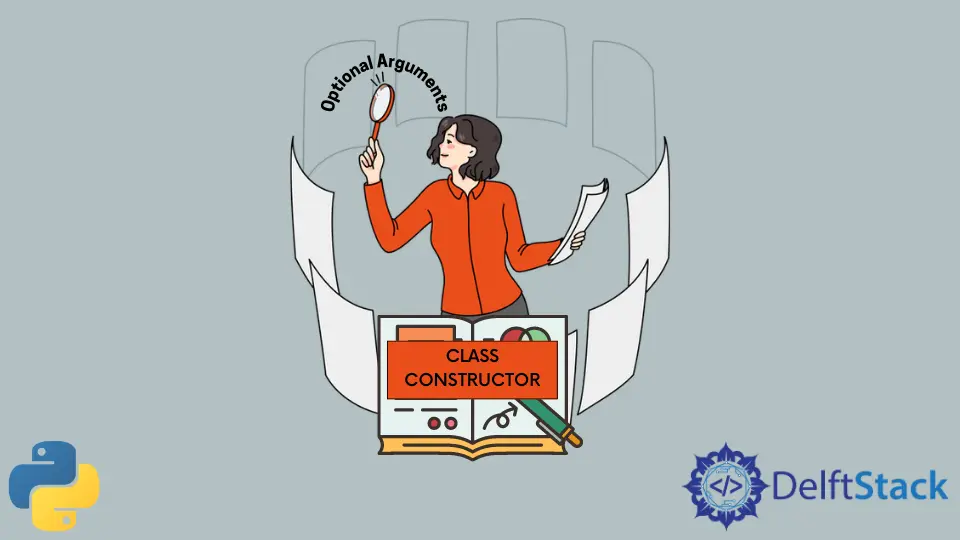
As the name suggests, an optional argument is an argument for which passing a value is not compulsory. For such an argument, a default value is assessed. If some value is passed for such an argument, the new value overwrites the default value.
Optional arguments provide a way to make certain parameters of a function or method optional. They can greatly improve the readability and usability of your code, as users can choose whether or not to provide values for these parameters.
Instead of forcing users to provide values for every parameter, you can set default values that are used when no value is explicitly passed.
In this article, we will learn how to set optional arguments for classes in Python.
Understanding Python Arguments
Before diving into optional arguments, let’s revisit the essence of Python arguments. In essence, an argument is a value supplied to a function, acting as a placeholder for data that the function will manipulate. For instance, consider the function below:
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
Here, name
is an argument that accepts the value Alice
when calling the function. Arguments facilitate the customization of function behavior based on external input.
Python Optional Arguments Explained
Optional arguments transcend the rigidity of mandatory arguments by allowing you to provide default values. These values come into play when an argument is not explicitly passed during function invocation. The beauty lies in the flexibility it introduces. Defining an optional argument is as simple as assigning a default value in the function’s parameter list:
def exponent(base, power=2):
return base ** power
print(exponent(3)) # Output: 9
print(exponent(2, 4)) # Output: 16
In the exponent
function, power
is an optional argument with a default value of 2. This enables you to calculate the square of a number conveniently.
Real-World Scenarios
Let’s delve into practical applications of optional arguments. Consider a customer order tracking function that needs to display shipping status. You can leverage optional arguments to make certain fields optional, like the shipping carrier:
def track_order(order_id, carrier=None):
if carrier:
print(f"Order {order_id} is shipped via {carrier}.")
else:
print(f"Order {order_id} is in transit.")
track_order(123) # Output: Order 123 is in transit.
track_order(456, carrier="UPS") # Output: Order 456 is shipped via UPS.
By utilizing the optional carrier
argument, the function maintains simplicity while accommodating different scenarios.
Set Optional Arguments for a Class Constructor in Python
To add optional arguments to classes in Python, we have to assign some default values to the arguments in the class’s constructor function signature. Adding default values is a straightforward task. We have to equate arguments to their default values like x = 3
, name = "Untitled"
, etc. The default value will be considered if no values are passed for these optional arguments. To following Python code depicts the concept discussed above.
class Point:
def __init__(self, x, y, z=0):
self.x = x
self.y = y
self.z = z
def __str__(self):
return f"X: {self.x}, Y: {self.y}, Z:{self.z}"
print(Point(1, 2)) # Object 1
print(Point(54, 92, 0)) # Object 2
print(Point(99, 26, 100)) # Object 3
Output:
X: 1, Y: 2, Z:0
X: 54, Y: 92, Z:0
X: 99, Y: 26, Z:100
The class Point
constructor accepts three arguments: x
, y
, and z
. Here z
is the optional argument because it has a default value set for it. This makes the other two arguments, x
and y
, compulsory. For Object 1
, no value was passed for the z
argument, and from the output, we can see that the default value was considered for z
. And, for Object 3, 100
was passed for the z
argument, and from the output, we can see that 100
was considered over the default 0
value.
Multiple Optional Arguments in the Python Class
You can set multiple optional arguments by defining default values for them in the class constructor’s signature. Let’s use a Rectangle
class example to include both length and width as mandatory attributes, and color as an optional attribute with a default value:
class Rectangle:
def __init__(self, length, width, color="white"):
self.length = length
self.width = width
self.color = color
def display_info(self):
return f"Length: {self.length}, Width: {self.width}, Color: {self.color}"
Now, users can create instances of the Rectangle
class with or without specifying a color:
rectangle1 = Rectangle(10, 5)
rectangle2 = Rectangle(8, 8, "blue")
print(rectangle1.display_info())
print(rectangle2.display_info())
Output:
Length: 10, Width: 5, Color: white
Length: 8, Width: 8, Color: blue
Comparing Optional and Positional Arguments
Understanding the distinction between optional and positional arguments is crucial. Positional arguments are determined by their position in the function call, while optional arguments are identified by their names. This enables you to call functions with more clarity and control:
def display_info(name, age, city="Unknown"):
print(f"{name} is {age} years old and lives in {city}.")
# Output: Alice is 28 years old and lives in Unknown.
display_info("Alice", 28)
display_info("Bob", 35, "Seattle") # Output: Bob is 35 years old and lives
Advanced Optional Python Class Argument Handling
For more advanced scenarios, you can utilize dictionary unpacking (**kwargs
) to handle a dynamic number of optional arguments. This technique allows users to provide any number of keyword arguments with values, which can then be processed within your constructor.
Here’s an example demonstrating the use of dictionary unpacking for advanced optional argument handling:
class AdvancedRectangle:
def __init__(self, length, width, **kwargs):
self.length = length
self.width = width
self.color = kwargs.get("color", "white")
self.border = kwargs.get("border", False)
def display_info(self):
return f"Length: {self.length}, Width: {self.width}, Color: {self.color}, Border: {self.border}"
In this example, the AdvancedRectangle
class accepts length
and width
as mandatory arguments. However, it can also accept additional optional arguments using dictionary unpacking. Users can pass any number of keyword arguments, such as color
and border
, to customize the instance:
rectangle3 = AdvancedRectangle(12, 6, color="green")
rectangle4 = AdvancedRectangle(15, 10, color="red", border=True)
print(rectangle3.display_info())
print(rectangle4.display_info())
Output:
Length: 12, Width: 6, Color: green, Border: False
Length: 15, Width: 10, Color: red, Border: True
The display_info()
method now includes information about the optional border
attribute.
Common Mistakes and Best Practices of Python Class Optional Arguments
Even though optional arguments are powerful, they can lead to subtle mistakes if not used carefully. Let’s explore common pitfalls and best practices to ensure your code remains clean and maintainable.
Placing Optional Arguments Before Required Ones
One of the most common mistakes is misplacing optional arguments before required ones in a function’s parameter list. This violates Python’s syntax rules and can lead to unexpected behavior. Required arguments are positional, meaning they must be provided in the order defined by the function signature.
Mistake:
def calculate_total(discount=0.1, price):
return price * (1 - discount)
# This will result in a syntax error
Best Practice: Always place optional arguments after required ones to maintain code clarity and prevent errors.
Unclear Documentation of Default Values
When using optional arguments, it’s crucial to document their default values and their intended behavior. Clear documentation helps other developers understand how your function behaves without needing to dive into the implementation details.
Mistake:
def send_email(subject, body, priority="low"):
"""
Sends an email with the specified subject, body, and priority.
Parameters:
subject (str): The subject of the email.
body (str): The body of the email.
priority (str): The priority of the email. Default is "low".
"""
# Implementation details
Best Practice: Clearly document default values and their intended use in your function’s docstrings. This makes it easier for others (and your future self) to understand your function’s behavior without examining the code.
Using Mutable Objects as Default Values
Using mutable objects like lists or dictionaries as default values for optional arguments can lead to unexpected behavior. Since mutable objects are shared between function calls, modifications made to them can have unintended consequences.
Mistake:
def add_item(item, items=[]):
items.append(item)
return items
print(add_item("apple")) # Output: ['apple']
print(add_item("banana")) # Output: ['apple', 'banana']
Best Practice: Use immutable objects (like None
) as default values for mutable optional arguments, and create the mutable object inside the function if needed.
Providing Values for Optional Arguments Unnecessarily
Sometimes, developers provide values for optional arguments even when the default value suffices. This can clutter function calls and make the code harder to read.
Mistake:
print(
calculate_total(discount=0.2, price=50)
) # Providing unnecessary value for 'discount'
Best Practice: Only provide values for optional arguments when you want to override the default behavior. Omit unnecessary argument assignments for cleaner code.
By avoiding these common mistakes and following best practices, you can harness the power of optional arguments effectively and maintain a clean, readable codebase.