How to Print Bold Text in Python
- Use the ANSI Escape Sequence Method to Print Bold Text in Python
- Use a Class to Print Bold Text in Python
-
Use the
termcolor
Module to Print Bold Text in Python -
Use the
colorama
Package to Print Bold Text in Python -
Use the
simple-color
Package to Print Bold Text in Python -
Use the
click
Library to Print Bold Text in Python - Conclusion
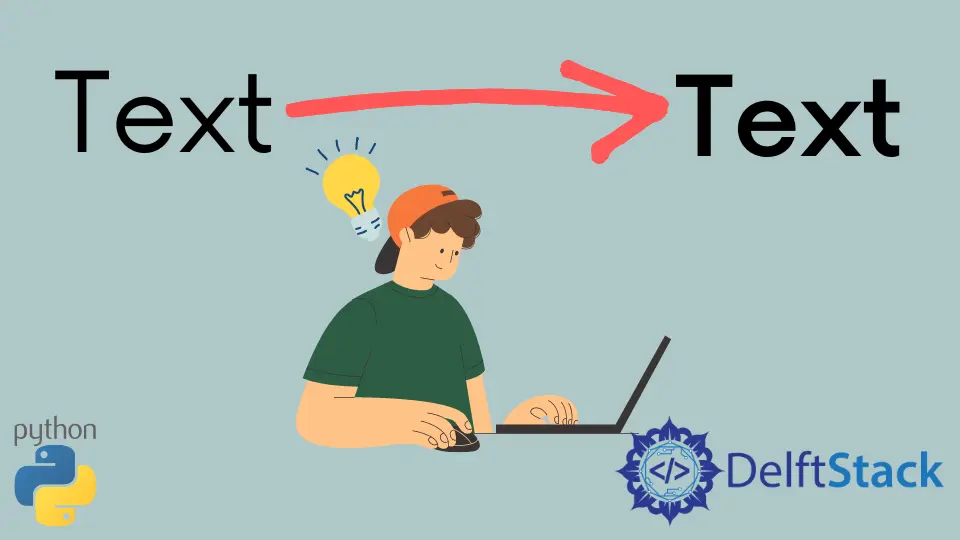
This article will discuss some methods to print bold text in Python.
Use the ANSI Escape Sequence Method to Print Bold Text in Python
We can use built-in ANSI escape sequences to make text bold. By using the special ANSI escape sequences, the text can be printed in different formats.
The ANSI escape sequence to print bold text is \033[1m
.
Example Code:
print("The bold text is", "\033[1m" + "Python" + "\033[0m")
Output:
In this code, we are using ANSI escape sequences to print bold text in Python. The string "The bold text is"
is followed by the expression "\033[1m" + "Python" + "\033[0m"
.
The \033[1m
represents the ANSI escape sequence for bold text, and \033[0m
serves to reset the formatting. The concatenation of these sequences with the word "Python"
ensures that only the word "Python"
is displayed in bold.
Upon executing the code, the output will be a statement with the specified prefix followed by the bold word Python
. Here, '\033[0m'
ends the bold formatting, and if it’s not added, the next print statement will keep printing the bold text.
Use a Class to Print Bold Text in Python
This method creates a bold_text
class. ANSI escape sequence for making text bold is listed in the class.
We can extract the ANSI escape sequences into a reusable class.
Example Code:
class bold_text:
BOLD = "\033[1m"
print(bold_text.BOLD + "Python Programming!")
Output:
In this code, we have defined a class named bold_text
, which contains a class attribute BOLD
assigned the ANSI escape sequence \033[1m
. This escape sequence represents bold text formatting.
Subsequently, we use this attribute by accessing it with the class name and concatenate it with the string "Python Programming!"
inside the print statement. As a result, when we execute this code, the output will be the specified text Python Programming!
displayed in bold.
The ANSI escape sequence ensures that the bold formatting is applied exclusively to the text within the print statement, offering a visually emphasized representation.
Use the termcolor
Module to Print Bold Text in Python
The termcolor
is a package for ANSI color formatting for output in the terminal with different properties for different terminals and certain text properties. We will use bold text attributes in this function, and the colored()
function gives the text a specific color and makes it bold.
To use this package, you must install it in your terminal using the following command. If you have not installed it, then the code will not work properly.
pip install termcolor
Example Code:
from termcolor import colored
print(colored("python", "green", attrs=["bold"]))
Output:
In this code, we are utilizing the colored
function from the termcolor
module to print stylized text in the terminal. We pass the string "python"
as the text to be colored, specify green
as the color, and include the attribute bold
in the attrs
parameter to make the text bold.
Upon executing the code, the output will be the word python
displayed in the terminal, styled in green and rendered in bold. The colored
function streamlines the process of applying both color and style attributes, offering a convenient way to enhance the visual appearance of text output in the terminal environment.
Use the colorama
Package to Print Bold Text in Python
The colorama
is a Python package that is a cross-platform for colored terminal text. It makes ANSI work under MS Windows for escape character sequences.
To use this package, you must install it in your terminal using the following command. If you have not installed it, then the code will not work properly.
pip install colorama
Example Code:
from colorama import init, Style
# Initialize colorama (required on Windows)
init()
print(f"{Style.BRIGHT}Bold Text{Style.RESET_ALL}")
Output:
In this code, we first import the necessary modules from the colorama
package, specifically the init
and Style
components. The init
function is then invoked to initialize colorama
, a step required for proper functionality on Windows systems.
Following this initialization, we use an f-string
within the print statement to output the text "Bold Text"
. The expression {Style.BRIGHT}
applies the ANSI escape sequence for bold text, ensuring that the subsequent text appears in a bold style.
The {Style.RESET_ALL}
expression resets the text style to its default after printing the bold text. When executed, this code will display Bold Text
in the terminal, with the specified text formatted in bold.
The combination of colorama
and ANSI escape sequences simplifies the process of enhancing text styling for improved visual presentation.
Use the simple-color
Package to Print Bold Text in Python
The simple-colors
package in Python is a utility that allows you to print colored and styled text to the terminal. We can use the simple-colors
package to print bold text in Python.
First, we need to install the package by running the following command. If you have not installed it, then the code will not work properly.
pip install simple_colors
Example Code:
from simple_colors import *
print(green("Python", "bold"))
Output:
In this code, we utilize the simple_colors
package to print styled text in the terminal. By importing the *
wildcard, we gain access to all color and style functions provided by the package.
The green
function is then employed to print the word "Python"
in green text. The second parameter, "bold"
, specifies that the text should be displayed in a bold style. When we compile this code, the output will be the word Python
presented in the terminal, highlighted in green and rendered in bold.
The simplicity of the simple_colors
package streamlines the process of applying both color and style attributes, offering a straightforward way to enhance the visual appearance of text output in the terminal environment.
Use the click
Library to Print Bold Text in Python
The click
library in Python provides a function called click.style()
that can be used to print bold text. The click.style()
function takes two arguments: the text to be styled and a dictionary of styles to apply.
The styles dictionary can include the bold
key to make the text bold.
If the code will not work on the first compile, then you need to install a package using the following command.
pip install click
Example Code:
import click
click.echo(click.style("Bold Text", bold=True))
Output:
In this code, we use the click
library to print bold text in the terminal. We begin by importing the click
module.
The click.style
function is then utilized, taking two arguments: the text to be styled ("Bold Text"
) and a dictionary specifying the style attributes. The attribute bold=True
is included in the dictionary, indicating that the text should be displayed in a bold style.
Upon execution of this code, the output will be the phrase Bold Text
presented in the terminal, with the specified text rendered in bold. The click
library provides a convenient way to enhance text styling, offering a clear and concise method for developers to incorporate bold formatting into their terminal output.
Remember that these methods mentioned in this article might not work in all environments, especially those that do not support ANSI escape sequences.
Conclusion
This article illuminates several avenues for implementing bold text in Python, catering to the diverse needs and preferences of developers. From fundamental techniques like ANSI escape sequences to the convenience of class-based solutions, the article covers an array of approaches.
External packages such as termcolor
, colorama
, simple-colors
, and the click
library are introduced, each offering its unique advantages in styling terminal text.
It is worth noting that while these methods empower developers with diverse ways to incorporate bold text in their Python output, it’s crucial to consider the compatibility of ANSI escape sequences, especially in environments that may not fully support them.