How to Check if a Value Is in a Dictionary in Python
-
Use
get()
and Key to Check if Value Exists in a Dictionary -
Use
values()
to Check if Specified Value Exists in a Dictionary - Check if Value Exists in a Dictionary if the Value Is a List
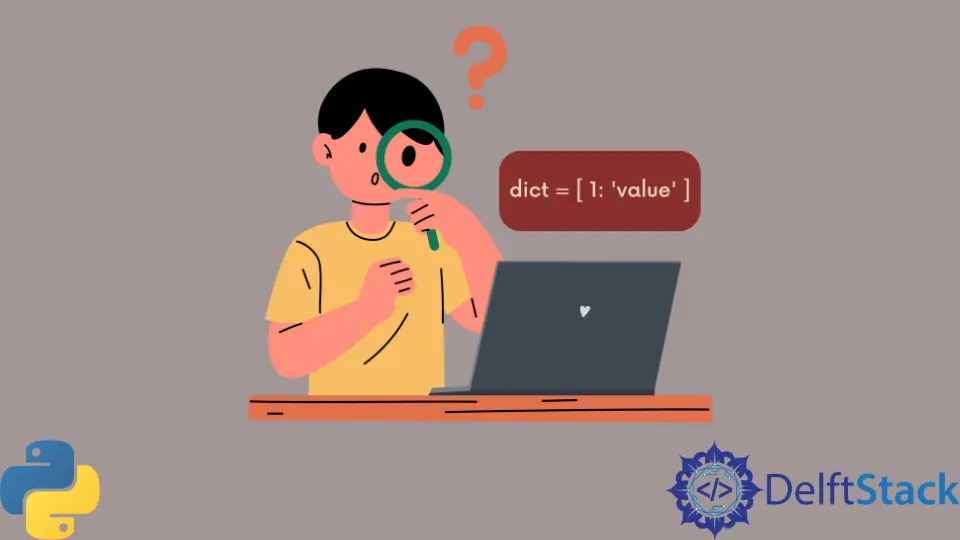
This tutorial shows how you can check a value if it exists within a Python dictionary. Here, we’ll cover relevant topics, such as searching for a value given by a key, searching for a specific value, and searching for a value that’s an object or a collection.
To start, you’d want to print True
if the value exists in the dictionary; otherwise, print False
.
Use get()
and Key to Check if Value Exists in a Dictionary
Dictionaries in Python have a built-in function key()
, which returns the value of the given key. At the same time, it would return None
if it doesn’t exist. You can use this function as a condition to determine whether or not a value exists within a dictionary.
Let’s first declare a simple dictionary with int
keys and string values.
simpDict = {1: "Ant", 2: "Bear", 3: "Cat", 4: "Dog", 5: "Elephant"}
If you want to look for the value using its key pair, then the key()
function is the way to go. For example, you want to search for any value with the 6 as their key; follow the code below.
if simpDict.key(6) != None:
print("True")
else:
print("False")
Since the simpDict
function doesn’t have the key 6, this block of code will output False
.
Use values()
to Check if Specified Value Exists in a Dictionary
Unlike the situation in looking for a specific value, looking for a key within a dictionary is very straightforward; you just have to use the keyword in
and the key you’re looking for in the dictionary.
print(3 in simpDict)
This will output True
since key 3 exists. If we replace the number with a non-existent key, then it will output False
.
On the other hand, you can use the function values()
if you want to look for a specific value in the dictionary. The values()
command will return a list of all the values within a dictionary. Using values()
, you can utilize the in
keyword to the dictionary and the specified value. Let’s say you want to know if the value Elephant
is in the dictionary, execute the following code:
print("Elephant" in simpDict.values())
This line will output True
since the word Elephant
exists in simpDict
on key 5.
In Python 2.X, there also are two other functions similar to values()
, they are the itervalues()
and viewvalues()
; these functions have been deprecated in the Python 3.X versions.
The itervalues()
and viewvalues()
commands will do the same task as the values()
command; however, different implementations, although very negligible, greatly affects the runtime.
print("Elephant" in simpDict.itervalues())
print("Elephant" in simpDict.viewvalues())
Both will return the same output as the values()
function. It’s important to note that redundancy is probably the primary reason why these functions have been deprecated from newer Python versions.
Now, what if the values of a dictionary are data structures like a list or an object? Let’s find out.
Check if Value Exists in a Dictionary if the Value Is a List
listDict = {1: ["a", "b"], 2: ["b", "c"], 3: ["c", "d"], 4: ["d", "e"]}
Now, we have a dictionary with int
keys and a list of characters as values.
Let’s say we want to search if the list ['c', 'd']
exists in the dictionary.
print(["c", "d"] in listDict.values())
The resulting output would be True
; this confirms that comparing collections by iterating the dictionary also works.
But what if you want to check if a value within a list value exists in the dictionary? To solve this, just loop over the values and use the in
keyword on the list, instead of on the dictionary.
For example, you want to check whether the character 'e'
exists within the values in this list or not. Here’s the code to execute for that:
tof = False
for value in listDict.values():
if "e" in value:
tof = True
break
print(tof)
The output will print out True
since 'e'
exists in the dictionary.
In summary, use the function values()
to iterate over the values and compare whether the value you’re looking for exists within the list of values. This helps when you want to check if a specified value exists in a dictionary.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn